Introduction to Algorithms
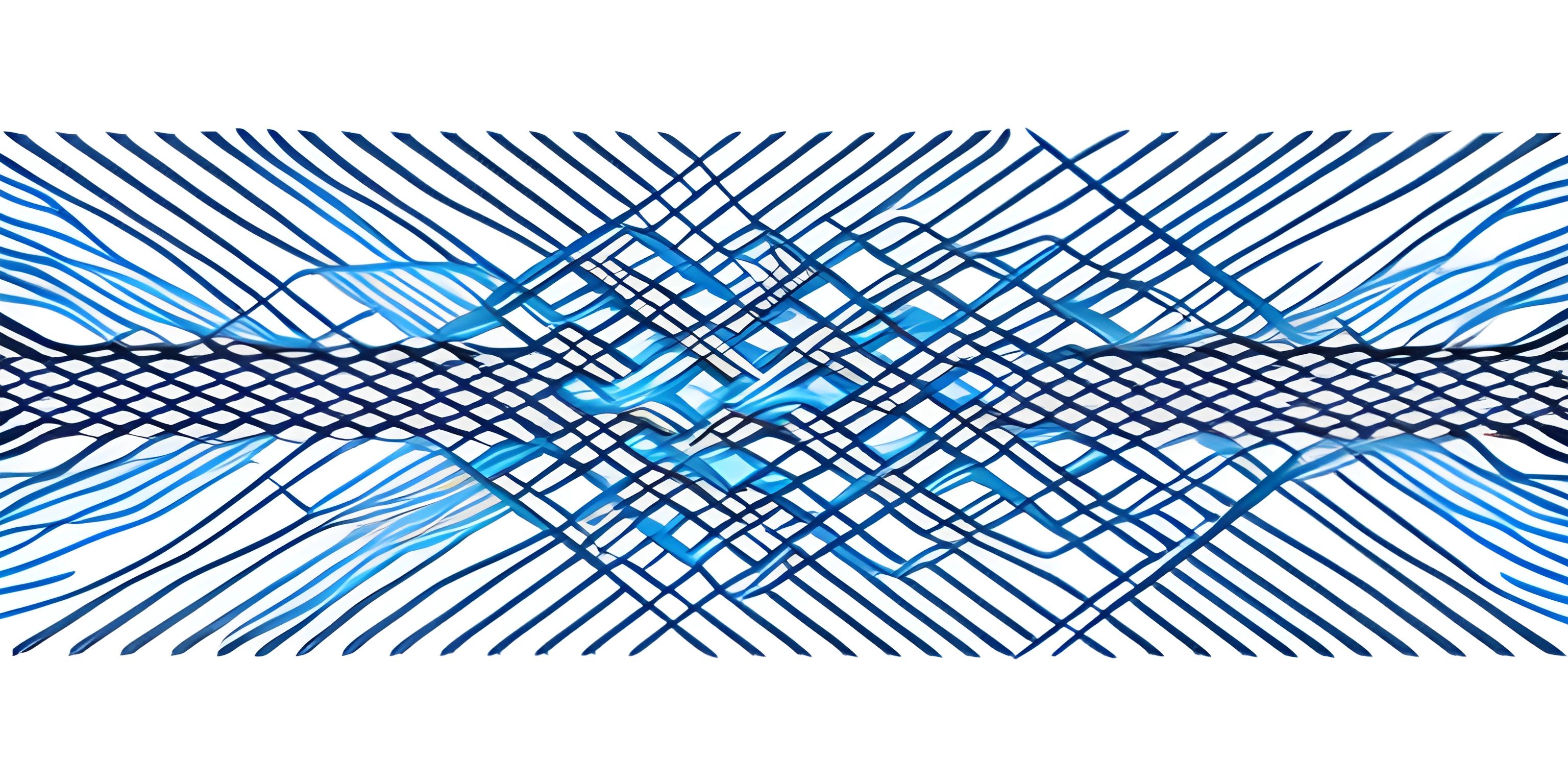
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Algorithms are the beating heart of computer science. They're the step-by-step instructions that tell computers how to solve a problem or perform a task. In this article, we'll explore the essence of algorithms and understand their importance in computer science. Let's dive in!
What is an Algorithm?
An algorithm is a well-defined sequence of steps, often expressed in pseudocode, that describes how to solve a specific problem or perform a particular task. It's like a recipe for your favorite dish, which contains a list of ingredients and step-by-step instructions on how to prepare it.
