Buffer Overflows
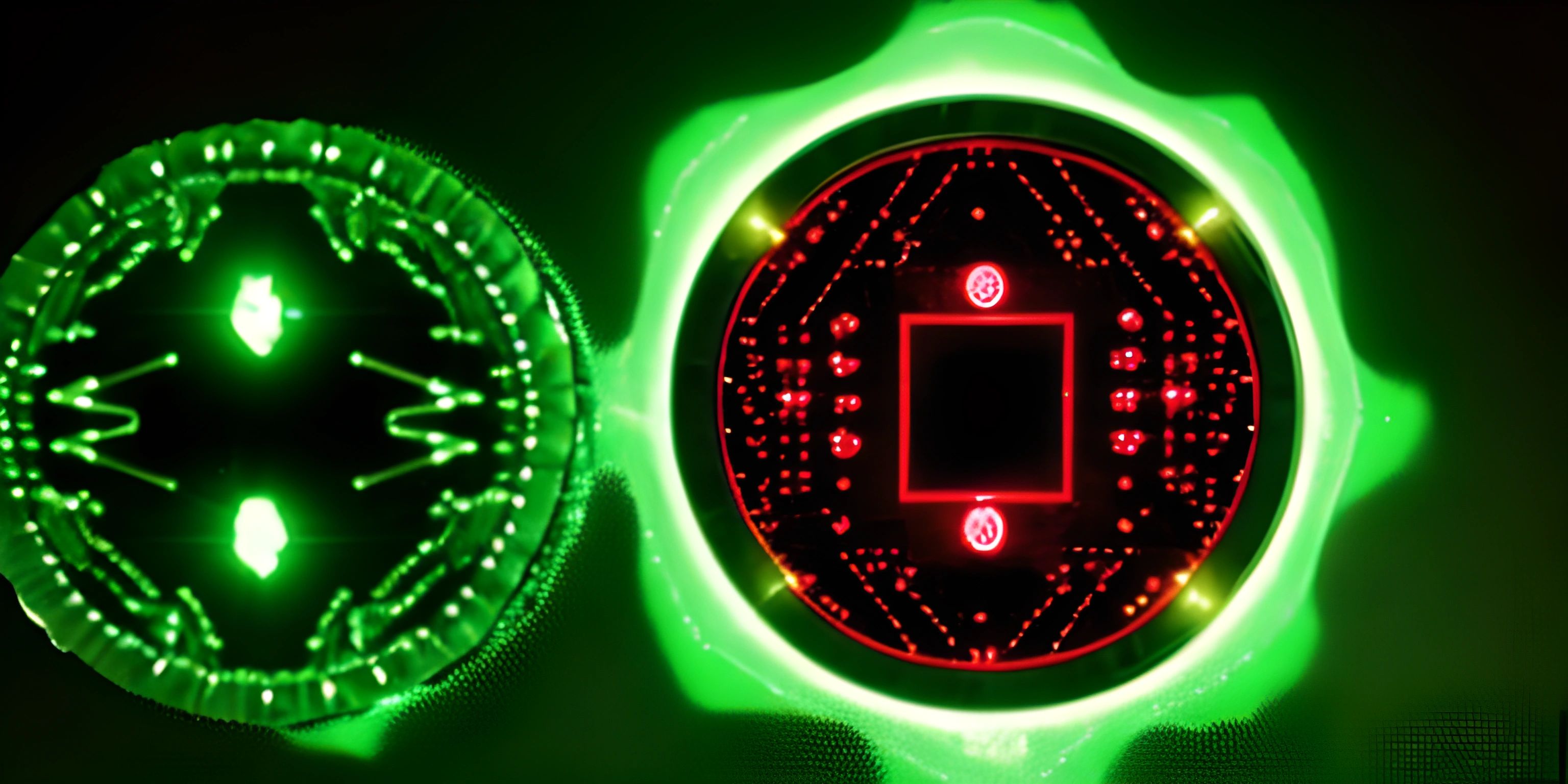
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Buffer overflows are like trying to pour too much water into a glass: eventually, it's going to spill over and make a mess. When it happens in programming, it can cause unexpected behavior, crashes, and even security vulnerabilities. So, let's dive into what causes buffer overflows and how to prevent them.
What is a Buffer Overflow?
In computer programming, a buffer is a region of memory used to temporarily store data. Buffers are often created to hold a specific amount or type of data, and have a predefined size. A buffer overflow occurs when more data is written to or read from a buffer than it can hold, causing the excess data to "spill over" into adjacent memory locations.
