Rust Traits Best Practices
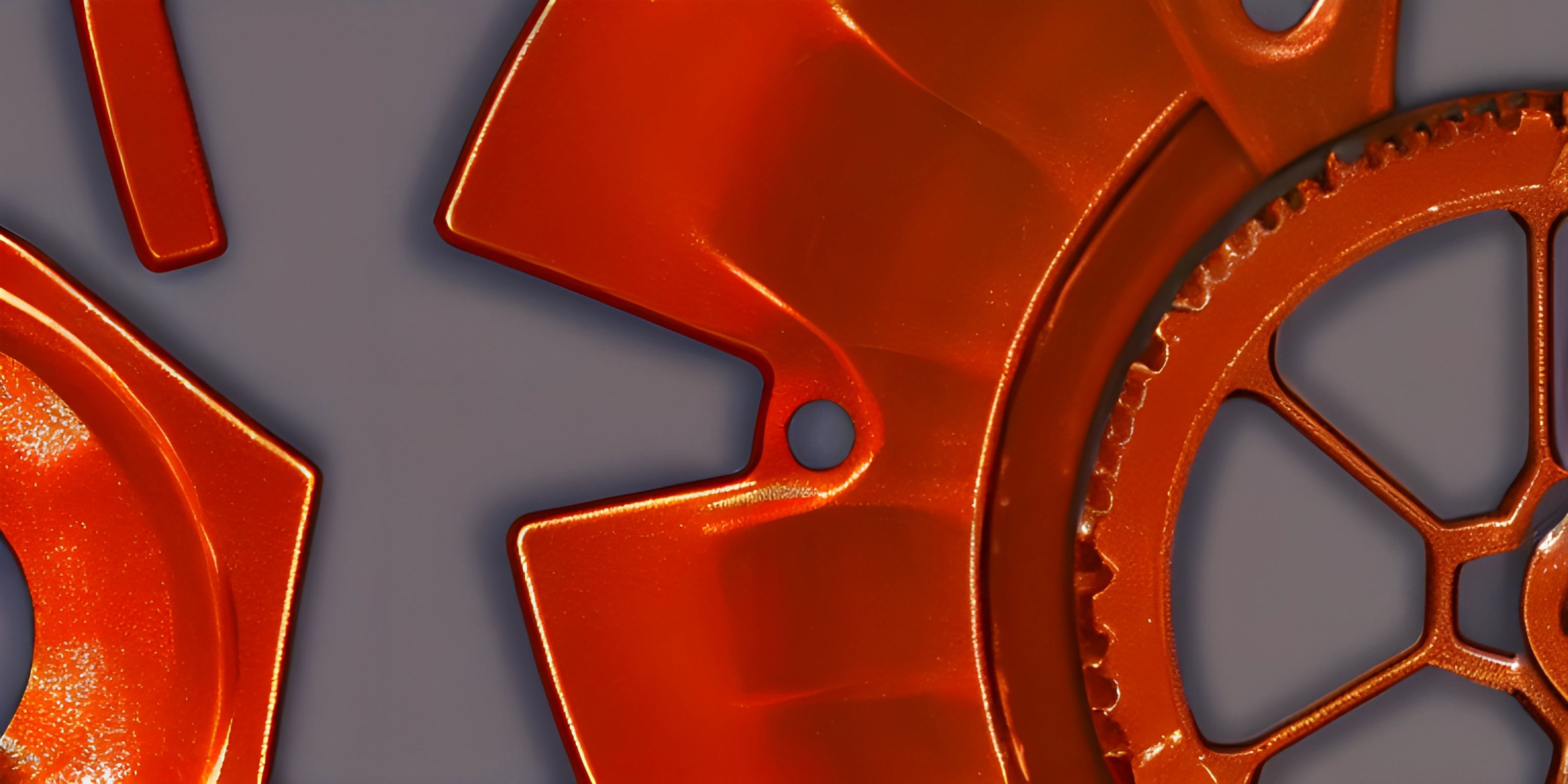
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Traits in Rust are a powerful way to define shared behavior between different data types. They are similar to interfaces in other languages and allow us to write clean, modular, and reusable code. To get the most out of Rust traits, follow these best practices:
1. Keep Traits Small and Focused
When defining a trait, it's essential to keep it small and focused on a specific behavior. This makes it easier to understand and implement for various data types. Avoid creating "god" traits that try to cover too many responsibilities.
// Good: Each trait has a clear, specific purpose trait Drawable { fn draw(&self); } trait Updatable { fn update(&mut self); } // Bad: A single trait with multiple responsibilities trait DrawableUpdatable { fn draw(&self); fn update(&mut self); }
2. Use Trait Bounds Wisely
Trait bounds are used to restrict the types that can implement a specific trait, making it easier to work with generic types. However, it's crucial not to overuse trait bounds, as doing so can lead to unnecessary complexity.
// Good: Using trait bounds when necessary fn display<T: Display>(item: T) { println!("{}", item); } // Bad: Overusing trait bounds fn display<T: Display + Debug + Clone>(item: T) { println!("{:?}", item.clone()); }
3. Leverage Default Implementations
Rust allows you to provide default implementations for trait methods, which can be overridden by implementing types. Use default implementations to provide common behavior that can be shared across different types without repetition.
trait Animal { fn speak(&self) { println!("The animal makes a noise."); } } struct Dog; impl Animal for Dog { fn speak(&self) { println!("Woof!"); } } struct Cat; impl Animal for Cat {} // Uses the default implementation of `speak` fn main() { let dog = Dog; dog.speak(); // "Woof!" let cat = Cat; cat.speak(); // "The animal makes a noise." }
4. Use Associated Types
Associated types allow you to define types within a trait that implementing types must specify. This enables greater flexibility and customization when implementing a trait.
trait Iterator { type Item; fn next(&mut self) -> Option<Self::Item>; } struct Counter { count: u32, } impl Iterator for Counter { type Item = u32; fn next(&mut self) -> Option<Self::Item> { self.count += 1; Some(self.count) } }
5. Implement Traits for References
When implementing traits, consider implementing them for references as well. This can help improve performance by avoiding unnecessary cloning.
trait Greet { fn greet(&self); } impl Greet for &str { fn greet(&self) { println!("Hello, {}!", self); } } fn main() { let name = "Alice"; name.greet(); // Hello, Alice! }
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are traits in Rust?
Traits in Rust are a way to define shared behavior between different data types. They are similar to interfaces in other languages and allow us to write clean, modular, and reusable code.
What is a trait bound?
A trait bound is a restriction placed on the types that can implement a specific trait. It is used to specify that a generic type must implement a certain trait or set of traits, making it easier to work with generic types.
What is an associated type?
An associated type is a type defined within a trait that implementing types must specify. This allows greater flexibility and customization when implementing a trait for different data types.
What is the purpose of default implementations in traits?
Default implementations in traits allow you to provide common behavior that can be shared across different types without repetition. Implementing types can choose to override the default implementation with their own specific behavior.