Java Basics
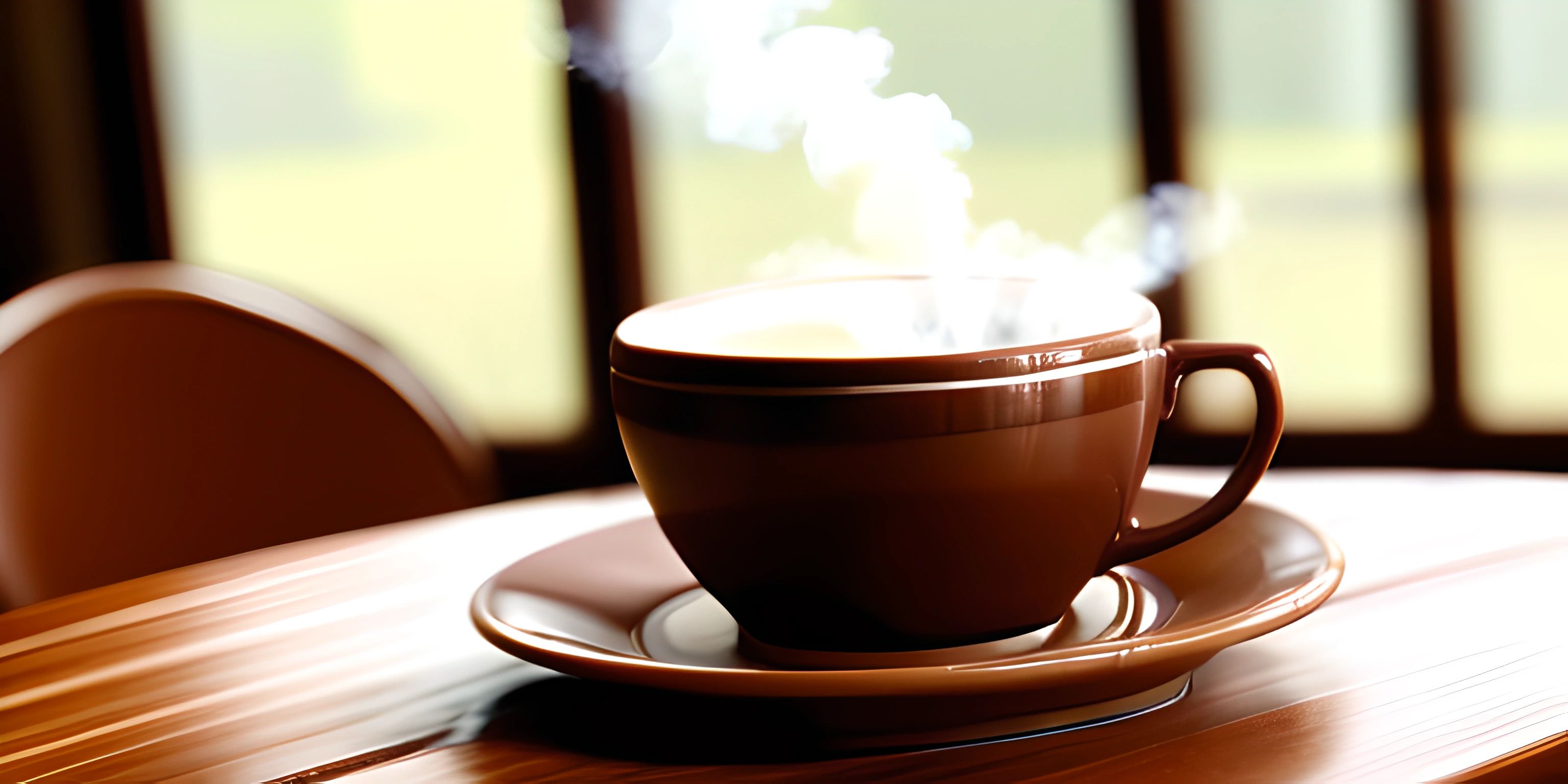
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java is a versatile and widely-used programming language that has withstood the test of time. If you're getting started with Java, it's essential to grasp some of the key concepts that will become the building blocks of your programming journey. Let's dive into the exciting world of Java!
