Making an LED Blink with Arduino
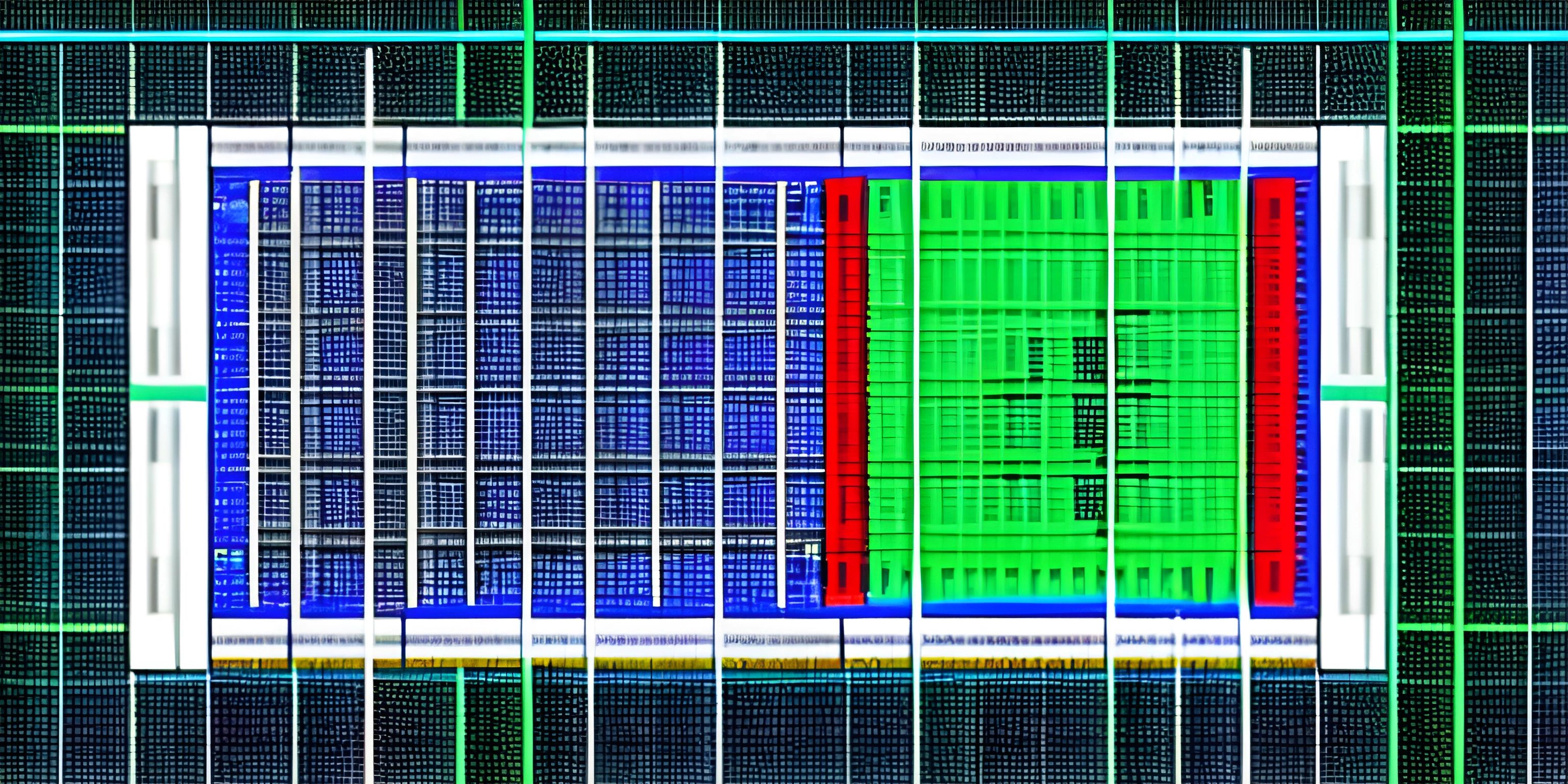
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ready to make your first LED blink with Arduino? It's a rite of passage for anyone diving into the world of microcontrollers. Think of it as the "Hello, World!" of hardware. In this article, we'll cover the basics of using Arduino and C++ to get that little light flashing. Buckle up—it's going to be illuminating!
