Python Common Error Messages
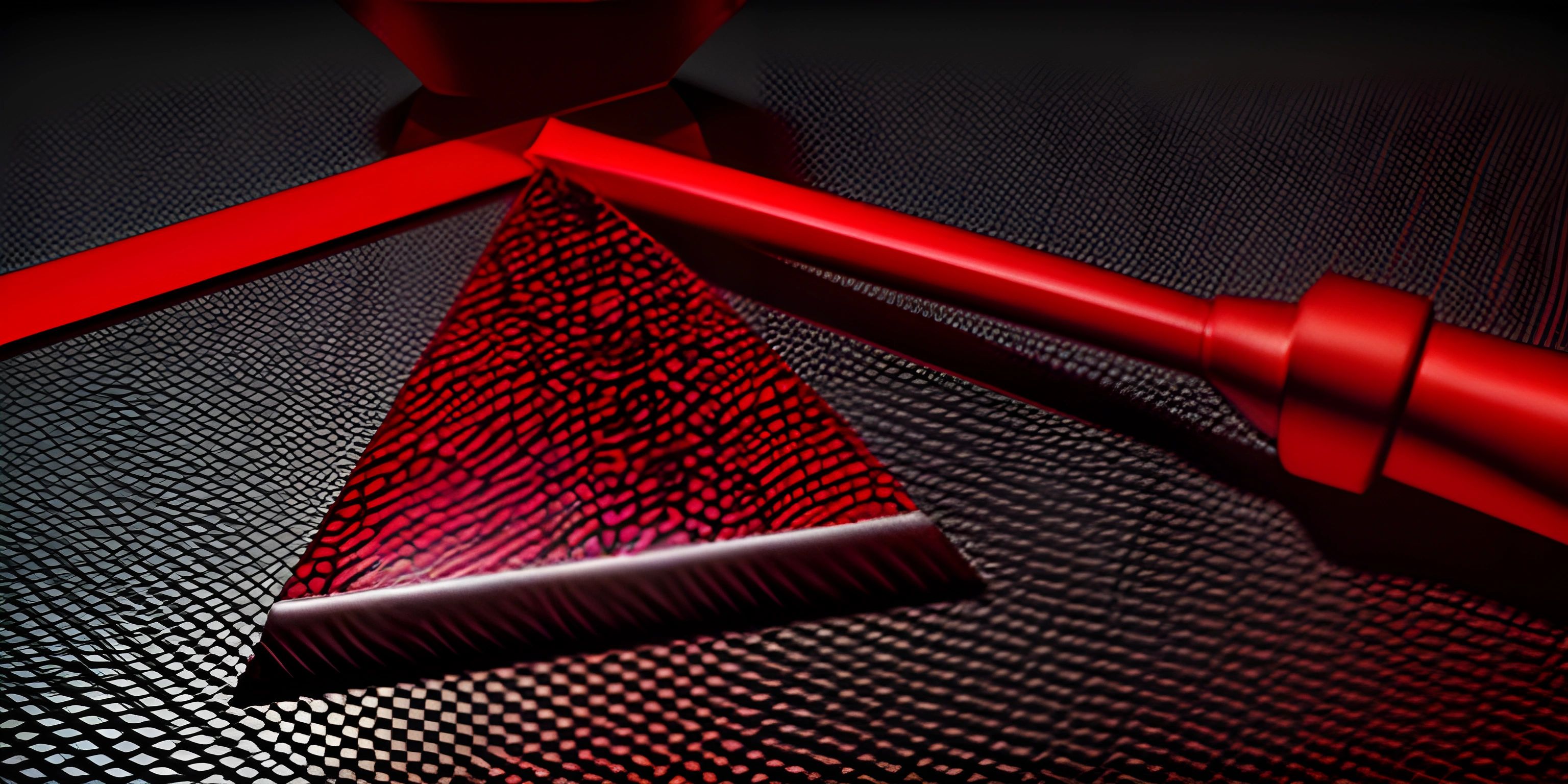
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Every programmer encounters error messages at some point. These messages can be confusing at first, but they're actually here to help us understand and fix the issues in our code. In this article, we'll cover some common error messages in Python, explain their causes, and provide solutions to resolve them.
SyntaxError: invalid syntax
A SyntaxError
occurs when Python encounters an issue in the structure of your code. This might be a missing or misplaced punctuation mark, a missing keyword, or incorrect indentation. Here's an example:
print("Hello, world!"
In this case, we forgot to close the parentheses, causing a SyntaxError
. To fix the error, add a closing parenthesis:
print("Hello, world!")
Always ensure that your code is properly formatted and follows Python's syntax rules.
NameError: name 'variable_name' is not defined
A NameError
occurs when you try to use a variable or function that hasn't been defined yet. Make sure to define the variable or import the required module before using it. For example:
print(name)
This code will result in a NameError
because name
hasn't been defined yet. To fix the error, define the variable before using it:
name = "Alice" print(name)
TypeError: unsupported operand type(s) for +: 'int' and 'str'
A TypeError
occurs when you try to perform an operation on incompatible data types. In this example, we're trying to add an integer and a string, which Python doesn't support:
result = 42 + "hello"
To fix the error, make sure the data types are compatible. You can convert the integer to a string or vice versa, depending on your needs:
result = str(42) + "hello"
AttributeError: 'module' object has no attribute 'function_name'
An AttributeError
occurs when you try to access an attribute or method that doesn't exist in the given object. This often happens when you import a module and try to call a function that isn't part of the module. For example:
import math result = math.sqaure(5) # Typo: should be square
To fix the error, make sure you're calling the correct method or attribute name:
result = math.sqrt(5) # Correct
ImportError: No module named 'module_name'
An ImportError
occurs when Python can't find the module you're trying to import. This might be because the module isn't installed, you misspelled the module name, or it's not in your system's PYTHONPATH
. For example:
import numpyy
To fix the error, make sure you've installed the required module and are using the correct name:
import numpy
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is a SyntaxError in Python?
A SyntaxError occurs when Python encounters an issue in the structure of your code, such as a missing or misplaced punctuation mark, a missing keyword, or incorrect indentation.
How can I fix a NameError in Python?
To fix a NameError, ensure that the variable or function you're trying to use has been defined or imported before using it. If it's a misspelled name, correct the spelling.
What causes a TypeError in Python?
A TypeError occurs when you try to perform an operation on incompatible data types, such as adding an integer and a string. To fix the error, make sure the data types are compatible, either by converting one data type to another or by using an appropriate operation for the given data types.
What is an AttributeError in Python?
An AttributeError occurs when you try to access an attribute or method that doesn't exist in the given object. To fix the error, make sure you're calling the correct method or attribute name.
Why might I encounter an ImportError in Python?
An ImportError occurs when Python can't find the module you're trying to import. This might be because the module isn't installed, you misspelled the module name, or it's not in your system's PYTHONPATH. To fix the error, ensure you've installed the required module and are using the correct name.