Understanding and Securing Against SQL Injection Attacks
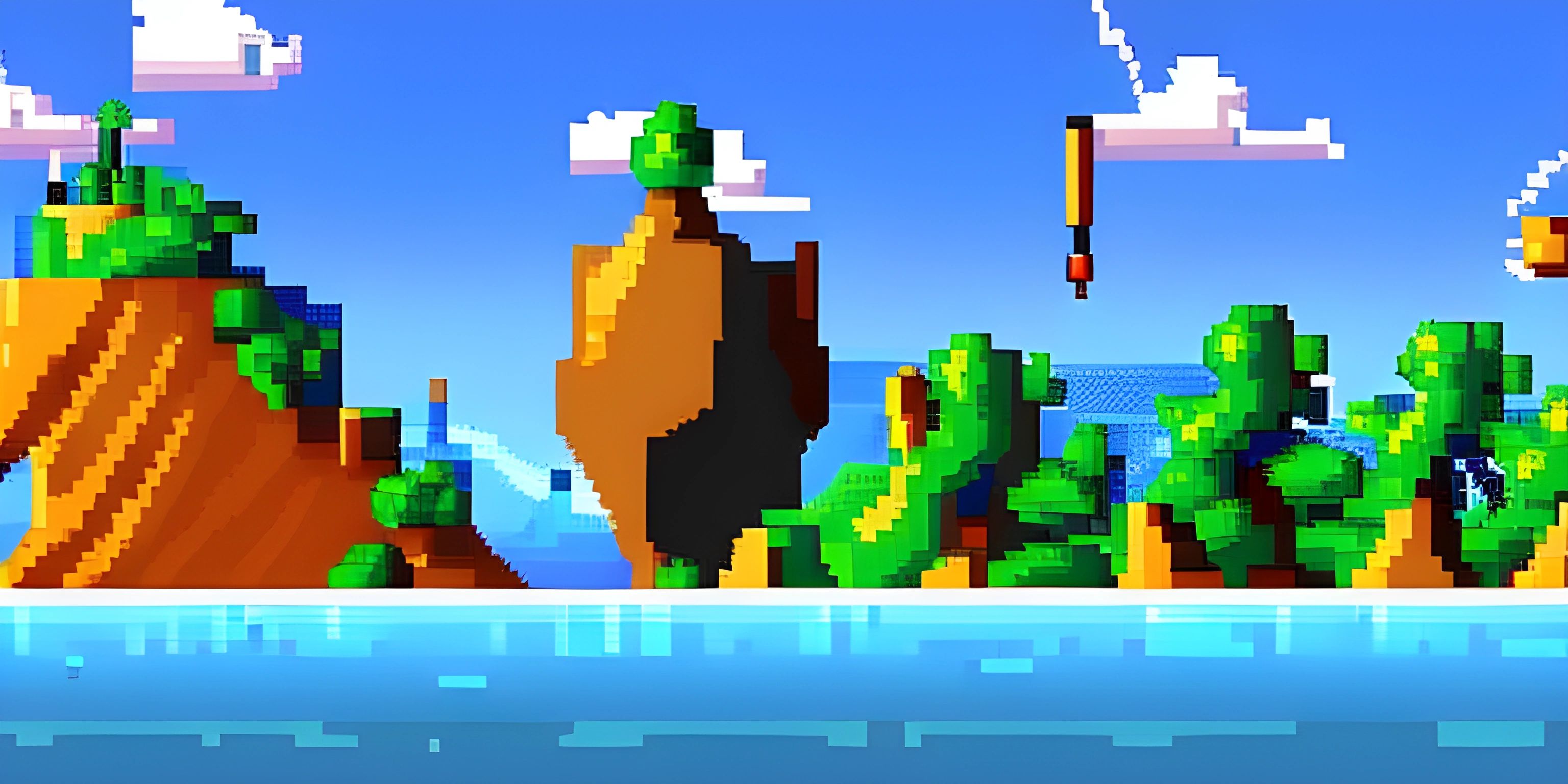
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Buckle up, brave code slinger, as we plunge into the dark world of SQL Injection Attacks! In this adventure, we'll get to understand this nefarious threat that lurks in the shadows of your database interactions, and arm ourselves with the knowledge to vanquish it!
The Villain Unmasked
SQL Injection is a type of attack that takes advantage of insecure code to manipulate your database queries. Think of it as a wolf in sheep's clothing; it sneaks in disguised as a harmless input, only to wreak havoc when you least expect it.
Imagine your application is a castle. Your database is the treasure room, filled with valuable data. The gate to this castle is guarded by your SQL queries. A SQL Injection attack is like a sneaky infiltrator who whispers the right words (or in our case, the right SQL statements) to the gatekeeper, tricking them into opening the gates and allowing access to your precious data.
A Taste of Treachery
Here's an example of how this villain operates using SQL. Let's say we have a login function in our application that accepts a username and password input:
SELECT * FROM Users WHERE Username = "input_username" AND Password = "input_password"
An infiltrator can provide a cleverly crafted username like "admin" --
, so the SQL statement becomes:
SELECT * FROM Users WHERE Username = "admin" -- AND Password = "input_password"
The --
is a comment in SQL, so everything after it is ignored. This means the infiltrator is now logged in as the admin
user without needing the password!
Fortifying The Castle
Fear not, for there are ways to fortify your castle against these attacks!
One popular way is to use parameterized queries or prepared statements, depending on your language of choice. These are like trusted gatekeepers who are immune to the infiltrator's tricks. They treat input as pure data, not part of the SQL command.
Another way is to sanitize your inputs, or in our case, strip out or escape characters that have special meaning in SQL. This is like teaching your gatekeeper to recognize and neutralize the infiltrator's cunning phrases.
Here's an example of a parameterized query using Python's psycopg2 library:
import psycopg2 # Setup connection conn = psycopg2.connect("dbname=test user=postgres") cur = conn.cursor() # Use a parameterized query cur.execute("SELECT * FROM Users WHERE Username = %s AND Password = %s", (input_username, input_password))
And remember, always keep your gatekeepers (software and packages) updated! They often come with enhancements that make them even more resistant to the infiltrator's tricks.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is SQL Injection?
SQL Injection is a type of attack where malicious users can manipulate your SQL queries through insecure code. By injecting malicious SQL statements into an input field, they can gain unauthorized access to data, modify your data, or even execute administrative operations on the database.
How can we prevent SQL Injection attacks?
SQL Injection attacks can be prevented by using techniques like parameterized queries, prepared statements, and input sanitization. These techniques ensure that user inputs are treated as pure data and not part of the SQL command. Regularly updating your software and packages also helps in tightening security.
What is the impact of a successful SQL Injection attack?
A successful SQL Injection attack can lead to unauthorized access to sensitive data, data loss, or even a complete system compromise. It can cause serious breaches of confidentiality, integrity, and availability of data and services, potentially leading to financial losses, damage to reputation, and legal implications.