Calling Conventions
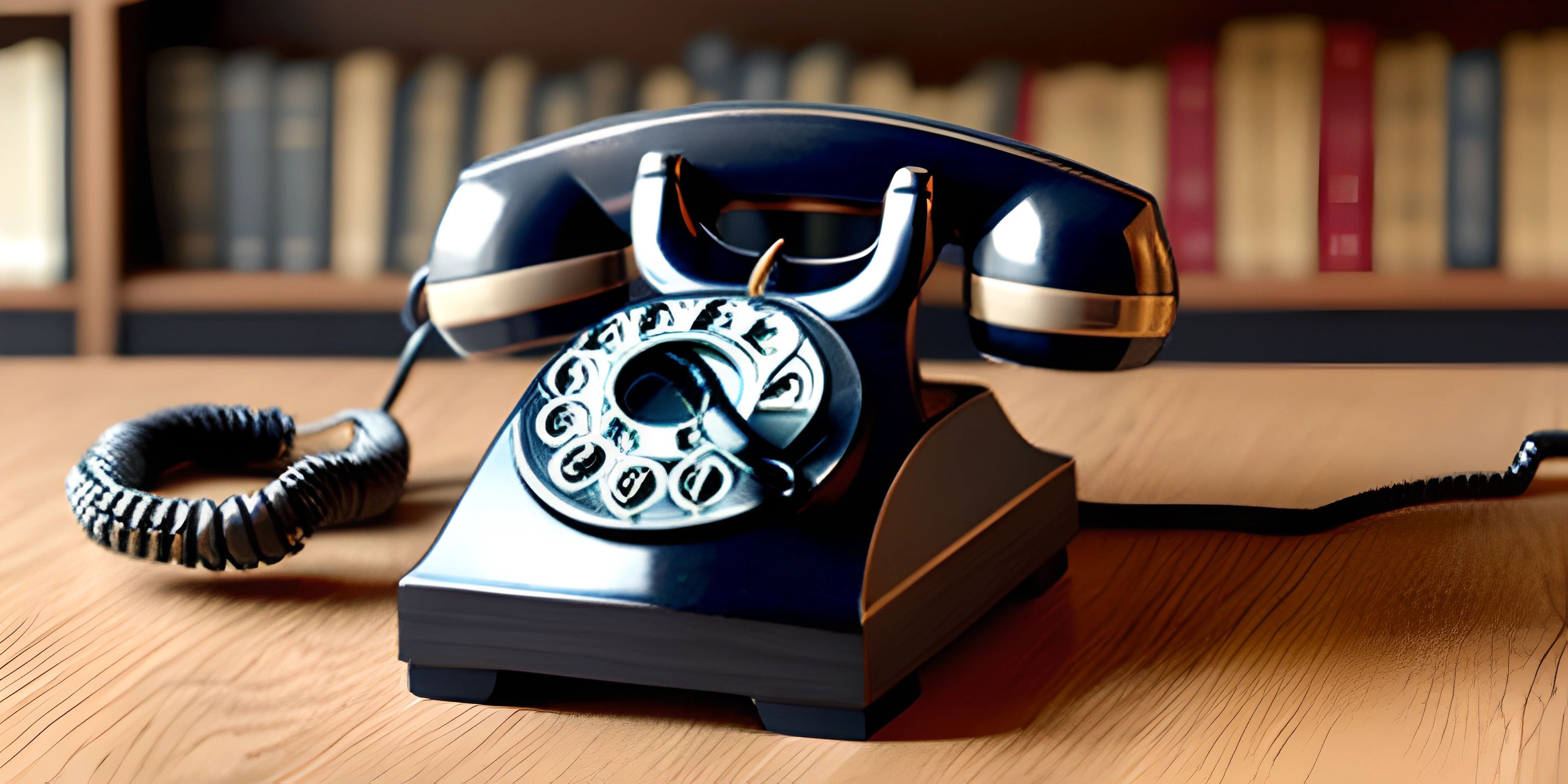
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the programming world, communication is key, not only between developers but also between different parts of a program. One important aspect of this communication is the way functions or methods are called and how they interact with each other. Enter the realm of calling conventions.
What are Calling Conventions?
Calling conventions are a set of rules that dictate how functions or methods communicate with each other during their execution. They define the order in which arguments are passed, how the return value is managed, and how the program's stack is maintained. In essence, calling conventions allow for consistency and predictability, which is crucial for a well-structured and maintainable program.
The calling conventions are particularly important when using different programming languages, libraries, or even compilers within the same project. They ensure that all parts of the program understand and follow the same rules, preventing any unexpected behavior or crashes.