First things first, you'll need:
- An Arduino board (like the Uno)
- An LED
- A resistor (220 ohms works well)
- Breadboard and jumper wires
Setting Up Your Circuit
Before we dive into the code, let’s set up the hardware. Think of it like building Lego—you need the right pieces in the right places.
- Place the LED on the breadboard: Make sure the longer leg (anode) is in a different row from the shorter leg (cathode).
- Connect the resistor: Attach one end to the anode of the LED and the other end to a row on the breadboard.
- Connect the jumper wires:
- One wire from the free end of the resistor to pin 13 on the Arduino.
- Another wire from the cathode (shorter leg) of the LED to a GND (ground) pin on the Arduino.
Voilà! Your circuit board is ready to blink. Now, on to the code.
The Code
Fire up your Arduino IDE. If you don't have it yet, you can download it from the official Arduino website.
Here’s a simple sketch to make your LED blink:
// Define the LED pin const int LED_PIN = 13; void setup() { // Initialize the digital pin as an output pinMode(LED_PIN, OUTPUT); } void loop() { // Turn the LED on (HIGH is the voltage level) digitalWrite(LED_PIN, HIGH); // Wait for a second delay(1000); // Turn the LED off by making the voltage LOW digitalWrite(LED_PIN, LOW); // Wait for a second delay(1000); }
Breaking Down the Code
Setup Function: The setup()
function runs once when the sketch starts. We use it to initialize the LED pin as an output pin.
Loop Function: The loop()
function runs over and over again, forever (or until you turn off the power). Here, we're turning the LED on and off with one-second delays in between.
Uploading Code to Arduino
- Connect your Arduino to your computer using a USB cable.
- In the Arduino IDE, select your board and port from the Tools menu.
- Click the upload button (the right arrow icon).
If everything is set up correctly, your LED should start blinking!
Troubleshooting Tips
- LED not blinking? Double-check your wiring. Ensure the LED is correctly oriented—long leg (anode) to the resistor and short leg (cathode) to GND.
- Error uploading code? Make sure the correct board and port are selected in the Arduino IDE.
Expanding the Project
Want to make things more exciting? Try changing the delay times to make different blink patterns!
void loop() { digitalWrite(LED_PIN, HIGH); delay(500); // Wait for half a second digitalWrite(LED_PIN, LOW); delay(200); // Wait for 0.2 seconds }
Or, how about using a for loop to create a blinking sequence?
void loop() { for (int i = 0; i < 5; i++) { digitalWrite(LED_PIN, HIGH); delay(300); digitalWrite(LED_PIN, LOW); delay(300); } delay(2000); // Wait for 2 seconds before the next sequence }
Going Further
Now that you've mastered the basics, you can move on to more advanced projects like controlling multiple LEDs or using sensors. The possibilities are endless!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is the purpose of the resistor in the circuit?
The resistor limits the current flowing through the LED to prevent it from burning out. Without the resistor, too much current could pass through the LED, damaging it.
Can I use a different pin other than pin 13 for the LED?
Yes, you can use any digital pin on the Arduino. Just make sure to update the LED_PIN
constant in your code to match the pin you’re using.
What if I want to control multiple LEDs?
You can control multiple LEDs by connecting each one to a different digital pin and defining the pins in your code. Then, use digitalWrite()
and pinMode()
for each LED just as you did for the single LED.
How can I make the LED blink faster or slower?
Adjust the delay times in the loop()
function. Shorter delays will make the LED blink faster, while longer delays will make it blink slower.
What if I don't have an LED or resistor?
You can still practice writing and uploading code to your Arduino. The built-in LED on pin 13 of most Arduino boards can be used as a substitute. Just remove the external LED and resistor from your circuit and the code will still make the built-in LED blink.