Algorithms Introduction
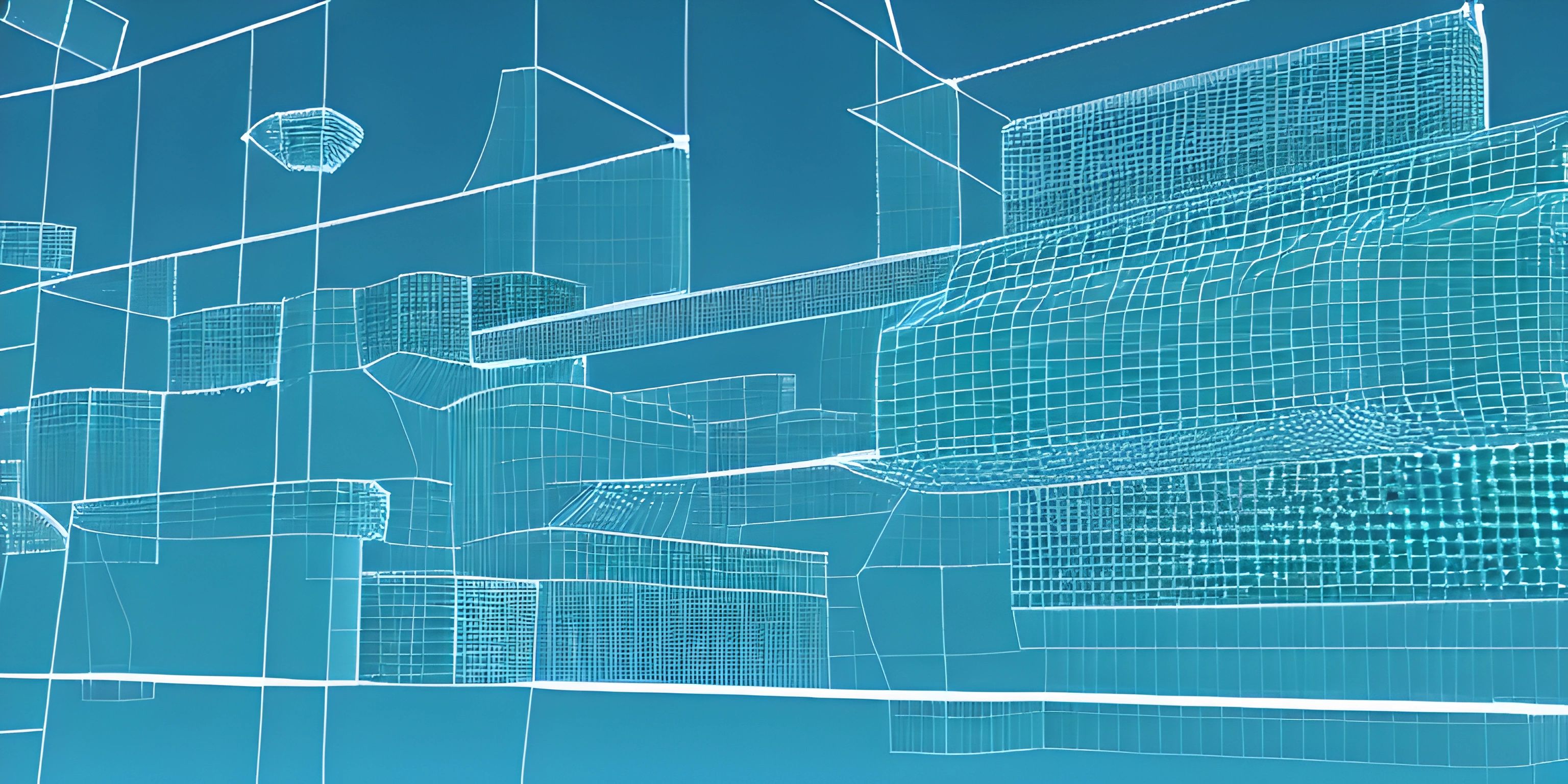
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Algorithms are the beating heart of computer programs, controlling the flow of data and enabling us to solve complex problems. Let's dive into the world of algorithms and discover what they are, how they work, and their importance in computer programming!
What is an Algorithm?
An algorithm is a sequence of steps or instructions designed to solve a specific problem or perform a specific task. A recipe for baking cookies, for example, is an algorithm that instructs you to gather ingredients, mix them together, and bake them at a specific temperature for a set amount of time. In computer programming, algorithms are vital as they dictate the way a program processes data and performs calculations.
Types of Algorithms
Algorithms come in many flavors, each tailored to tackle specific problems. Let's take a look at some common types:
-
Sorting algorithms – Arrange elements in a specific order, such as bubble sort or merge sort.
-
Searching algorithms – Find a specific element in a dataset, like linear search or binary search.
-
Graph algorithms – Solve problems related to graphs, such as Dijkstra's shortest path algorithm or depth-first search.
-
Dynamic programming algorithms – Break a problem into smaller subproblems and solve them using a combination of memory and recursion. Examples include Fibonacci sequence generation and knapsack problem.
Algorithm Complexity
An algorithm's efficiency is crucial, as it directly impacts a program's performance. There are two primary factors that contribute to an algorithm's complexity:
-
Time complexity – The amount of time an algorithm takes to run as a function of its input size. For example, if an algorithm takes twice as long to process a dataset that's twice as large, its time complexity is linear (O(n)).
-
Space complexity – The amount of memory an algorithm uses as a function of its input size. An algorithm with low space complexity uses minimal additional memory, while one with high space complexity might require significant memory resources.
Big-O notation is commonly used to express an algorithm's time and space complexity. For example, O(n) represents linear complexity, while O(n^2) denotes quadratic complexity.
Creating and Optimizing Algorithms
When developing an algorithm, it's crucial to consider both time and space complexity. A well-optimized algorithm should strike a balance between the two, providing an efficient solution without overburdening system resources.
To create and optimize algorithms, programmers often follow these steps:
- Problem definition – Clearly define the problem the algorithm needs to solve.
- Design – Determine the algorithm's structure and steps.
- Implementation – Translate the algorithm into code using a programming language.
- Testing – Verify the algorithm's correctness and efficiency by running it on sample inputs.
- Optimization – Refine the algorithm to improve its time and/or space complexity.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is an algorithm?
An algorithm is a sequence of steps or instructions designed to solve a specific problem or perform a specific task. In computer programming, algorithms are crucial as they dictate the way a program processes data and performs calculations.
What are some common types of algorithms?
Some common types of algorithms include sorting algorithms (e.g., bubble sort, merge sort), searching algorithms (e.g., linear search, binary search), graph algorithms (e.g., Dijkstra's shortest path algorithm, depth-first search), and dynamic programming algorithms (e.g., Fibonacci sequence generation, knapsack problem).
How do you measure an algorithm's complexity?
An algorithm's complexity is typically measured in terms of time complexity (how long the algorithm takes to run as a function of its input size) and space complexity (how much memory the algorithm uses as a function of its input size). Big-O notation is commonly used to express these complexities.