Why are Calling Conventions Important?
Picture a world where you're attending a party with people speaking multiple languages. To communicate effectively, everyone must agree on which language to use and the rules of the conversation. In the programming world, calling conventions play a similar role, ensuring that different parts of a program can effectively "speak" and "listen" to each other.
Here are some key benefits of using calling conventions:
- Consistency: They provide a uniform way for methods or functions to interact, regardless of the programming language or compiler used.
- Interoperability: They enable smooth communication between different programming languages, libraries, and compilers within a project.
- Optimization: They aid in creating efficient code by reducing the overhead of function calls and improving the program's overall performance.
- Maintainability: They make it easier for developers to understand and manage the codebase, particularly when working with large projects or collaborating with other developers.
Common Calling Conventions
There are several calling conventions used across different programming languages and platforms. Some of the most common ones include:
-
Cdecl: Short for "C declaration," cdecl is the default calling convention for the C programming language. It passes arguments from right to left on the stack and requires the caller to clean up the stack after the function call.
-
Stdcall: Used primarily in the Windows API, stdcall passes arguments from right to left on the stack, like cdecl. However, the called function is responsible for cleaning up the stack, making it more efficient for fixed-arity functions (functions with a fixed number of arguments).
-
Fastcall: Designed for performance, fastcall attempts to use CPU registers to pass arguments whenever possible, reducing the overhead of stack manipulation. This convention is usually platform-dependent, with different implementations for different architectures.
-
Thiscall: Specific to C++ class member functions, thiscall passes the
this
pointer (an instance of the class) in a CPU register, with the rest of the arguments passed using conventions like cdecl or stdcall. -
Application Binary Interface (ABI): ABIs are platform-specific calling conventions that dictate how a compiled program interacts with the system at runtime. They define not only function calling rules, but also data representation, memory layout, and system libraries. Examples of well-known ABIs include the System V ABI (used on Unix systems) and the Microsoft x64 ABI.
Conclusion
Calling conventions play a critical role in ensuring smooth communication between different parts of a program. By understanding and adhering to these conventions, developers can create consistent, interoperable, and maintainable code. So the next time you're at a programming party, remember the importance of speaking the same language and following the rules of the conversation – your code will thank you!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What are calling conventions and why are they important?
Calling conventions are a set of rules that dictate how functions should be called and how they should return values in various programming languages. They define how parameters are passed, how the function caller cleans up the stack, and how the return values are handled. Calling conventions are important because they ensure that different functions and libraries can work together seamlessly, keeping the code organized, efficient, and compatible across different compilers and platforms.
What are some common calling conventions?
There are several common calling conventions, including:
- cdecl (C declaration) - The default calling convention for C programs. Parameters are passed from right to left, and the caller is responsible for cleaning up the stack.
- stdcall - The standard calling convention for Windows APIs. Parameters are passed from right to left, and the callee is responsible for cleaning up the stack.
- fastcall - A convention that aims to optimize function calls by passing the first few arguments in registers instead of the stack.
- thiscall - The default calling convention for C++ member functions. The 'this' pointer is passed in a register, and the callee cleans up the stack.
Can I use different calling conventions in the same program?
Yes, you can use different calling conventions in the same program. However, it's important to ensure that the functions using different conventions are correctly declared and called. Mixing calling conventions without proper declaration can lead to unexpected behavior, crashes, or data corruption. Most compilers provide a way to specify the calling convention of a function using keywords or attributes.
How do I specify a calling convention for a function in C or C++?
In C or C++, you can specify a calling convention for a function using specific keywords or compiler-specific attributes. For example, in Microsoft Visual C++, you can use the following syntax:
// cdecl (default in C) void __cdecl my_function(int x, int y); // stdcall (common in Windows APIs) void __stdcall my_function(int x, int y); // fastcall (passes some arguments in registers) void __fastcall my_function(int x, int y);
For GCC, you can use the following syntax:
// cdecl void __attribute__((cdecl)) my_function(int x, int y); // stdcall void __attribute__((stdcall)) my_function(int x, int y); // fastcall void __attribute__((fastcall)) my_function(int x, int y);
Keep in mind that these conventions might not be supported or may have different keywords depending on the compiler you're using.
Are calling conventions platform-specific?
Calling conventions can be platform-specific or platform-independent. Some calling conventions, like cdecl and stdcall, are widely used across different platforms, while others may be specific to certain operating systems or hardware architectures. When writing cross-platform code, it's important to be aware of the calling conventions supported by your target platforms and, if necessary, use conditional compilation to ensure compatibility.