C Debugging Tips
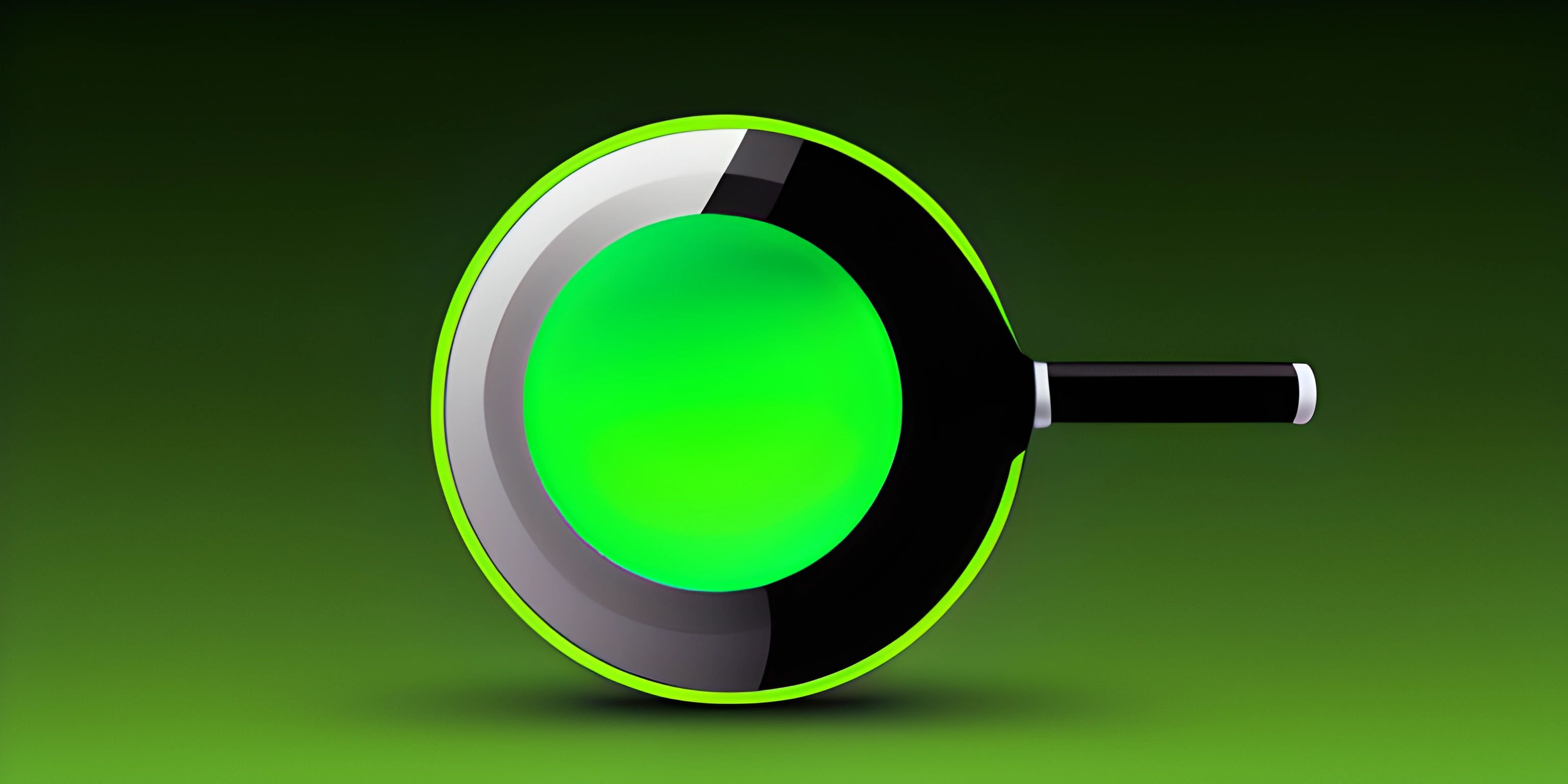
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Getting your code to run smoothly can sometimes feel like trying to solve a puzzle. But fear not! Debugging your C code doesn't have to be a headache. Here are some tips and tricks to help you pinpoint and fix those pesky bugs.
Use a Debugger
A debugger is an essential tool for any C programmer. It allows you to inspect your code as it runs and identify any problems that may occur. Some popular debuggers for C are GDB and LLDB.
With a debugger, you can step through your code line by line or set breakpoints to pause the execution at specific points, allowing you to examine the contents of variables or the call stack.
Here's a simple example of how to use GDB:
#include <stdio.h> int main() { int a = 5; int b = 0; int result = a / b; printf("Result: %d", result); return 0; }
This code has a division by zero error. Let's see how GDB can help us identify the problem:
- Compile your code with the
-g
flag to include debugging information:
gcc -g sample.c -o sample
- Run GDB with the compiled binary:
gdb sample
- Set a breakpoint at the line with the division operation:
break 6
- Run the program:
run
- When the breakpoint is hit, check the values of
a
andb
:
print a print b
Now you can see that b
holds the value 0
, which will cause a division by zero error. Using the debugger, you can quickly identify and fix this issue.
Print Statements
Sometimes, the quickest way to identify an issue is by adding print statements to your code. This can be helpful to monitor the values of variables or track the flow of execution. Use printf()
to output valuable information to the console:
#include <stdio.h> int main() { int a = 5; int b = 0; printf("a: %d, b: %d\n", a, b); int result = a / b; printf("Result: %d", result); return 0; }
Running this code will show the values of a
and b
, indicating that the division by zero is causing the problem.
Code Review and Rubber Duck Debugging
Sometimes, the best way to find a bug is to explain your code to someone else or even to an inanimate object like a rubber duck. This technique is called Rubber Duck Debugging.
By articulating your thought process and walking through each line of code, you may suddenly realize where the issue lies. This can be a surprisingly effective method for spotting logic errors or identifying incorrect variable assignments.
Divide and Conquer
If you're having trouble finding the root cause of a bug, try isolating different sections of your code. By commenting out or temporarily removing parts of your code, you can narrow down the location of the issue. This process of elimination will help you focus on the problematic area and fix the bug more efficiently.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are some effective methods for debugging C code?
Some effective methods for debugging C code include using a debugger like GDB or LLDB, adding print statements to monitor variable values or code execution flow, conducting code reviews or using Rubber Duck Debugging, and isolating different sections of your code to identify the problematic area.
What is Rubber Duck Debugging?
Rubber Duck Debugging is a technique where you explain your code line-by-line to an inanimate object, such as a rubber duck. By articulating your thought process and walking through each line of code, you may suddenly realize where the issue lies, helping you spot logic errors or identify incorrect variable assignments.
How can a debugger like GDB or LLDB help in troubleshooting C code?
Debuggers like GDB or LLDB allow you to step through your code line by line, set breakpoints to pause the execution at specific points, and examine the contents of variables or the call stack. This helps you identify any problems that may occur during the execution of your code and fix them accordingly.