Creative Coding with Python Turtle Library
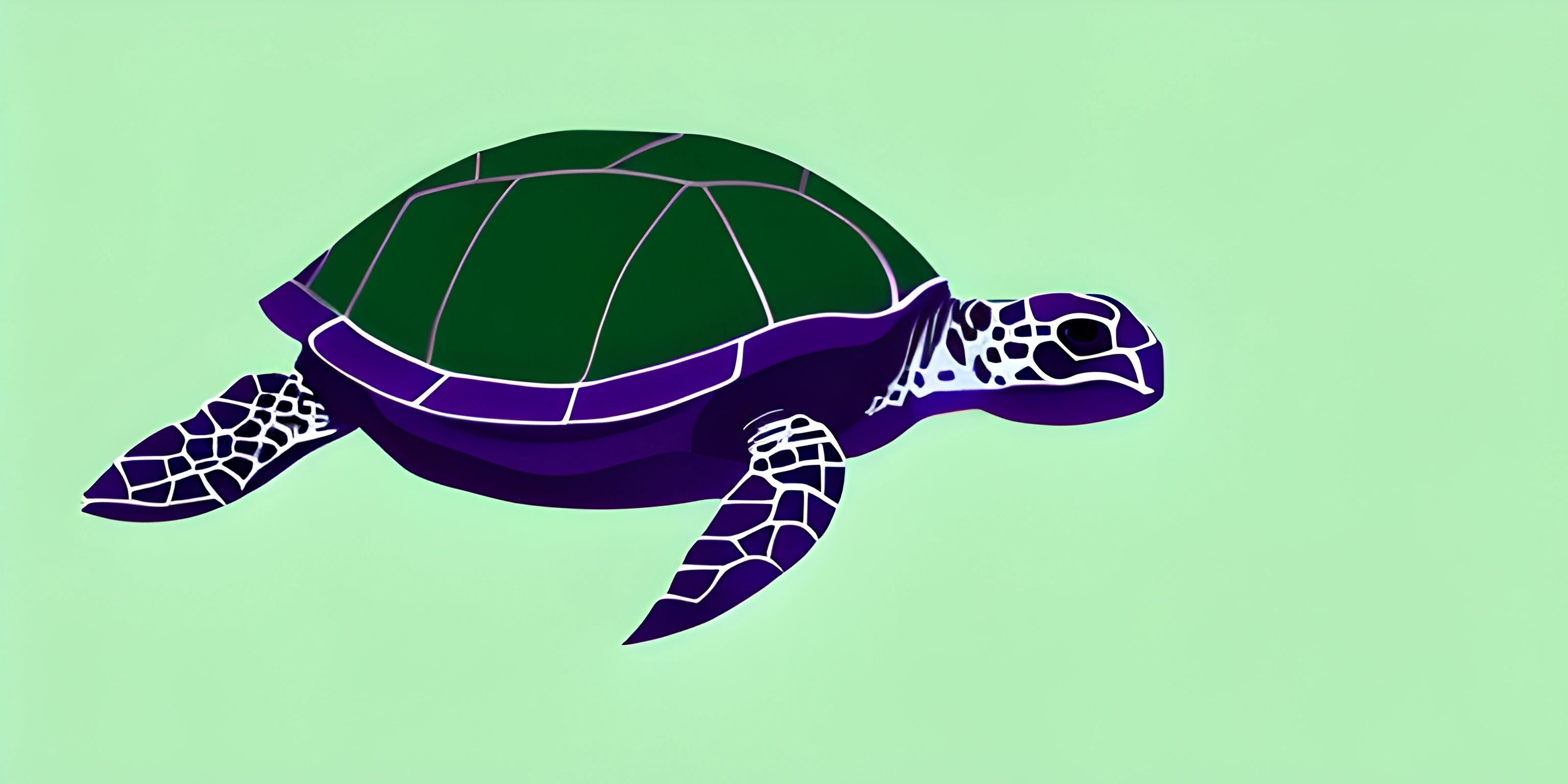
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Drawing art with code can be both entertaining and educational. The Python Turtle library is a fantastic tool for creating graphics and exploring creative coding. It's time to unleash your inner Picasso!
Python Turtle Library
The Turtle library is a collection of functions that allow you to control a virtual "turtle" on your screen. The turtle is a pen that can move, turn, and draw lines or shapes. It's inspired by the Logo programming language, which was used to teach programming concepts in the 1960s.
To get started, you'll need Python installed on your machine. If you don't have Python yet, head over to the Python website to download and install the latest version.
Once you have Python installed, you can access the Turtle library, as it comes bundled with Python. No need to install anything else!
Your First Turtle Drawing
Let's begin by drawing a simple square. First, create a new Python file named square.py
and add the following code:
import turtle # Create a turtle object pen = turtle.Turtle() # Draw a square for i in range(4): pen.forward(100) pen.right(90) # Close the turtle window when done turtle.done()
Save the file and run it using your Python interpreter. You should see a window pop up with a square drawn inside. Congratulations, you've just created your first Turtle drawing!
Let's break down the code:
- We start by importing the Turtle library.
- We create a new Turtle object named
pen
. This is our virtual turtle that will draw on the screen. - We use a for loop to repeat the drawing process four times, one for each side of the square.
- Inside the loop, we tell the turtle to move forward by 100 units and then turn right 90 degrees.
- After the loop, we call
turtle.done()
to signal that we're finished drawing.
Exploring Turtle Commands
The Turtle library has many commands to control the turtle's movement and appearance. Here are some essential ones:
pen.forward(distance)
: Move the turtle forward by the specified distance.pen.backward(distance)
: Move the turtle backward by the specified distance.pen.right(angle)
: Turn the turtle right by the specified angle.pen.left(angle)
: Turn the turtle left by the specified angle.pen.penup()
: Lift the pen off the screen, so it doesn't draw while moving.pen.pendown()
: Lower the pen onto the screen so it can draw.pen.color("color_name")
: Change the pen's color. You can use color names like "red", "blue", "green", etc.pen.width(width)
: Set the width of the pen's line.
Try experimenting with these commands to create different shapes and patterns.
Creating a Colorful Spiral
Time to get more creative! Let's use the Turtle library to draw a colorful spiral pattern. Create a new Python file named spiral.py
and add the following code:
import turtle # Create a turtle object pen = turtle.Turtle() # Set the initial pen color pen.color("red") # Draw a spiral for i in range(100): pen.forward(i * 2) pen.right(144) # Change the pen color if i % 4 == 0: pen.color("blue") elif i % 4 == 1: pen.color("green") elif i % 4 == 2: pen.color("yellow") else: pen.color("red") # Close the turtle window when done turtle.done()
Save the file and run it. You should see a mesmerizing spiral pattern with alternating colors. Feel free to modify the code and experiment with different angles, colors, and shapes.
Now that you've got the hang of creative coding with the Python Turtle library, the possibilities are endless. Keep exploring, and remember to have fun!