Linear Search: An Introduction
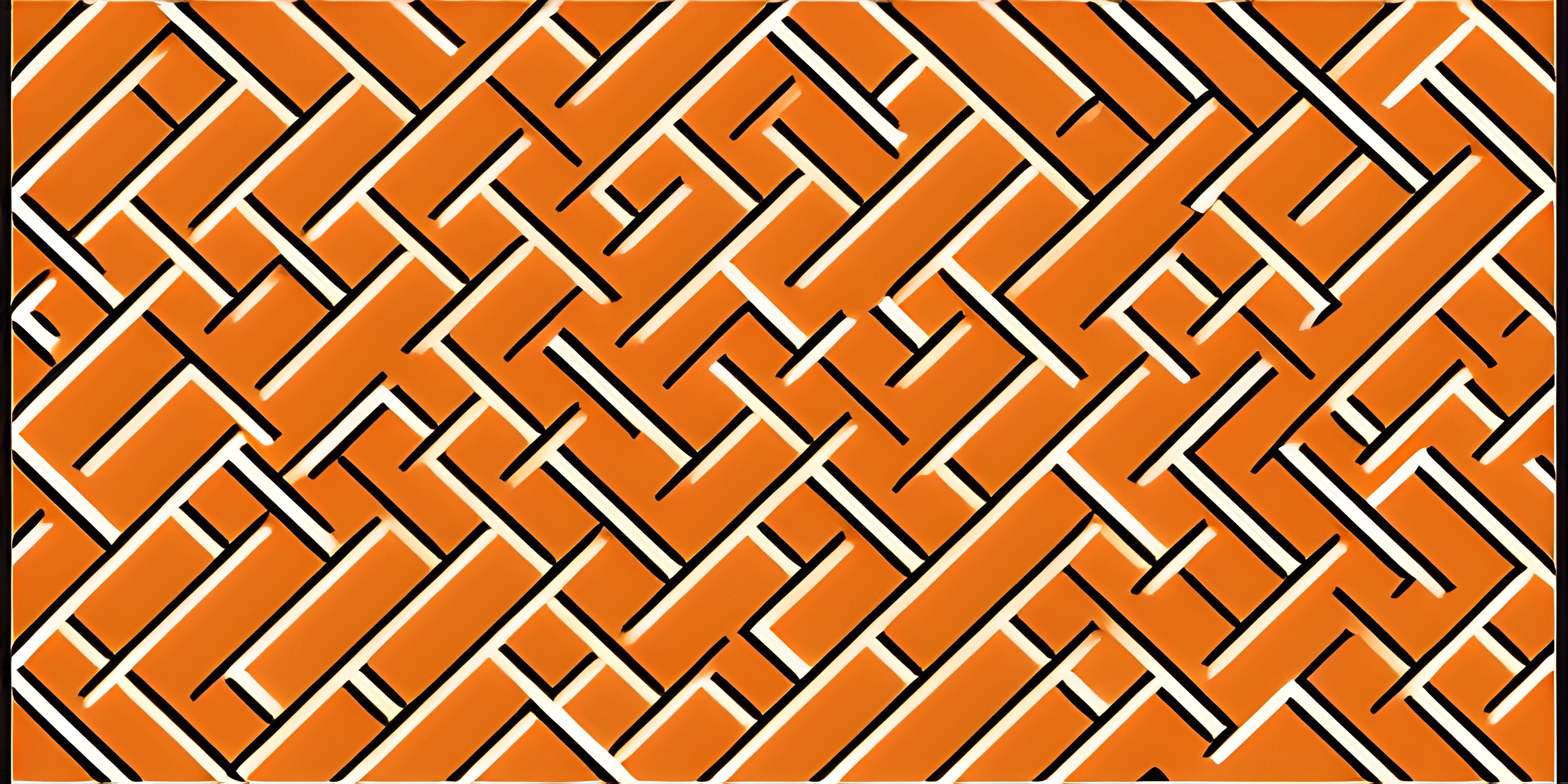
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Searching for a specific element in a collection is a common task in programming. One of the simplest ways to perform this task is by using the linear search algorithm. It's like looking for a book in a disorganized shelf, checking one by one until you find what you're looking for.
What is Linear Search?
Linear search, also known as a sequential search, is an algorithm that searches for a target element within a list or an array by iterating through each element from the beginning until the desired element is found or the end is reached.
The algorithm can be applied to any type of list or array, whether it's sorted or unsorted, and is considered an easy-to-understand and beginner-friendly search algorithm. However, linear search may not be the most efficient option, especially for large datasets, as it can take a long time to search through each element.
Use Cases
Linear search is best suited for small datasets or when the data is unsorted, and more advanced search algorithms like binary search cannot be applied. Some common use cases for linear search include:
- Searching for a specific item in a small list or array
- Finding the index of an element in an unsorted dataset
- Checking if an element exists in a collection without any specific order
- When the data is continuously changing, and maintaining a sorted dataset is not feasible
Implementing Linear Search
Let's take a look at a simple implementation of the linear search algorithm in Python:
def linear_search(arr, target): for index, element in enumerate(arr): if element == target: return index return -1 my_list = [34, 2, 19, 47, 6, 12] target_value = 19 result = linear_search(my_list, target_value) if result != -1: print(f"Element {target_value} found at index {result}") else: print(f"Element {target_value} not found in the list")
In this example, the linear_search
function takes an array (or list) and a target value as input, iterates through each element using a for
loop, and checks if the current element is equal to the target. If the target is found, the function returns its index; otherwise, it returns -1
to indicate that the target is not present in the array.
Remember that the linear search algorithm can be implemented in any programming language, and the overall logic remains the same.
Conclusion
Linear search is a straightforward and easy-to-understand algorithm for searching elements in a list or an array. Although it's not the most efficient search algorithm, it's useful in specific scenarios and serves as a foundation for understanding more advanced search algorithms. As you progress in your programming journey, exploring different techniques and their use cases helps you make better decisions when solving problems.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Smiley Face (psst, it's free!).