Javascript Common Error Messages and Fixes
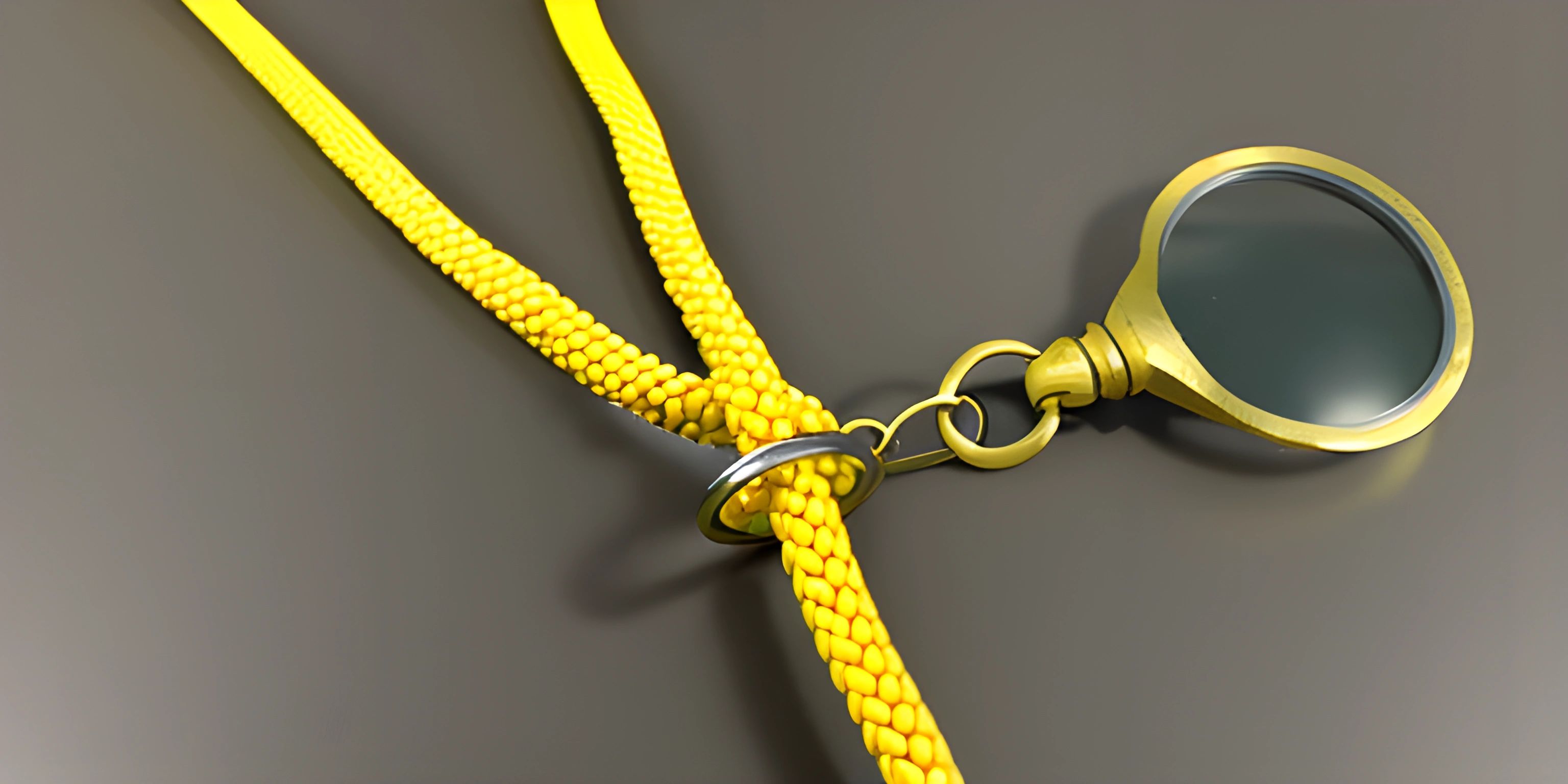
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Debugging is an essential part of programming, and JavaScript is no exception. When you encounter errors in your code, it's crucial to understand the error messages you receive and how to fix them. Let's dive into some common JavaScript error messages and their solutions.
ReferenceError: Undefined Variable
This error occurs when you try to use a variable that hasn't been declared or is misspelled. For example:
console.log(nonExistentVariable);
To fix this error, make sure the variable is correctly spelled and declared before using it. In this case, declare nonExistentVariable
first:
let nonExistentVariable = "Hello, JavaScript!"; console.log(nonExistentVariable);
TypeError: Cannot read property 'x' of undefined
This error typically occurs when you try to access a property or method on an undefined object. For example:
let user; console.log(user.name);
To fix this error, ensure the object is properly defined before accessing its properties:
let user = { name: "John Doe" }; console.log(user.name);
SyntaxError: Unexpected token
This error usually indicates a syntax issue, such as a missing parenthesis, semicolon, or curly brace. For example:
function greet() { console.log("Hello, JavaScript!" }
In this case, the error occurs because a closing parenthesis is missing after the console.log statement. To fix it, add the missing parenthesis:
function greet() { console.log("Hello, JavaScript!"); }
RangeError: Maximum call stack size exceeded
This error occurs when a function calls itself recursively without a terminating condition, causing an infinite loop. For example:
function recursiveFunction() { recursiveFunction(); } recursiveFunction();
To fix this error, ensure your recursive function has a terminating condition:
function recursiveFunction(n) { if (n <= 0) { return; } console.log(n); recursiveFunction(n - 1); } recursiveFunction(5);
URIError: Malformed URI
This error occurs when you pass an incorrect URI to methods like encodeURI()
or decodeURI()
. For example:
let malformedURI = "https://%test.com"; console.log(decodeURI(malformedURI));
To fix this error, make sure the URI is correctly formatted before passing it to the method:
let validURI = "https://test.com"; console.log(decodeURI(validURI));
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is the "ReferenceError: Undefined Variable" error in JavaScript?
It occurs when you try to use a variable that hasn't been declared or is misspelled. To fix this error, make sure the variable is correctly spelled and declared before using it.
What is the "TypeError: Cannot read property 'x' of undefined" error in JavaScript?
This error typically occurs when you try to access a property or method on an undefined object. To fix this error, ensure the object is properly defined before accessing its properties.
What is the "SyntaxError: Unexpected token" error in JavaScript?
This error usually indicates a syntax issue, such as a missing parenthesis, semicolon, or curly brace. To fix it, carefully examine your code and add or modify the missing syntax elements.
What is the "RangeError: Maximum call stack size exceeded" error in JavaScript?
This error occurs when a function calls itself recursively without a terminating condition, causing an infinite loop. To fix this error, ensure your recursive function has a terminating condition.
What is the "URIError: Malformed URI" error in JavaScript?
This error occurs when you pass an incorrect URI to methods like encodeURI()
or decodeURI()
. To fix this error, make sure the URI is correctly formatted before passing it to the method.