Understanding and Managing Node.js Package Dependencies with npm
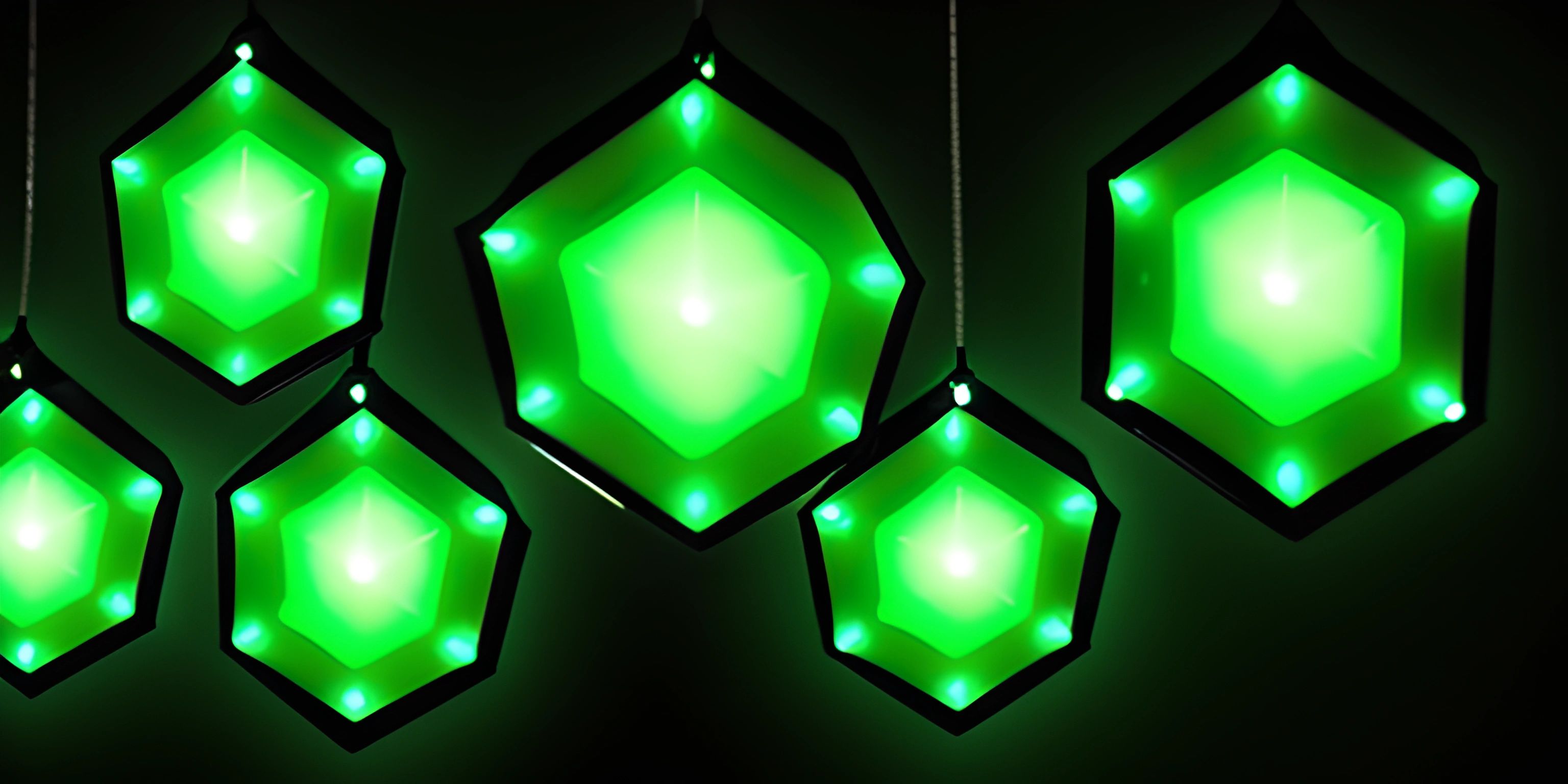
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Node.js is a popular JavaScript runtime environment that allows you to run JavaScript code on the server-side. One of the key features of Node.js is its built-in package manager called npm. npm stands for Node Package Manager and is responsible for managing package dependencies in Node.js projects.
This article will cover:
- What are packages and dependencies?
- How to use npm to manage dependencies in a Node.js project.
- Common npm commands.
What are packages and dependencies?
A package is a reusable piece of code that performs a specific task or provides a specific functionality. Packages can be shared and reused across multiple projects. In Node.js, packages are also known as modules.
A dependency is a package that your project relies on to function correctly. For example, if your project uses a package to handle HTTP requests, that package becomes a dependency of your project.
Using npm to manage dependencies
To manage dependencies in a Node.js project, you need to create a file called package.json
. This file contains information about your project, such as its name, version, and a list of its dependencies.
To create a package.json
file, navigate to your project folder in the terminal and run:
npm init
You'll be prompted to provide some information about your project. You can either fill in the details or press Enter to accept the default values. Once you're done, a package.json
file will be created in your project folder.
To add a package as a dependency, you can use the npm install
command followed by the package name. For example, to install the popular express
web framework, you'd run:
npm install express
This command will download the express
package and its dependencies and save them in a folder called node_modules
. It will also update the package.json
file with the newly added dependency.
Common npm commands
Here are some common npm commands you'll use while managing packages in a Node.js project:
npm init
: Initializes a new Node.js project and creates apackage.json
file.npm install <package_name>
: Installs a package and adds it as a dependency in thepackage.json
file.npm install
: Installs all the dependencies listed in thepackage.json
file. This is useful when setting up a project on a new machine or after cloning a repository.npm uninstall <package_name>
: Uninstalls a package and removes it from thepackage.json
file.npm update
: Updates all the packages listed in thepackage.json
file to their latest versions.npm list
: Lists all the installed packages and their dependencies.
By understanding and using npm effectively, you can manage your Node.js project's dependencies with ease and ensure your project runs smoothly.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is npm and why is it important for Node.js development?
npm, short for Node Package Manager, is an essential tool for Node.js development. It is a package manager that allows developers to easily install, update, and manage external libraries and modules in their Node.js projects. The use of npm streamlines the development process and promotes code reusability, as developers can share and use packages created by others.
How do I install a package using npm?
To install a package using npm, you need to open your command line or terminal and navigate to your project's root directory, where your package.json
file is located. Then, run the following command, replacing package-name
with the name of the package you want to install:
npm install package-name
This command will install the package and its dependencies in the node_modules
folder and update your package.json
file accordingly.
What is the purpose of the package.json file?
The package.json
file is a crucial component of any Node.js project that uses npm. It serves as a manifest file that contains metadata about your project, such as its name, version, description, and author. Most importantly, it lists the project's dependencies – the external packages your project relies on – along with their specific versions. This enables other developers to easily install and manage the necessary dependencies when working on your project, ensuring consistent behavior across different environments.
How can I update an existing npm package in my project?
To update a specific npm package in your project, you can run the following command in your terminal or command line, replacing package-name
with the name of the package you want to update:
npm update package-name
This command will check for the latest version of the specified package and update it in your node_modules
folder and package.json
file if a newer version is available. To update all packages to their latest versions, simply run:
npm update
How do I uninstall an npm package from my project?
To uninstall an npm package from your project, open your terminal or command line, navigate to your project's root directory, and run the following command, replacing package-name
with the name of the package you wish to remove:
npm uninstall package-name
This will remove the package and its dependencies from the node_modules
folder and update your package.json
file accordingly.