Exploring the C Standard Library
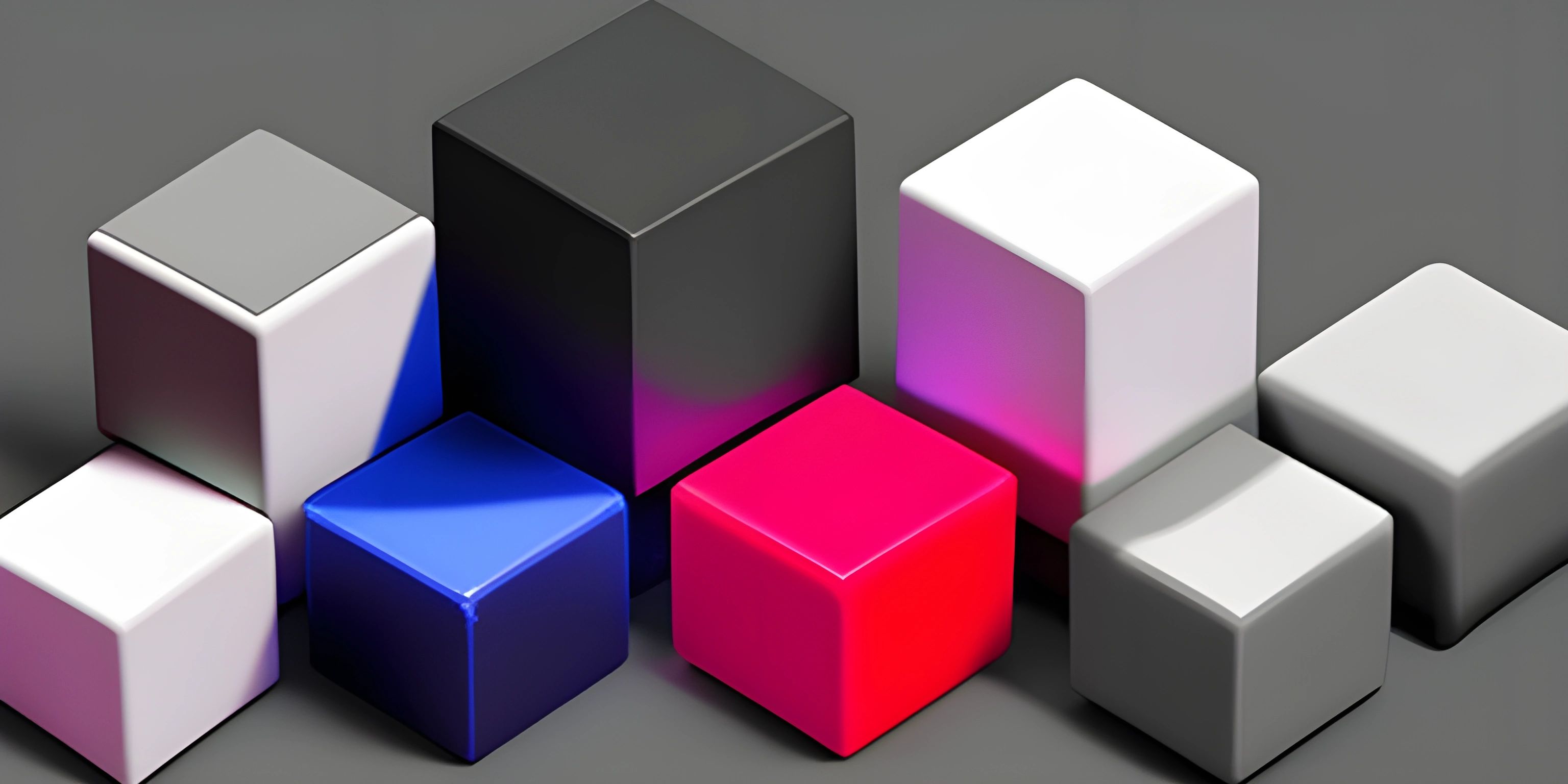
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Every programming language has its own set of built-in tools and utilities to simplify the development process. In C, we have the C Standard Library, which is a collection of header files containing functions, macros, and other helpful utilities. It's like a Swiss Army knife for C programming, helping you tackle a wide range of tasks without having to reinvent the wheel.
Header Files and Their Purpose
In the C Standard Library, functionality is grouped into header files. These files act as a gateway to access the functions and other utilities contained within them. To use a specific feature, you simply #include
the corresponding header file at the beginning of your C program. Let's dive into a few common header files and their features.
stdio.h
stdio.h
stands for Standard Input/Output, and it's a cornerstone of the C Standard Library. This header file contains functions for working with files, console input/output, and more. For example, the classic "Hello, World!" program relies on the printf()
function, which is part of stdio.h
:
#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
stdlib.h
stdlib.h
is all about Standard Library functions, which deal with memory allocation, number conversion, and other miscellaneous tasks. For instance, it provides the malloc()
function for dynamic memory allocation, and the rand()
function for generating random numbers.
#include <stdio.h> #include <stdlib.h> int main() { int random_number = rand(); printf("Random number: %d\n", random_number); return 0; }
string.h
As the name suggests, string.h
focuses on string manipulation functions. It includes utilities for comparing, copying, and concatenating strings, as well as finding the length of a string. Here's an example that showcases the strlen()
function:
#include <stdio.h> #include <string.h> int main() { char my_string[] = "Cratecode"; printf("Length of the string: %lu\n", strlen(my_string)); return 0; }
Common Standard Library Functions
There are many functions in the C Standard Library, but a few stand out as particularly useful across a wide range of applications. Get familiar with these, and you'll be well-equipped to handle a variety of programming challenges:
printf()
andscanf()
(fromstdio.h
) for formatted input and output.strcmp()
andstrcpy()
(fromstring.h
) for string comparison and copying.malloc()
andfree()
(fromstdlib.h
) for dynamic memory allocation and deallocation.qsort()
(fromstdlib.h
) for quick and efficient sorting of arrays.
A World of Possibilities
The C Standard Library is vast and feature-rich, and we've only scratched the surface here. As you progress in your C programming journey, you'll discover an abundance of helpful functions and utilities tucked away in its many header files. Consult C documentation to explore these treasures and elevate your code to new heights.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the C Standard Library?
The C Standard Library is a collection of header files containing functions, macros, and other utilities that simplify the development process in C.
How do I use a function from the C Standard Library in my program?
To use a function from the C Standard Library, simply #include
the corresponding header file at the beginning of your C program.
Which header file contains functions for string manipulation?
The string.h
header file focuses on string manipulation functions.
What are some common functions in the C Standard Library?
Some common functions in the C Standard Library include printf()
, scanf()
, strcmp()
, strcpy()
, malloc()
, free()
, and qsort()
.