Python Best Practices
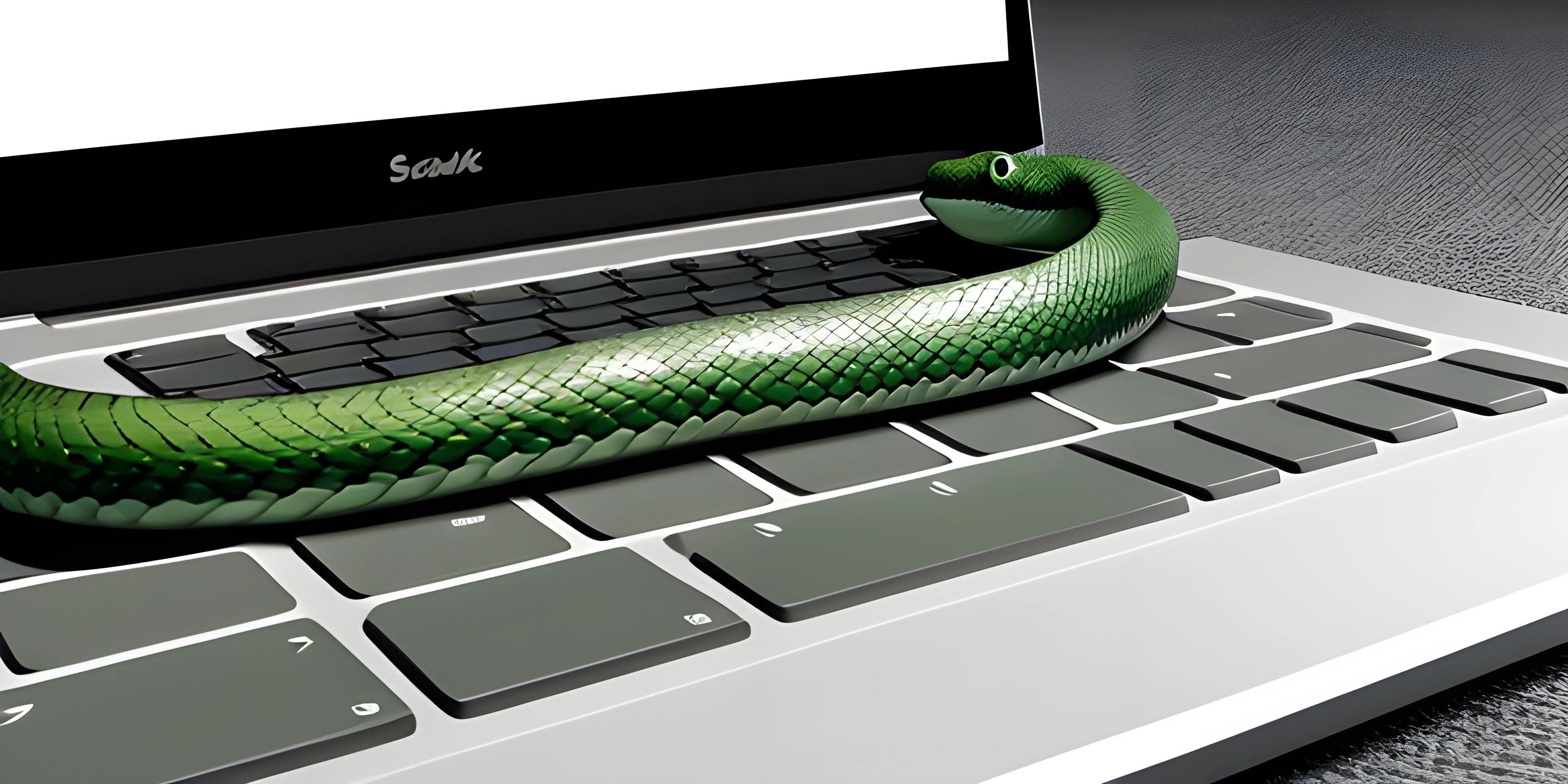
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python is a versatile and powerful programming language, and it's often the go-to choice for developers due to its readability and simplicity. But, as with any language, there are ways to write Python code that's more efficient, easier to understand, and less prone to errors. In this article, we'll explore some Python best practices that will help you write cleaner, more efficient code.
