Data Structures Basics
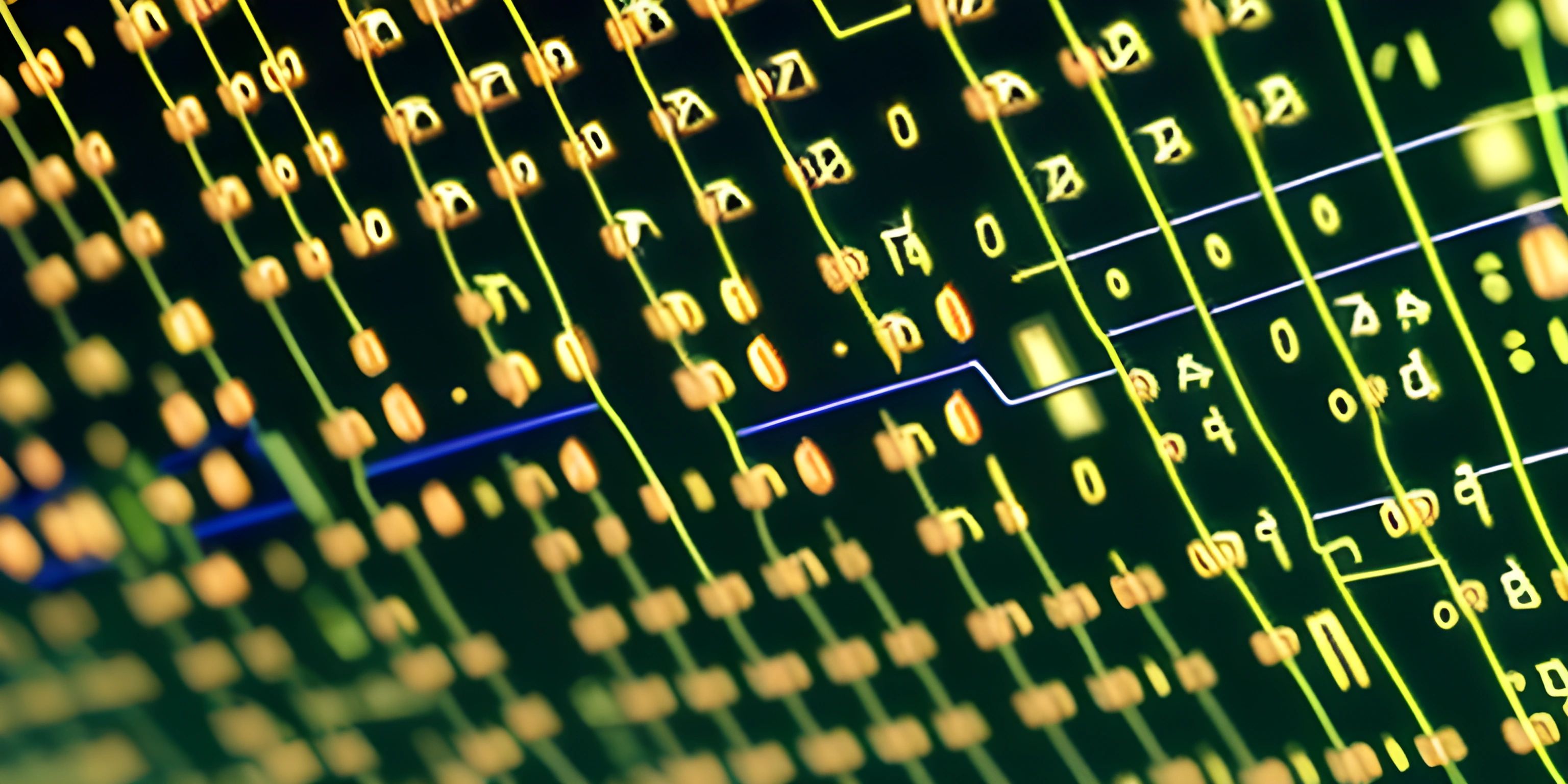
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of programming, organizing and managing data is crucial. Data structures are the backbone of this organization, and understanding them is essential for any aspiring programmer. So buckle up, as we embark on a journey to explore the basics of data structures and why they are so important.
What Are Data Structures?
Simply put, data structures are ways to store and organize data. They determine how data is accessed, manipulated, and stored within a program. There are many types of data structures, each with its strengths and weaknesses. Some of the most common data structures include arrays, lists, stacks, queues, and trees.
A well-chosen data structure can make your code more efficient, easier to read, and easier to maintain. On the flip side, a poorly chosen data structure can lead to slow, hard-to-understand code that's prone to errors. That's why it's crucial to understand the fundamentals, so you can make informed choices when designing your programs.
Arrays and Lists
Let's start with two of the most basic data structures: arrays and lists. These are essentially containers that store data in a linear fashion. Think of them like a row of lockers, each holding a single item.
Arrays are fixed in size and store data of the same type (e.g., integers, strings, or even other data structures). Lists, on the other hand, can grow or shrink in size and can store elements of different types. While arrays are faster and more memory-efficient, lists offer greater flexibility.
Here's a simple example of an array in Python:
my_array = [1, 2, 3, 4, 5]
And here's a list:
my_list = [1, "apple", 3.14]
Stacks and Queues
Stacks and queues are two more data structures that are similar to arrays and lists but have specific rules for adding and removing data. Imagine a stack of plates or a queue of people waiting in line.
A stack follows the LIFO (Last In, First Out) principle, meaning that the last item added to the stack is the first one to be removed. A queue, on the other hand, follows the FIFO (First In, First Out) principle, so the first item added is the first one to be removed.
Trees
Moving away from linear structures, we have trees—hierarchical data structures that consist of nodes connected by edges. Trees are particularly useful for organizing data that has a natural hierarchy, like the files and folders on your computer.
The topmost node, known as the root, is the starting point of the tree. Each node can have one or more child nodes, which can have their children and so on. The node without any children is called a leaf. There are various types of trees, such as binary trees and balanced trees, each with their specific use cases.
Why Are Data Structures Important?
Data structures are the foundation of any program, allowing you to store, access, and manipulate data efficiently. Choosing the right data structure for your specific problem can lead to more elegant and efficient code, making it easier to understand and maintain. As a programmer, it's essential to have a firm grasp of the different data structures and their benefits and trade-offs to make the best decisions for your projects.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: AI Test Playground (psst, it's free!).
FAQ
What are data structures?
Data structures are ways to store and organize data within a program. They determine how data is accessed, manipulated, and stored. Some common data structures include arrays, lists, stacks, queues, and trees.
Why are data structures important in programming?
Data structures are essential in programming because they allow you to store, access, and manipulate data efficiently. Choosing the right data structure for your specific problem can lead to more elegant and efficient code, making it easier to understand and maintain.
What are some basic data structures?
Some of the most basic data structures include arrays, lists, stacks, queues, and trees. Arrays and lists are linear data structures that store items in a sequential fashion, while stacks and queues are linear data structures with specific rules for adding and removing items. Trees are hierarchical data structures that organize data in a tree-like structure with nodes connected by edges.