Intel x86 Memory Management
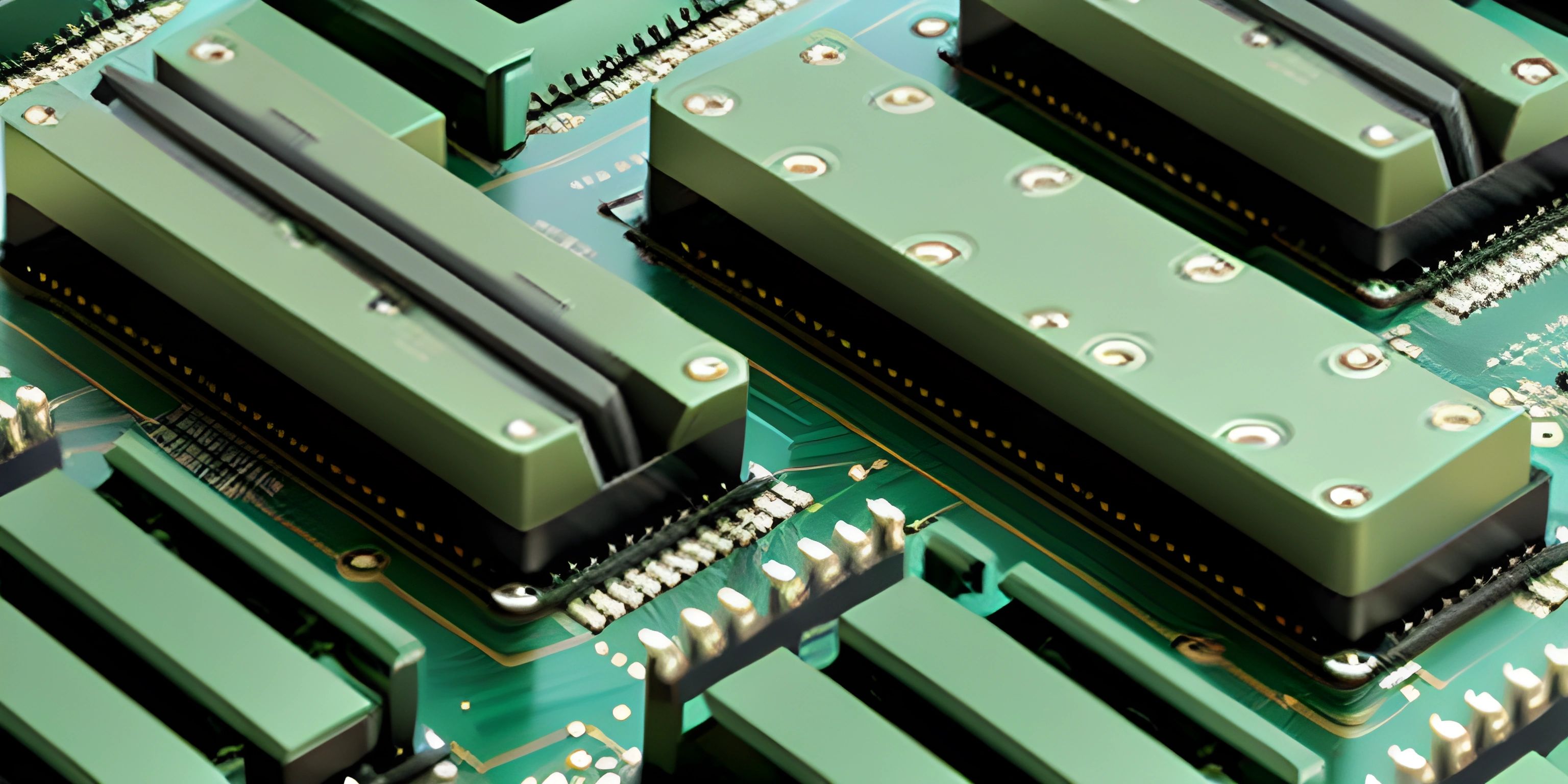
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Memory management can feel like juggling flaming torches while riding a unicycle. But don’t worry; by the end of this article, you’ll be gracefully pirouetting through the world of Intel x86 memory management techniques and practices. Let’s get started!
The Basics of Memory Management
At its core, memory management is the process of controlling and coordinating computer memory, assigning blocks to various running programs to optimize overall system performance. Think of it as the librarian of your computer, ensuring every book (data) is in the right place.
