Getting Started With Python's Turtle Library
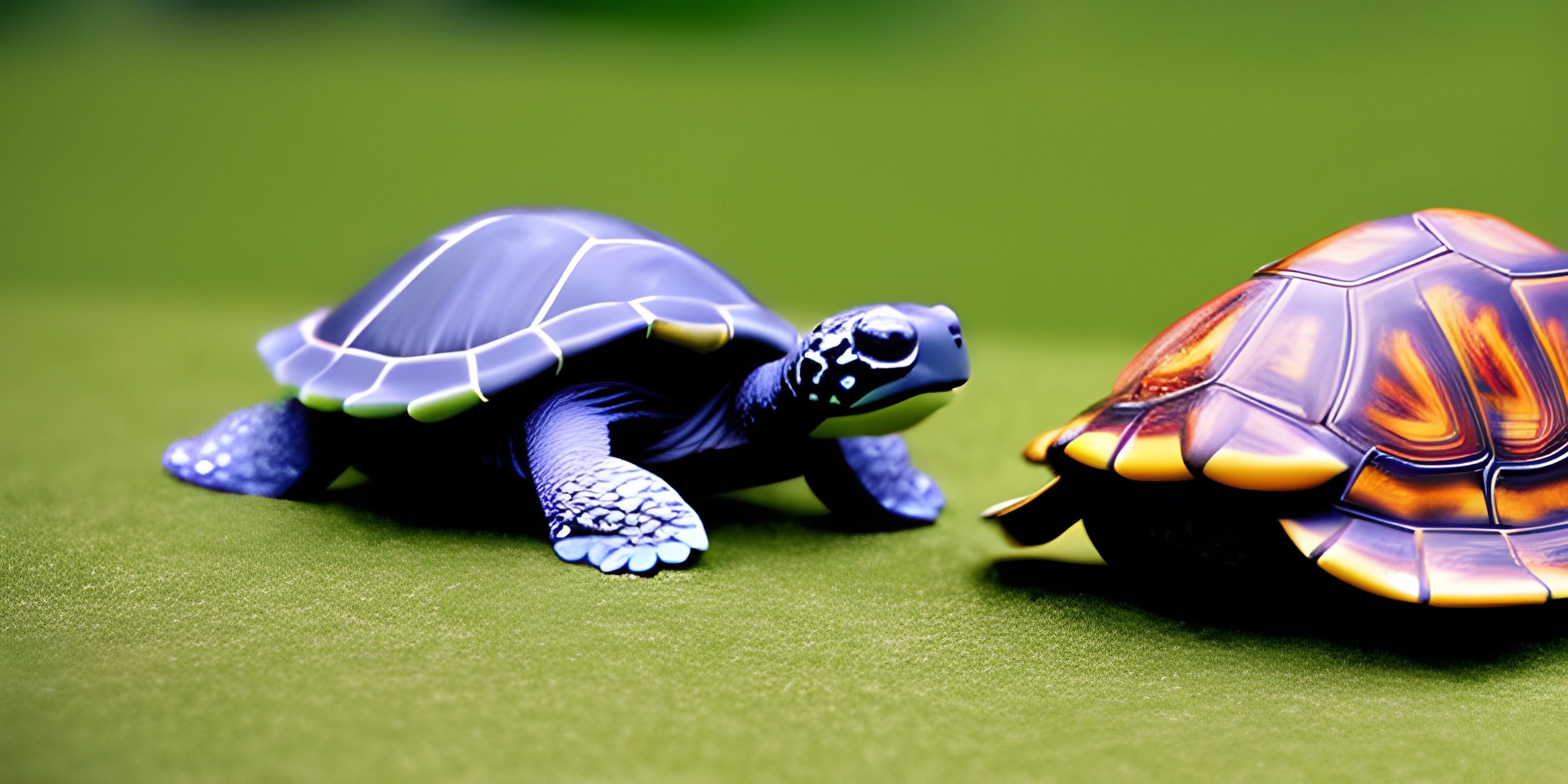
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Let's dive into an exciting facet of Python - the Turtle library. This library lets us control a tiny creature called a 'turtle' that leaves a trail as it moves. With this turtle, we can create interesting shapes, designs, and even animations. Think of it as your personal, programmable doodle machine!
Meet the Turtle
The turtle is a cursor in a graphical window, controlled by a set of commands. In Python, we can import the turtle library using import turtle
, which gives our code access to all the turtle methods.
import turtle
This line of code is like unlocking a secret door that leads to a wizard's workshop, filled with magical commands to control our turtle.
Drawing with the Turtle
Once the turtle is imported, we can use it to start drawing. Let's make our turtle draw a square.
import turtle turtle.forward(100) # Moves the turtle forward by 100 units turtle.right(90) # Rotates the turtle by 90 degrees to the right turtle.forward(100) turtle.right(90) turtle.forward(100) turtle.right(90) turtle.forward(100) turtle.done() # Ends the turtle drawing
This piece of code will draw a square. The turtle starts at the center of the window, moves forward by 100 units, rotates 90 degrees to the right, and repeats these two steps until it has drawn a complete square. It's like we're whispering instructions to the turtle, and it dutifully follows them, leaving a trail of ink behind.
The Power of Loops
Repeating commands can be tiring. Luckily, Python offers a concept known as loops to repeat a block of code multiple times. Let's revisit our previous square-drawing example but with loops:
import turtle for _ in range(4): turtle.forward(100) turtle.right(90) turtle.done()
Much cleaner, isn't it? The turtle still draws the square, but this time we're using a for-loop, making the code more efficient and easier to read.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Upgrading our Calculator (psst, it's free!).
FAQ
What is the Python Turtle library?
The Python Turtle library is a collection of functions that allow you to create images using a panel and pen. It's a great library to learn about graphics, as it provides intuitive commands to control a cursor called a 'turtle.'
How do I draw a square using the Turtle library?
To draw a square using the Turtle library, you would use the forward
command to draw a line and the right
or left
command to turn the turtle. You would repeat this process four times to complete the square.
Can I create complex shapes using the Turtle library?
Absolutely! You can create a wide variety of shapes and designs using the Turtle library. It provides numerous commands to move the turtle around the screen, change the line color, fill shapes, and more. With a bit of practice and creativity, you can create stunning graphics using the Turtle library.
What is the use of `turtle.done()` function?
The turtle.done()
function is used at the end of the program when you're done drawing. It terminates the turtle graphics window and should always be included at the end of your turtle program.
How can I make my code more efficient when using the Turtle library?
You can use loops in Python to repeat a block of code multiple times, making your code more efficient and easier to read. For instance, if you're drawing a square, you can use a for-loop to repeat the drawing and turning commands four times instead of writing them out individually.