C Best Practices
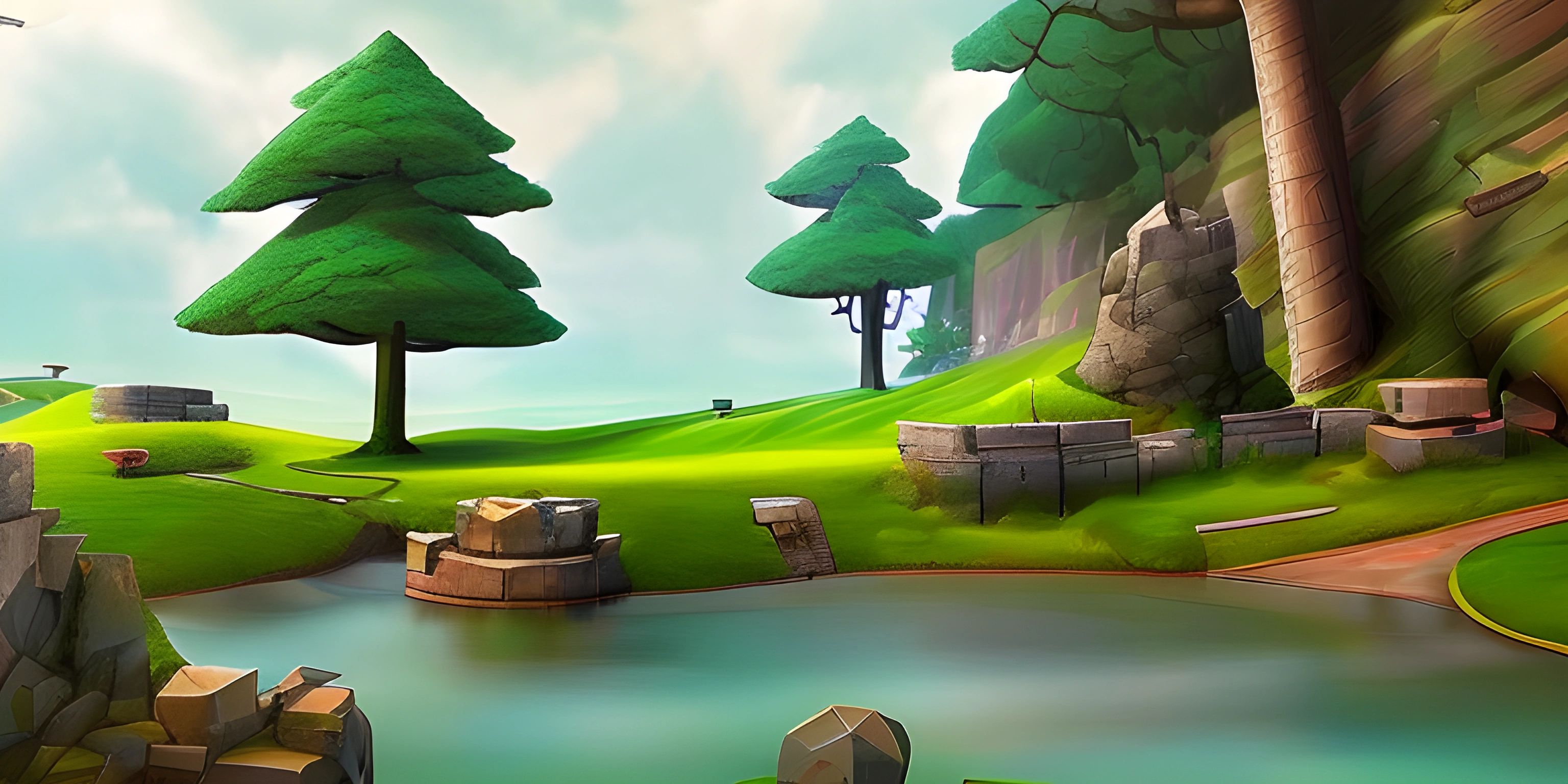
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
C is a versatile and powerful programming language, but with great power comes great responsibility. Writing clean, maintainable, and reliable C code requires a solid understanding of the language and attention to best practices. In this guide, we'll cover essential tips and techniques to help you avoid common pitfalls and write code that stands the test of time.
Use Descriptive Variable and Function Names
Clear, descriptive names make your code easier to read and understand. When choosing names for variables and functions, try to be as descriptive as possible without making them overly long. For example, instead of using i
and j
as loop counters, use names that reflect their purpose, like row
and column
.
for (int row = 0; row < num_rows; row++) { for (int column = 0; column < num_columns; column++) { // Do something with matrix[row][column] } }
Keep Functions Short and Focused
Each function should have a single, well-defined responsibility. By keeping functions short and focused, you make your code easier to understand, test, and debug. If a function seems to be doing too many things, consider breaking it up into smaller, more specialized functions.
Use Static Functions When Possible
Using static functions in C limits their scope to the file they are defined in, making your code more modular and helping to prevent unintended interactions between different parts of your program. This can be particularly useful when working on large projects or when collaborating with other developers.
static int helper_function(int x, int y) { // Implementation }
Error Handling
When writing a function that can return an error, make sure to provide a clear method for the calling code to handle that error. One common approach is to return an error code and use a separate output parameter for the result.
int divide(int x, int y, int *result) { if (y == 0) { return -1; // Error code for division by zero } *result = x / y; return 0; // Success }
Use const Correctly
The const
keyword in C allows you to declare that a variable or function parameter should not be modified. Using const
can help you catch unintended modifications in your code and make your intentions clear to other developers.
int find_max(const int *array, int length) { int max = array[0]; for (int i = 1; i < length; i++) { if (array[i] > max) { max = array[i]; } } return max; }
Be Mindful of Memory Management
In C, you have direct control over memory allocation and deallocation. Be sure to allocate and deallocate memory correctly to avoid memory leaks and segmentation faults. Use the standard library functions malloc()
, calloc()
, realloc()
, and free()
for dynamic memory management.
int *array = malloc(sizeof(int) * num_elements); if (array == NULL) { // Handle allocation failure } // Use array free(array); // Don't forget to free the memory!
Comment Your Code
Well-placed comments can greatly improve the readability of your code. Use comments to explain the purpose of a section of code or to provide additional context for complex or obscure implementations. Avoid writing comments that simply restate what the code is doing.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
Why is it important to use descriptive variable and function names?
Descriptive names make your code more readable and easier to understand. This helps both you and others when revisiting the code later, making it faster to comprehend and debug.
What is the purpose of using static functions in C?
Static functions in C have their scope limited to the file they are defined in. This makes your code more modular and helps prevent unintended interactions between different parts of your program.
How can you handle errors in C functions?
One approach for handling errors in C functions is to return an error code and use a separate output parameter for the result. This allows the calling code to easily check for and handle errors.