Common C Error Messages
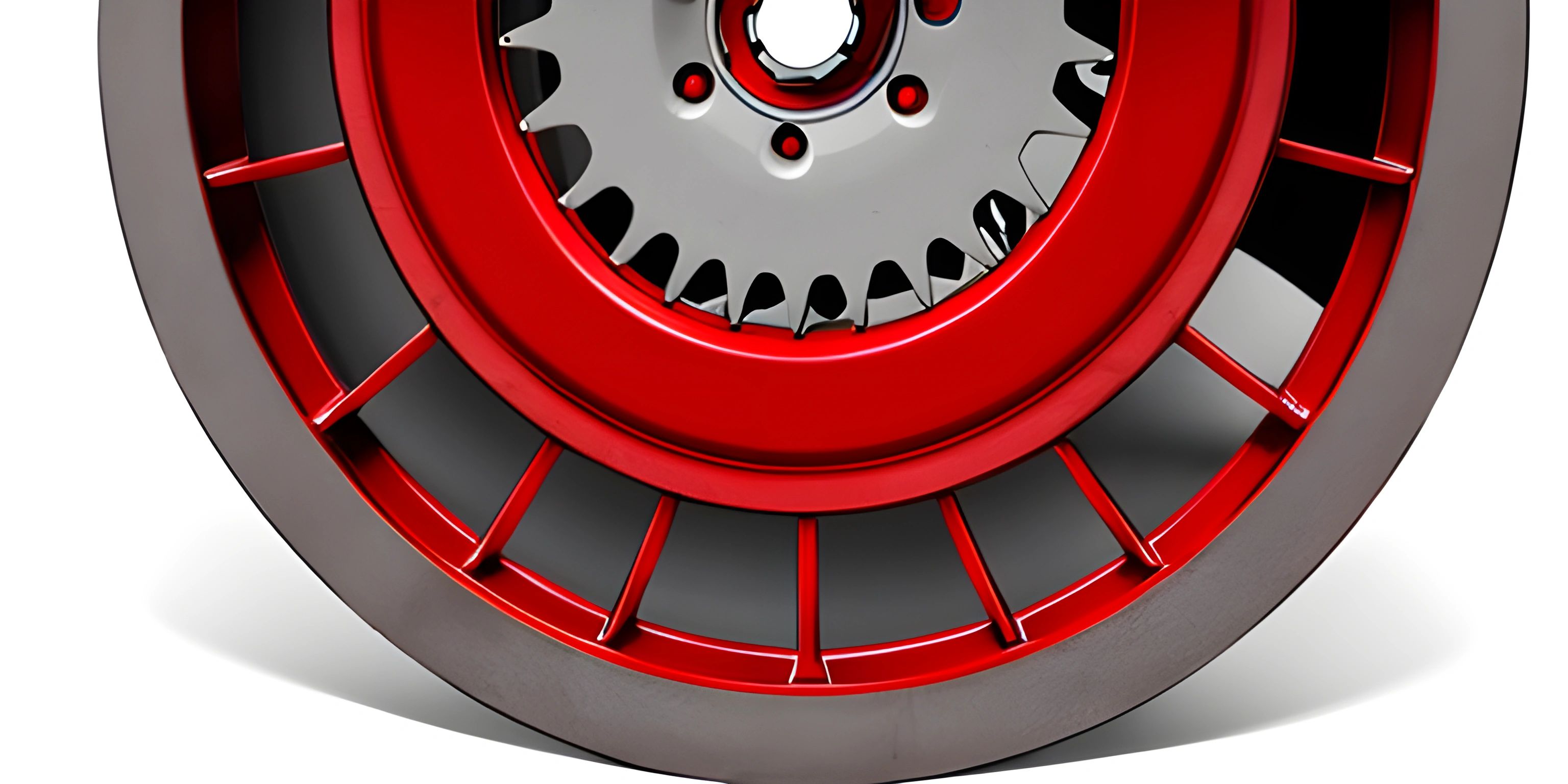
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming in C can sometimes feel like navigating a minefield. Errors are lurking around every corner, waiting to trip you up. But fear not, as we'll help you decipher those cryptic error messages and arm you with the tools to fix them. Buckle up!
Syntax Errors
Syntax errors arise when the compiler encounters code that doesn't follow C's grammar rules. Just like how human languages have grammar, so do programming languages.
Missing Semicolon
A semicolon (;
) is required at the end of each statement. If you forget it, you might see an error like this:
error: expected ‘;’ before ‘return’
In this case, you're missing a semicolon before a return
statement. To fix it, simply add a semicolon:
printf("Hello, World!"); return 0;
Mismatched Brackets
Mismatched brackets {}
are another common syntax error. The error message might look like this:
error: expected ‘}’ before ‘else’
This means you've missed a closing brace }
before an else
statement. To fix this, add the missing brace:
if (x > 0) { printf("Positive"); } else { printf("Negative"); }
Semantic Errors
Semantic errors occur when your code is syntactically correct but doesn't make logical sense. These can be trickier to spot because the compiler can't always provide specific guidance.
Undefined Reference
An undefined reference error occurs when you try to use a function or variable that hasn't been defined yet. The error message could look like this:
error: undefined reference to ‘some_function’
To fix this, make sure the function or variable is defined before it's used. You can either define it in your code or include the appropriate header file if it's from an external library:
#include "some_library.h" int main() { some_function(); return 0; }
Implicit Declaration
An implicit declaration error occurs when you call a function without declaring its prototype. The error message might look like this:
warning: implicit declaration of function ‘some_function’
To fix this, add a function prototype before the function call:
void some_function(); // Function prototype int main() { some_function(); return 0; }
Runtime Errors
Runtime errors happen while your program is running and can cause it to crash or produce incorrect results.
Segmentation Fault
A segmentation fault occurs when your program tries to access memory it shouldn't. This error message is common:
Segmentation fault (core dumped)
To fix this, double-check your pointers and array indices to ensure you're not accessing memory out of bounds.
int arr[5]; arr[6] = 42; // This will cause a segmentation fault
Division by Zero
A division by zero error happens when you attempt to divide by zero, which is not allowed. The error message might look like this:
Floating point exception (core dumped)
To fix this, ensure you're not dividing by zero by adding a conditional check:
if (denominator != 0) { result = numerator / denominator; } else { printf("Division by zero is not allowed."); }
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are the three main categories of C error messages?
The three main categories of C error messages are syntax errors, semantic errors, and runtime errors. Syntax errors are caused by code that doesn't follow C's grammar rules, semantic errors occur when the code is syntactically correct but doesn't make logical sense, and runtime errors happen while the program is running and can cause crashes or incorrect results.
How can I fix a missing semicolon error?
To fix a missing semicolon error, simply add a semicolon (;
) at the end of the statement where the error occurs. The error message will usually indicate the line and position where the semicolon is expected.
What causes an undefined reference error?
An undefined reference error occurs when you try to use a function or variable that hasn't been defined yet. To fix this error, make sure the function or variable is defined before it's used or include the appropriate header file if it's from an external library.
How can I prevent a segmentation fault?
To prevent a segmentation fault, ensure that your program doesn't access memory it shouldn't. Double-check your pointers and array indices to make sure you're not accessing memory out of bounds. Also, make sure you properly allocate and deallocate memory when using dynamic memory allocation functions like malloc
and free
.