Introduction to Algorithms
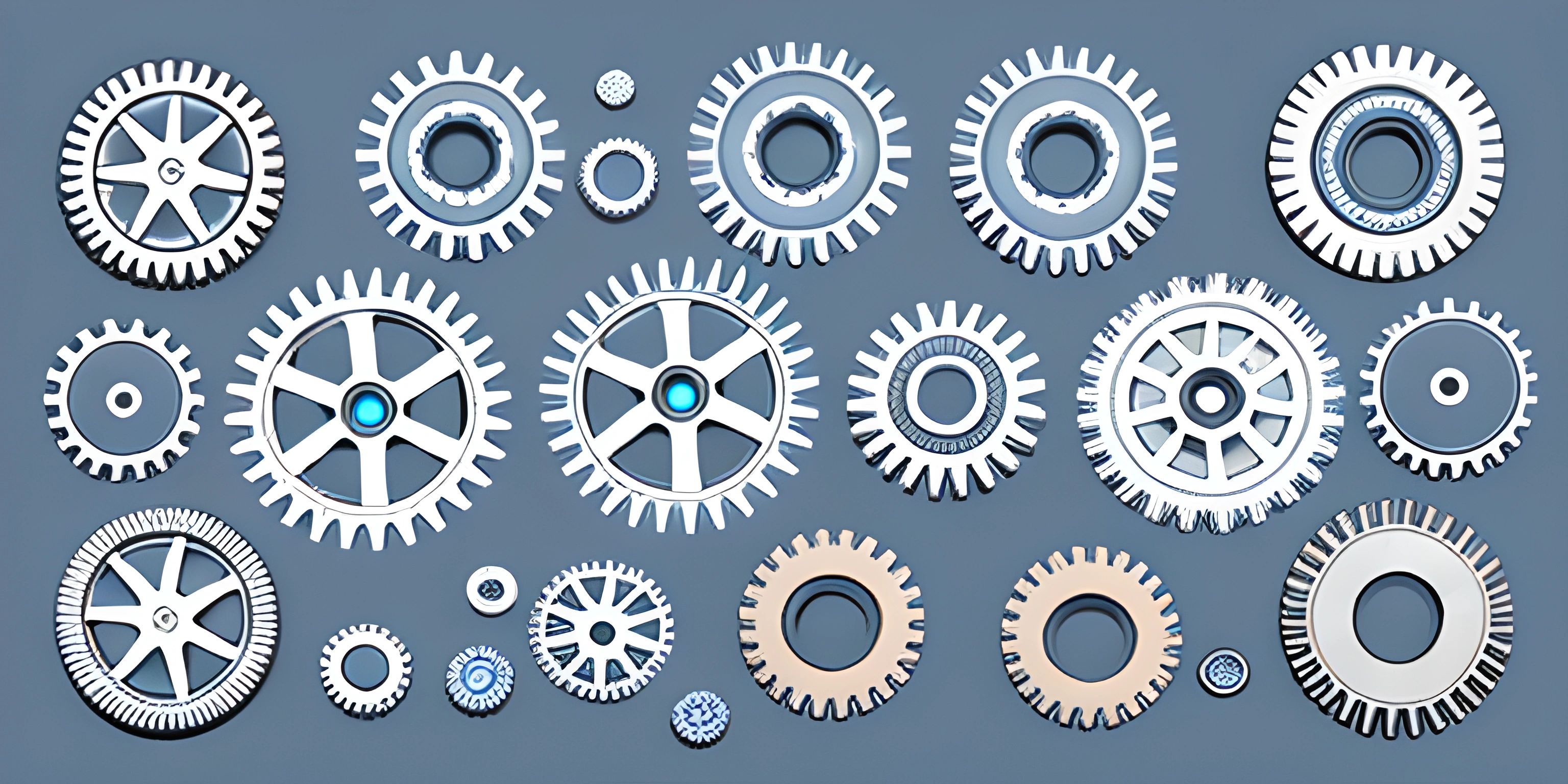
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming is like a magical toolbox, and algorithms are the enchanted tools inside. An algorithm is a sequence of steps or a set of rules to accomplish a specific task or solve a problem. They are the building blocks of any software or application, and they're responsible for making your computer perform its many tricks. Let's dive into the world of algorithms and uncover their mysteries.