In computer science, algorithms are designed to be executed by computers, using a programming language. The language used to implement an algorithm can vary, but the logic of the algorithm remains the same.
Here's a simple example of an algorithm to find the largest number in a list of numbers:
- Start with the first number as the largest number found so far.
- For each remaining number in the list: a. Compare the current number with the largest number found so far. b. If the current number is larger, update the largest number.
- After checking all the numbers, the largest number found is the largest number in the list.
Why are Algorithms Important?
Algorithms play a pivotal role in computer science for several reasons:
Efficiency
An efficient algorithm can significantly reduce the time and resources required to solve a problem. As the size of the input data grows, the performance difference between a good algorithm and a poor one can become dramatic. Efficient algorithms can help us manage and process large-scale data, such as the ones generated by social media platforms or scientific simulations.
Reusability
Algorithms are often designed to be reusable, allowing them to be applied to a variety of problems. For example, sorting algorithms can be used to arrange data in a specific order, regardless of the type of data being sorted. By reusing algorithms, developers can save time and effort, focusing on other aspects of their software.
Foundation for Advanced Concepts
Algorithms lay the groundwork for more advanced computer science concepts, such as data structures, artificial intelligence, and machine learning. A solid understanding of algorithms allows developers to build efficient, reliable, and scalable software systems.
Types of Algorithms
There are several types of algorithms, each designed to solve a particular category of problems. Some common types include:
- Sorting Algorithms - Arrange data in a specific order (e.g., ascending or descending).
- Searching Algorithms - Find a specific element within a data structure.
- Graph Algorithms - Solve problems related to graph data structures, such as finding the shortest path between two nodes.
- Dynamic Programming - Solve problems by breaking them into smaller, overlapping subproblems and solving each subproblem only once.
The Art of Crafting Algorithms
Creating an algorithm involves a combination of creativity, logic, and problem-solving skills. It's an iterative process that includes:
- Understanding the problem and its requirements.
- Designing a step-by-step solution using pseudocode or flowcharts.
- Implementing the algorithm using a programming language.
- Testing and refining the algorithm to ensure it meets the desired goals.
In conclusion, algorithms are a fundamental aspect of computer science. They provide the foundation for efficient, reusable, and scalable software systems. By understanding the importance of algorithms and their various types, you'll be better equipped to tackle complex programming challenges and create innovative solutions.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is an algorithm?
An algorithm is a step-by-step procedure or set of instructions to solve a specific problem or accomplish a certain task. In the context of computer science, algorithms are the foundation of programs that run on computers, helping them process data and perform various operations.
Why are algorithms important in computer science?
Algorithms are crucial in computer science because they provide the logic behind every computer program. They enable computers to perform complex tasks, solve problems, and make decisions. Understanding and designing efficient algorithms is key to developing faster and more effective software applications and systems.
What are some common types of algorithms?
There are numerous types of algorithms, some of which include:
- Sorting algorithms (e.g., Bubble Sort, Quick Sort, Merge Sort)
- Searching algorithms (e.g., Linear Search, Binary Search)
- Graph algorithms (e.g., Breadth-First Search, Depth-First Search, Dijkstra's Shortest Path)
- Dynamic programming algorithms (e.g., Fibonacci series, Knapsack problem)
- Divide and conquer algorithms (e.g., Fast Fourier Transform, Strassen's Matrix Multiplication)
- Greedy algorithms (e.g., Kruskal's Minimum Spanning Tree, Huffman Coding)
How can I evaluate the performance of an algorithm?
The performance of an algorithm is typically evaluated based on its time complexity and space complexity. Time complexity refers to the amount of time an algorithm takes to run as a function of its input size, while space complexity refers to the amount of memory an algorithm uses during its execution. Common notations used to describe complexities include Big O (O), Big Omega (Ω), and Big Theta (Θ) notations.
Can you provide a simple example of an algorithm?
Sure! Let's look at a basic algorithm to find the largest number in a list:
def find_largest_number(numbers): largest = numbers[0] for number in numbers: if number > largest: largest = number return largest numbers = [34, 12, 67, 89, 21, 56] print(find_largest_number(numbers))
In this example, the algorithm iterates through the list of numbers, comparing each element to the current largest number. If an element is larger, it replaces the current largest number. Once the iteration is complete, the largest number is returned.