C++ Common Error Messages
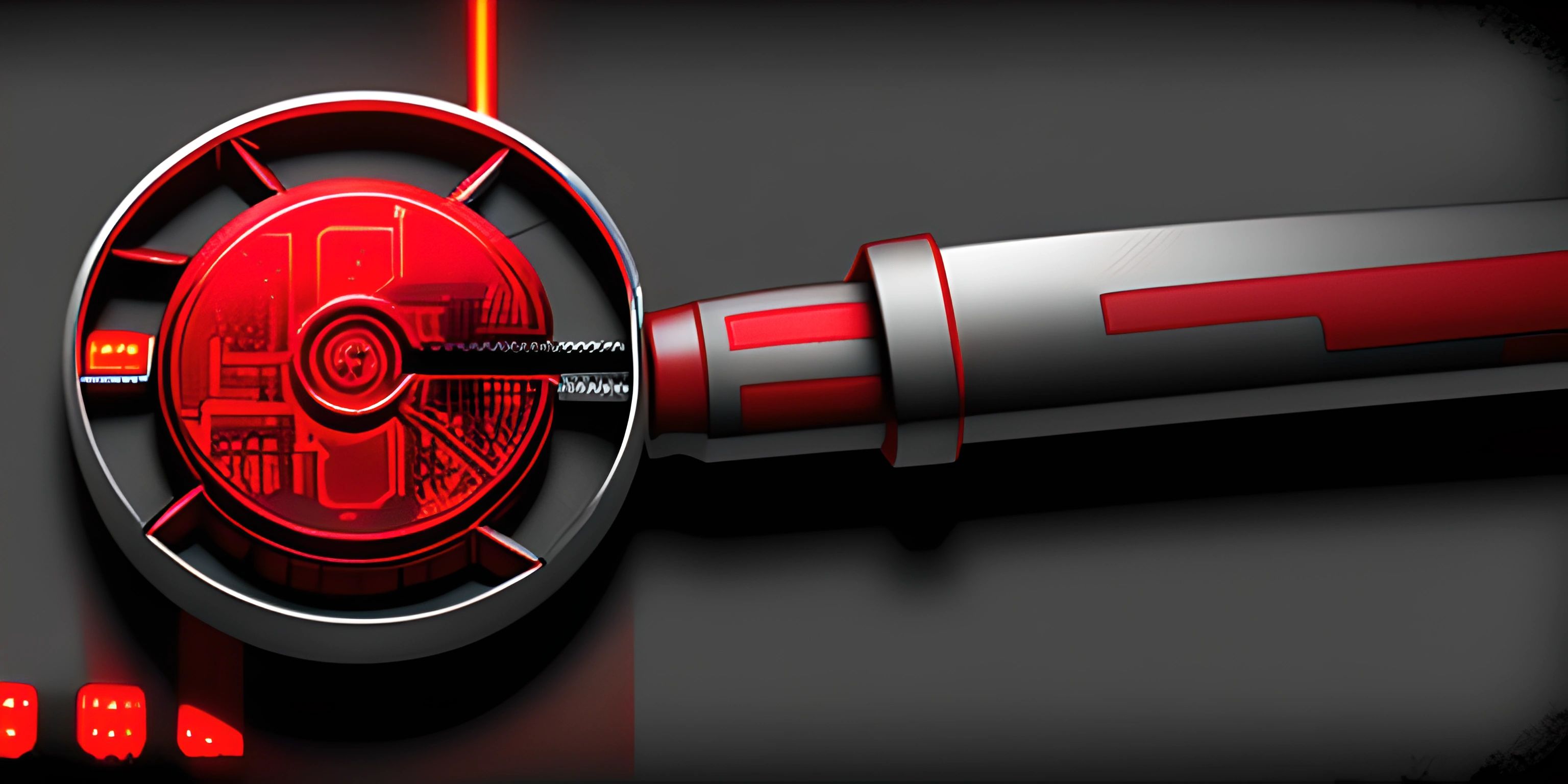
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming in C++ can sometimes be a daunting task, especially when you encounter error messages that you don't understand. Fear not! This article will help you decipher some of the most common error messages in C++ and guide you on how to fix them.
1. Syntax Errors
Syntax errors are mistakes in the structure of your code, often caused by typos, missing characters, or incorrect usage of language elements. Here are some common syntax errors:
a. Missing Semicolon
C++ requires a semicolon ";" at the end of each statement. A common error is forgetting to add one:
#include <iostream> int main() { std::cout << "Hello, world!" // Missing semicolon return 0; }
Fix: Add the missing semicolon.
#include <iostream> int main() { std::cout << "Hello, world!"; return 0; }
b. Mismatched Parentheses, Braces, or Brackets
Opening and closing parentheses "()", braces "{}", and brackets "[]" should always come in pairs. Mismatched or missing ones will trigger a syntax error:
#include <iostream> int main() { int arr[] = {1, 2, 3; // Missing closing bracket return 0; }
Fix: Make sure all opening and closing characters are properly matched.
#include <iostream> int main() { int arr[] = {1, 2, 3}; // Fixed return 0; }
2. Semantic Errors
Semantic errors occur when your code is syntactically correct but doesn't make sense in the context of the language rules. Here are some common semantic errors:
a. Undeclared Identifier
This error happens when you try to use a variable or function before declaring it:
#include <iostream> int main() { myFunction(); // Undeclared identifier return 0; } void myFunction() { std::cout << "Function called!"; }
Fix: Declare the function or variable before using it, or include the appropriate header file.
#include <iostream> void myFunction(); // Function declaration int main() { myFunction(); // Now it's declared return 0; } void myFunction() { std::cout << "Function called!"; }
b. Type Mismatch
A type mismatch occurs when you try to assign a value of one type to a variable of a different type:
#include <iostream> int main() { int num = "Hello, world!"; // Type mismatch return 0; }
Fix: Make sure the value being assigned matches the variable type.
#include <iostream> int main() { std::string text = "Hello, world!"; // Fixed return 0; }
3. Linker Errors
Linker errors are related to the process of combining object files and libraries to create an executable program. Here are some common linker errors:
a. Undefined Reference
This error occurs when the linker cannot find a definition for a function or variable:
#include <iostream> void myFunction(); int main() { myFunction(); // Undefined reference return 0; }
Fix: Ensure that the function or variable is properly defined and included.
#include <iostream> void myFunction(); // Function declaration int main() { myFunction(); // Now it's declared return 0; } void myFunction() { // Function definition std::cout << "Function called!"; }
b. Multiple Definitions
This error happens when a function or variable has been defined more than once:
#include <iostream> void myFunction(); int main() { myFunction(); return 0; } void myFunction() { std::cout << "Function called!"; } void myFunction() { // Multiple definitions std::cout << "Another function with the same name!"; }
Fix: Remove the duplicate definition.
#include <iostream> void myFunction(); int main() { myFunction(); return 0; } void myFunction() { std::cout << "Function called!"; }
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are the three main types of error messages in C++?
The three main types of error messages in C++ are syntax errors, semantic errors, and linker errors.
What is a syntax error?
A syntax error is a mistake in the structure of your code, often caused by typos, missing characters, or incorrect usage of language elements. Examples include missing semicolons and mismatched parentheses.
What is a semantic error?
A semantic error occurs when your code is syntactically correct but doesn't make sense in the context of the language rules. Examples include undeclared identifiers and type mismatches.
What is a linker error?
A linker error is related to the process of combining object files and libraries to create an executable program. Examples include undefined references and multiple definitions.
How can I fix an "undeclared identifier" error?
To fix an "undeclared identifier" error, declare the function or variable before using it or include the appropriate header file.