This can lead to a variety of problems, such as:
- Corrupted data
- Application crashes
- Unauthorized access to sensitive information
- Execution of malicious code
Causes of Buffer Overflows
Buffer overflows are typically caused by two main factors: insufficient bounds checking and incorrect buffer size calculations.
Insufficient Bounds Checking
This occurs when a program does not properly verify that the data being written to or read from a buffer is within the buffer's size limitations. For example, consider the following C++ code:
#include <iostream> #include <cstring> int main() { char buffer[10]; strcpy(buffer, "This string is too long for the buffer!"); std::cout << buffer << std::endl; return 0; }
In this example, the strcpy()
function copies a string that is longer than the buffer's size, causing a buffer overflow. The program does not check whether the string will fit into the buffer, leading to the overflow.
Incorrect Buffer Size Calculations
Another cause of buffer overflows is incorrect buffer size calculations. This can occur when a programmer misunderstands the size of a data type or forgets to account for null terminators in strings. For example, the following Python code creates a buffer that is too small to hold a string:
data = "Hello, world!" buffer_size = len(data) # This should be len(data) + 1 to account for the null terminator buffer = bytearray(buffer_size) for i in range(len(data)): buffer[i] = data[i]
In this example, the buffer size is calculated based on the length of the string, but it doesn't account for the null terminator. This leads to a buffer overflow when the string is copied into the buffer.
Prevention Techniques
There are several techniques that can help prevent buffer overflows:
-
Bounds Checking: Always verify that the data being written to or read from a buffer is within the buffer's size limitations. This can be done using built-in functions or manually checking the size of the data before writing or reading it.
-
Use Safe Functions: Many programming languages offer "safe" alternatives to functions prone to buffer overflows, such as
strncpy()
in C++ orcopy()
in Python. These functions typically include bounds checking or other safety measures to prevent overflows. -
Correct Buffer Size Calculations: Be mindful of data type sizes and null terminators in strings when calculating buffer sizes. Ensure that buffers are large enough to hold the intended data, including any necessary termination characters.
-
Address Space Layout Randomization (ASLR): ASLR is a security technique used by some operating systems to randomize the location of memory segments, making it more difficult for an attacker to exploit buffer overflows.
By understanding the causes and consequences of buffer overflows and applying these prevention techniques, you can help make your code more secure and robust against potential vulnerabilities.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a buffer overflow?
A buffer overflow is a programming error that occurs when a program tries to store more data in a buffer than it can hold. This causes the extra data to "overflow" into adjacent memory locations, potentially leading to unpredictable behavior or even crashing the program. Buffer overflows are particularly dangerous because they can be exploited by attackers to execute malicious code or gain unauthorized access to a system.
How do buffer overflows happen?
Buffer overflows typically happen when a programmer fails to properly manage memory allocation or doesn't perform adequate bounds checking when writing data to a buffer. When the program tries to write more data than the buffer can hold, the extra data "spills" into adjacent memory locations, leading to corruption of other data or causing the program to behave unexpectedly.
How can buffer overflows be prevented?
Preventing buffer overflows requires careful attention to memory management and data handling in a program. Here are some tips for avoiding buffer overflows:
- Always perform bounds checking when writing data to a buffer.
- Use built-in functions or libraries that handle memory allocation and buffer management safely.
- Limit input sizes to ensure they do not exceed buffer capacity.
- Regularly test your code for vulnerabilities and use tools like static code analyzers to help identify potential issues.
Can you give an example of a simple buffer overflow?
Sure! Here's an example in C that demonstrates a buffer overflow:
#include <stdio.h> #include <string.h> int main() { char buffer[10]; printf("Enter your name: "); gets(buffer); // Unsafe function, should use fgets() instead printf("Hello, %s!\n", buffer); return 0; }
In this example, the gets()
function is used to read input from the user. However, gets()
does not perform bounds checking and can write more data to the buffer than it can hold, leading to a buffer overflow. To fix this, you should use fgets()
instead, which allows you to specify the maximum number of characters to read.
How can attackers exploit buffer overflows?
Attackers can exploit buffer overflows by providing carefully crafted input that overwrites the buffer and adjacent memory locations with malicious data. This can lead to the execution of attacker-controlled code or the modification of critical program data, allowing the attacker to gain unauthorized access to the system or cause other types of harm. To protect against these attacks, it's important to follow secure programming practices and regularly test your code for vulnerabilities.