1. Follow the PEP-8 Style Guide
The PEP-8 Style Guide is a widely recognized set of coding conventions for Python that helps make your code more readable and maintainable. Following these guidelines will make your code look professional and easier for others to understand. Some key PEP-8 points include:
- Use 4 spaces for indentation.
- Limit lines to 79 characters.
- Separate functions and classes with two blank lines.
- Use single-line comments starting with a
#
.
Many editors, such as Visual Studio Code, can automatically apply PEP-8 rules as you type.
2. Use Descriptive Variable and Function Names
Choose clear, descriptive names for your variables and functions. This makes your code easier to understand and maintain. For example, use word_list
instead of wl
and calculate_average
instead of calc_avg
. Stick to lowercase names with underscores for variables and functions, and use CamelCase for class names, following the PEP-8 guidelines.
# Good def calculate_average(numbers): return sum(numbers) / len(numbers) # Bad def calc_avg(n): return sum(n) / len(n)
3. Write Modular Code
Break down your code into small, manageable functions that do one specific task. This keeps your code organized, easier to debug, and makes it more reusable. For example, instead of writing a long function to process a list of numbers, break it down into smaller functions for filtering, sorting, and calculating the average.
def filter_even_numbers(numbers): return [num for num in numbers if num % 2 == 0] def sort_numbers(numbers): return sorted(numbers) def process_numbers(numbers): even_numbers = filter_even_numbers(numbers) sorted_numbers = sort_numbers(even_numbers) average = calculate_average(sorted_numbers) return average
4. Use List Comprehensions
Python's list comprehensions allow you to create lists in a more concise, readable way. Use them instead of for
loops when generating lists from other lists or iterables.
# Good squares = [x * x for x in range(10)] # Bad squares = [] for x in range(10): squares.append(x * x)
However, avoid using overly complex list comprehensions that make the code harder to read.
5. Handle Exceptions Gracefully
When handling exceptions with try
and except
blocks, be specific about the types of exceptions you're catching. This prevents unintentionally catching unrelated errors and makes your code more robust.
# Good try: result = 1 / number except ZeroDivisionError: print("Cannot divide by zero") # Bad try: result = 1 / number except Exception: # Catches all exceptions, even unrelated ones print("An error occurred")
6. Use Docstrings
Include docstrings in your code to provide documentation and explain the purpose of your functions, classes, and modules. This helps others understand your code and makes it more maintainable.
def calculate_average(numbers): """ Calculates the average of a list of numbers. Args: numbers (list): A list of numbers. Returns: float: The average of the numbers. """ return sum(numbers) / len(numbers)
Following these best practices will help you write Python code that's clean, efficient, and less prone to errors. Keep learning and refining your skills, and always be on the lookout for ways to improve your code.
FAQ
What is the PEP-8 Style Guide?
The PEP-8 Style Guide is a set of coding conventions for Python that helps make your code more readable and maintainable. It covers topics such as indentation, line length, and naming conventions.
Why is it important to use descriptive variable and function names?
Using descriptive names for variables and functions makes your code easier to understand and maintain. It helps others quickly grasp the purpose of your code, and can save time when working with large projects or collaborating with others.
What is a list comprehension in Python?
A list comprehension is a concise way to create a list from another list or iterable in Python. It allows you to generate a new list using a single line of code instead of using a for
loop.
How should exceptions be handled in Python?
Exceptions should be handled gracefully using try
and except
blocks. Be specific about the types of exceptions you're catching to prevent unintentionally catching unrelated errors.