Introduction to Genetic Algorithms
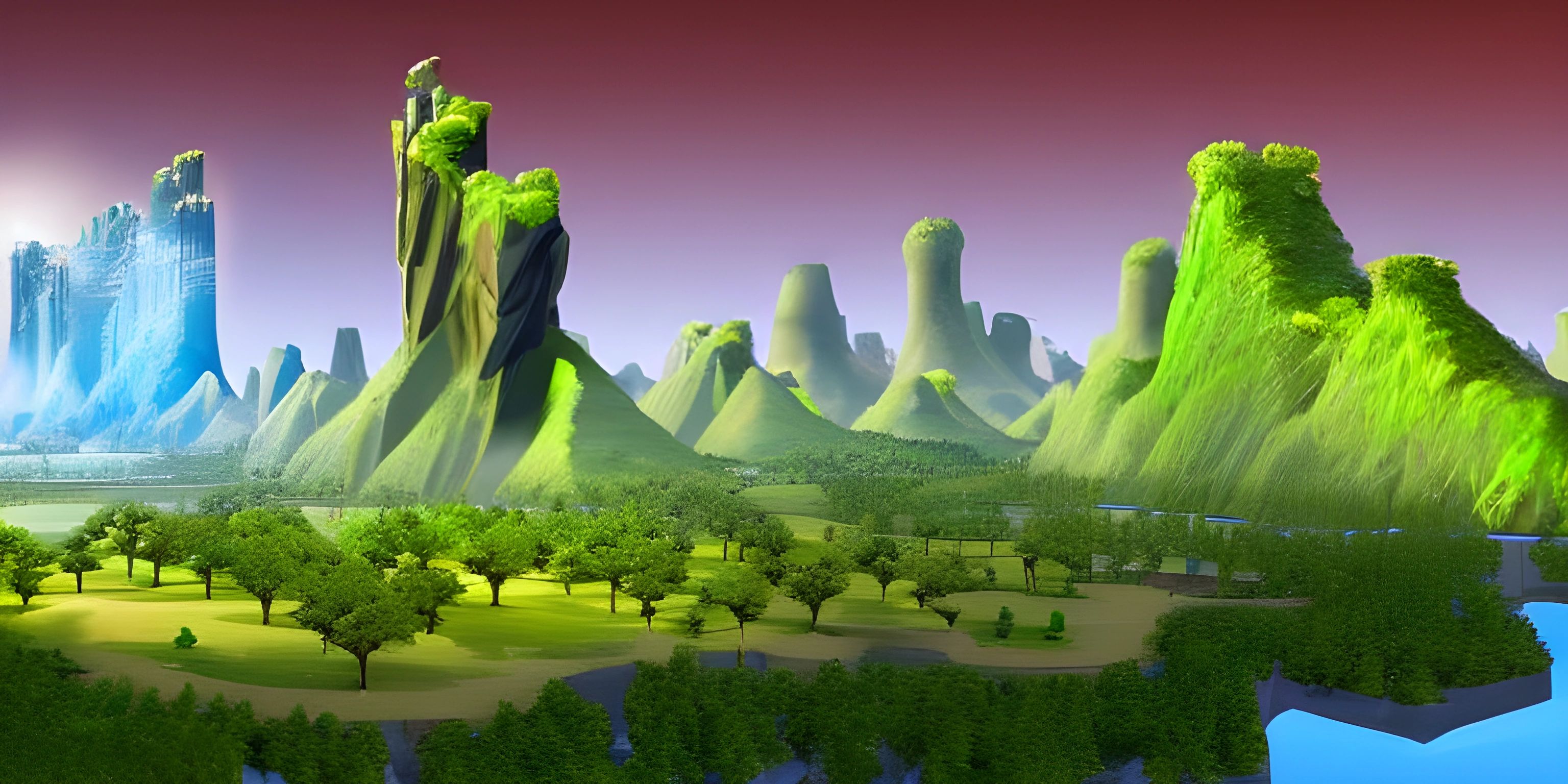
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of AI and optimization, genetic algorithms (GAs) are like nature's cheat codes. Inspired by Charles Darwin's theory of natural evolution, GAs use the principles of selection, crossover, and mutation to solve complex problems. But before you start imagining robots in white lab coats, let’s dive into the nuts and bolts of genetic algorithms.
What Are Genetic Algorithms?
Genetic algorithms are search heuristics that mimic the process of natural selection to find optimal solutions. Think of them as digital gardeners, planting seeds (potential solutions), weeding out the weak, and cross-breeding the strong until they cultivate the best possible result.
Key Components
To understand genetic algorithms, you need to get cozy with a few essential concepts:
- Population: A set of potential solutions.
- Chromosomes: Encoded versions of the potential solutions.