Segmentation
In the Intel x86 architecture, memory is managed using a combination of segmentation and paging.
What is Segmentation?
Segmentation is like breaking a library into different sections: fiction, non-fiction, reference, etc. Each section contains different books, and each book has a unique identifier within that section. Similarly, in segmentation, memory is divided into different segments, each containing a specific type of data or code.
How Does It Work?
Segmentation in Intel x86 uses segment registers such as CS
(Code Segment), DS
(Data Segment), SS
(Stack Segment), and others. Each segment register holds the base address of the segment it points to. Combine this with an offset, and you get the actual physical address.
; Example of using segment registers in assembly mov ax, 0x1234 ; Load segment base address mov ds, ax ; Assign to Data Segment register mov bx, 0x0004 ; Offset within the segment mov al, [ds:bx] ; Access memory at DS:BX
Paging
What is Paging?
Paging is another layer of memory management, like having an index that maps book titles to their specific locations on the shelves. It breaks the memory into fixed-size blocks called pages and maps them to physical memory frames.
How Does It Work?
When the CPU needs to access a memory location, it uses a page table to translate the virtual address to a physical address. This allows for efficient use of memory and helps in implementing virtual memory.
; Simplified example of paging mov cr3, page_directory_base ; Load page directory base address mov eax, [virtual_address] ; Attempt to access a virtual address
Page Tables
A page table is a data structure used by the virtual memory system to store the mapping between virtual addresses and physical addresses. Each entry in the page table corresponds to one page of virtual memory.
Memory Protection
What is Memory Protection?
Memory protection is like having a security guard in your library who ensures that only authorized individuals can access certain sections. It prevents programs from interfering with each other’s memory.
How Does It Work?
Memory protection in Intel x86 can be achieved through segmentation and paging. Each segment and page can have different access rights, like read, write, or execute. This is managed by setting appropriate bits in the segment descriptors and page table entries.
; Example of setting access rights in segment descriptor mov ax, segment_selector mov es, ax ; Load segment selector into ES register ; Access rights are defined in the segment descriptor
Advanced Techniques
Virtual Memory
Virtual memory is like having an infinite library where books can be stored in different warehouses. The system provides an illusion of a vast memory space by swapping pages between physical memory and disk storage.
How Does It Work?
When a program exceeds the available physical memory, the operating system moves some pages to a disk file called the swap file. When those pages are needed again, they are swapped back into physical memory.
; Conceptual example of virtual memory management mov eax, [virtual_address] ; Attempt to access virtual memory ; If not present in physical memory, a page fault occurs ; OS handles the page fault by swapping the required page into physical memory
Demand Paging
Demand paging is like getting books from the warehouse only when they are requested. It loads pages into memory only when they are needed, rather than loading the entire program into memory at once.
How Does It Work?
When a program accesses a page that is not currently in memory, a page fault occurs. The operating system then loads the required page from disk into physical memory.
; Example of handling a page fault mov eax, [virtual_address] ; Access virtual address ; Page fault occurs if page not in memory ; OS handles the page fault by loading the page into memory
Conclusion
Memory management in Intel x86 architecture might seem complex, but it’s all about organizing and protecting data efficiently. Whether it’s through segmentation, paging, or advanced techniques like virtual memory and demand paging, each method plays a crucial role in ensuring your programs run smoothly.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the difference between segmentation and paging?
Segmentation divides memory into variable-sized segments with specific types, while paging divides memory into fixed-size pages and maps them to physical frames. Segmentation is like sections in a library, and paging is like an index for the book locations.
How does virtual memory work?
Virtual memory provides the illusion of a large memory space by using disk storage to extend physical memory. When physical memory is full, pages are swapped between RAM and disk storage, allowing programs to exceed the physical memory limits.
What is a page fault?
A page fault occurs when a program tries to access a page that is not currently in physical memory. The operating system handles the fault by loading the required page from disk into memory and then resuming the program.
Why is memory protection important?
Memory protection prevents programs from interfering with each other’s memory, ensuring system stability and security. It restricts access rights to different memory segments and pages, allowing only authorized operations.
What are page tables?
Page tables are data structures used by the virtual memory system to map virtual addresses to physical addresses. Each entry in a page table corresponds to a page of virtual memory, helping translate virtual addresses during memory access.