The Essence of Algorithms
Imagine you're a wizard-in-training and you've been given the task of brewing a potion. You'll need a recipe, which is essentially an algorithm. The recipe tells you the ingredients, their quantities, and the sequence of steps to mix and cook them to perfection.
Similarly, algorithms in programming are step-by-step instructions to solve problems or perform tasks. These instructions can be followed by a computer, which translates them into actions. The algorithm's efficiency and effectiveness can make or break the performance of your software.
Types of Algorithms
There are countless algorithms, each with its own strengths and weaknesses. Some common types of algorithms include:
- Search algorithms: Help you find a specific item in a data set, like a lost wand in a pile of wands. Examples include linear search and binary search.
- Sorting algorithms: Organize data in a specific order, like arranging potion ingredients alphabetically. Examples include bubble sort and quick sort.
- Graph algorithms: Solve problems related to graphs (networks of nodes and edges), like finding the shortest path between two magical realms. Examples include Dijkstra's algorithm and Kruskal's algorithm.
Creating and Analyzing Algorithms
Creating an algorithm is like crafting a spell. You need to carefully choose the right incantations (steps) and ingredients (data) to make it work. Here's a simple example of an algorithm to calculate the sum of two numbers:
def add_numbers(a, b): sum = a + b return sum
This algorithm is a function that takes two numbers as input (a and b), calculates their sum, and returns the result.
Once you've created an algorithm, you'll want to analyze its performance. This is often done by looking at its time complexity and space complexity. Time complexity measures how long an algorithm takes to run, while space complexity measures the amount of memory it uses.
For example, if an algorithm has a time complexity of O(n), it means that its running time increases linearly with the size of the input. If the input size doubles, the running time will also double. Understanding the complexities of an algorithm helps you identify potential bottlenecks and optimize your code for better performance.
The Power of Algorithms
Algorithms are the spells that bring your software to life. They empower your applications to search, sort, and process data efficiently. By mastering the art of algorithms, you'll be well on your way to becoming a programming wizard.
Remember, practice makes perfect. The more algorithms you create and analyze, the better you'll become at crafting efficient and effective solutions to solve a wide variety of problems. So, grab your wand (keyboard) and start weaving your own algorithmic magic!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is an algorithm?
An algorithm is a step-by-step procedure for solving a problem or performing a specific task. In programming, algorithms are essential for developing efficient and effective solutions to various problems. They consist of a series of instructions that are executed in a particular order, transforming input data into the desired output.
Why are algorithms important in programming?
Algorithms are the foundation of programming. They provide a clear and structured approach to problem-solving, ensuring that the software produces correct and efficient results. Understanding and implementing algorithms can help in:
- Developing more efficient code
- Reducing the time and resources required for a program to run
- Enhancing the problem-solving abilities of a programmer
- Making it easier to communicate and collaborate with other developers
How do I create an algorithm?
Creating an algorithm involves the following steps:
- Define the problem: Clearly understand the problem you're trying to solve.
- Determine the inputs and outputs: Identify the required input data and the desired output for the problem.
- Design the algorithm: Break down the problem into smaller tasks, and develop a step-by-step procedure to solve it.
- Test the algorithm: Verify the correctness and efficiency of the algorithm using different input data and test cases.
- Refine the algorithm: If necessary, improve the algorithm by optimizing its steps or introducing alternative solutions.
What are some common techniques for analyzing algorithms?
Some common techniques for analyzing algorithms include:
- Time complexity: Evaluating the performance of an algorithm based on the number of steps or operations it takes to execute, relative to the size of the input data.
- Space complexity: Assessing the amount of memory required by an algorithm as a function of the input size.
- Asymptotic notation: Using notations such as Big O, Big Omega, and Big Theta to describe the upper and lower bounds of an algorithm's performance.
- Empirical analysis: Running the algorithm on various test cases and measuring its performance using real-world data.
Can you provide an example of a simple algorithm?
Sure! Here's an example of a simple algorithm to find the largest number in a list of integers:
- Initialize a variable
max
to the first element of the list. - Iterate through the list, comparing each element to the current
max
value. - If an element is greater than
max
, updatemax
to the current element. - Continue iterating until the end of the list is reached.
- The
max
variable now holds the largest number in the list.