- Genes: Parts of a chromosome that represent specific parameters.
- Fitness Function: A way to evaluate how good a solution is.
- Selection: Choosing the best solutions for reproduction.
- Crossover: Combining two parent solutions to create offspring.
- Mutation: Randomly altering parts of a solution to introduce diversity.
The Genetic Algorithm Process
Here’s the general flow of a genetic algorithm:
-
Initialization:
- Begin with a randomly generated population of N chromosomes.
- Each chromosome represents a potential solution to the problem.
-
Evaluation:
- Evaluate the fitness of each chromosome using the fitness function.
-
Selection:
- Select a subset of the current population based on fitness.
- Typically, better solutions have a higher chance of being selected.
-
Crossover:
- Combine pairs of parent chromosomes to produce offspring.
- This is akin to biological reproduction, mixing genetic material to create variations.
-
Mutation:
- Randomly tweak genes in offspring chromosomes to introduce new traits.
- This helps maintain genetic diversity and avoid premature convergence.
-
Replacement:
- Replace the old population with the new generation of chromosomes.
-
Termination:
- Repeat steps 2-6 until a stopping condition is met (e.g., a satisfactory fitness level or a maximum number of generations).
Example: Solving the Traveling Salesman Problem (TSP)
Let’s break down an example where we use a genetic algorithm to solve the Traveling Salesman Problem (TSP). The TSP asks: “Given a list of cities and the distances between them, what is the shortest possible route that visits each city once and returns to the origin city?”
Step-by-Step TSP Solution
-
Initialization: Generate a population of random possible routes between cities.
import random def create_route(city_list): return random.sample(city_list, len(city_list))
-
Fitness Function: Define a function to calculate the total distance of a route.
def route_distance(route): distance = 0 for i in range(len(route) - 1): distance += distance_between(route[i], route[i + 1]) distance += distance_between(route[-1], route[0]) # Return to start return distance def fitness(route): return 1 / route_distance(route)
-
Selection: Select the fittest routes for mating.
def select_parents(population, fitness_scores): parents = random.choices( population, weights=fitness_scores, k=len(population)//2 ) return parents
-
Crossover: Create new offspring by combining parts of parent routes.
def crossover(parent1, parent2): child = [] start_pos = random.randint(0, len(parent1) - 1) end_pos = random.randint(0, len(parent1) - 1) for i in range(min(start_pos, end_pos), max(start_pos, end_pos)): child.append(parent1[i]) child += [gene for gene in parent2 if gene not in child] return child
-
Mutation: Randomly swap two cities in the route.
def mutate(route, mutation_rate): for swapped in range(len(route)): if random.random() < mutation_rate: swap_with = int(random.random() * len(route)) city1 = route[swapped] city2 = route[swap_with] route[swapped] = city2 route[swap_with] = city1 return route
-
Create New Population: Generate the next generation.
def next_generation(current_gen, mutation_rate): fitness_scores = [fitness(route) for route in current_gen] parents = select_parents(current_gen, fitness_scores) children = [] for i in range(len(parents)//2): child = crossover(parents[i], parents[len(parents) - i - 1]) children.append(mutate(child, mutation_rate)) return children
-
Run the Genetic Algorithm: Iterate until convergence.
def genetic_algorithm(city_list, pop_size, mutation_rate, generations): population = [create_route(city_list) for _ in range(pop_size)] for i in range(generations): population = next_generation(population, mutation_rate) best_route = min(population, key=route_distance) return best_route
Voilà! You've just created a rudimentary genetic algorithm to solve the TSP.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What makes genetic algorithms useful?
Genetic algorithms are particularly useful for optimization problems where the search space is large and complex. They can provide good solutions quickly, even if those solutions aren't always perfect. Their ability to explore a wide range of possible solutions makes them versatile and powerful.
Can genetic algorithms guarantee the best solution?
No, genetic algorithms do not guarantee the best solution. They are heuristic methods, meaning they provide good solutions within a reasonable amount of time, but there's no certainty that the solution will be the absolute best. They work well when exact solutions are not feasible.
How do I choose a fitness function?
Choosing a fitness function depends on the specific problem you are solving. The fitness function should accurately measure how good a potential solution is. For example, in the TSP, the fitness function could be the inverse of the total distance of the route.
What are some common applications of genetic algorithms?
Genetic algorithms are used in a variety of fields such as robotics, game development, bioinformatics, and financial modeling. They are valuable for solving problems like scheduling, evolving neural networks, designing efficient circuits, and optimizing investment portfolios.
Are there any limitations to using genetic algorithms?
Yes, genetic algorithms can be computationally expensive and may converge to local optima rather than global optima. They also require careful tuning of parameters like population size, crossover rate, and mutation rate to perform effectively.