Java Syntax
Java has a unique way of structuring its code, known as its syntax. Here are the main parts of Java syntax that you'll encounter when writing programs:
Variables
In Java, variables are used to store and manipulate data. To create a variable, you'll need to declare its type, followed by its name. For example:
int myNumber;
This line declares a variable named myNumber
of type int
(integer). You can also assign a value to the variable when declaring it:
int myNumber = 42;
Java supports various data types, including int
, double
, char
, boolean
, and String
. Each type is used for different purposes, depending on the data you're working with.
Methods
Methods are reusable blocks of code that perform a specific task. They can be called by their name whenever you need to use them. In Java, every program starts with a main method, which acts as the entry point for the program:
public static void main(String[] args) { // Your code goes here }
You can create your own methods in Java by defining their signature, which includes the method's name, return type, and any parameters it takes. Here's an example of a simple method in Java:
public static int addNumbers(int a, int b) { return a + b; }
This method takes two integer parameters, a
and b
, and returns their sum as an integer.
Control Structures
Java provides various control structures that allow you to control the flow of your program. Some common control structures include if
, else
, for
, while
, and switch
. These structures help you create conditional statements and loops in your code.
For example, here's a simple if-else
statement in Java:
int temperature = 75; if (temperature > 80) { System.out.println("It's hot outside!"); } else { System.out.println("It's not too hot today."); }
This code checks if the temperature
variable is greater than 80. If it is, it prints "It's hot outside!", otherwise it prints "It's not too hot today."
Putting It All Together
Now that you understand the basics of Java syntax, let's see an example of a simple Java program that uses variables, methods, and control structures:
public class HelloWorld { public static void main(String[] args) { int a = 10; int b = 20; int sum = addNumbers(a, b); if (sum > 0) { System.out.println("The sum is positive: " + sum); } else { System.out.println("The sum is not positive: " + sum); } } public static int addNumbers(int a, int b) { return a + b; } }
This program defines a main
method, declares variables a
, b
, and sum
, calls the addNumbers
method, and uses an if-else
statement to print the result.
With these basics under your belt, you're well on your way to exploring the vast world of Java programming. Keep practicing, and you'll soon be creating complex programs in no time!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are the main components of a basic Java program?
A basic Java program consists of the following components:
- Class: A blueprint that defines the structure and behavior of objects. Every Java program must have at least one class.
- Variables: Containers that store data values. In Java, you need to specify the type of data a variable can hold.
- Methods: Blocks of code that perform specific tasks. Methods can be called to execute their code.
- Control Structures: Statements that control the flow of execution in a program, such as loops and conditional statements.
How do I declare and initialize variables in Java?
To declare a variable in Java, you need to specify its data type, followed by the variable name. To initialize the variable, you can assign it a value using the assignment operator =
. Here's an example:
int myNumber; // Declaration myNumber = 42; // Initialization String myString = "Hello, World!"; // Declaration & Initialization
How do I create a simple method in Java?
To create a method in Java, you need to define its return type, method name, and any parameters it might accept. The method code goes inside a pair of curly braces {}
. Here's an example of a simple method that adds two numbers:
public int addNumbers(int num1, int num2) { int result = num1 + num2; return result; }
What are some common control structures in Java?
Some common control structures in Java include:
- If-else statement: Executes a block of code if a certain condition is met, or another block of code if the condition is not met.
- For loop: Repeats a block of code a specific number of times, based on a loop counter.
- While loop: Repeats a block of code as long as a certain condition remains true.
- Switch statement: Executes a block of code based on the value of a specific variable or expression.
How can I create a simple Java program that prints "Hello, World!"?
To create a simple Java program that prints "Hello, World!", follow these steps:
- Create a new Java class with a
main
method. - Inside the
main
method, use theSystem.out.println()
method to print "Hello, World!". Here's an example of the complete program:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }