Discord.js Command Handling
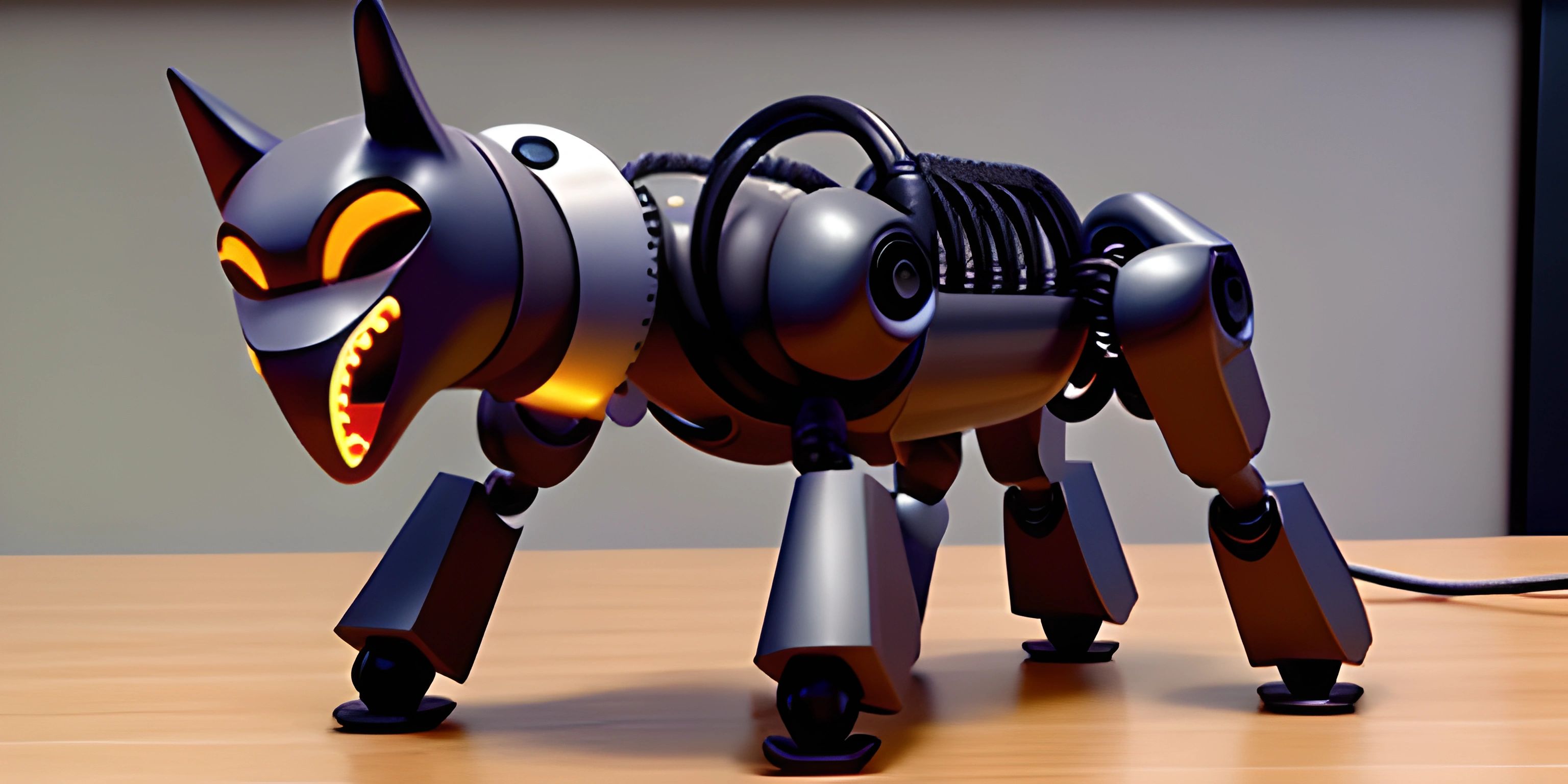
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Creating a Discord bot can be a fun and rewarding experience, but as your bot grows, managing its commands can become cumbersome. Fear not, for command handling comes to the rescue! In this adventure, we'll explore the magical land of command handlers, making our Discord.js bot more organized and easier to manage. So, buckle up and let's dive in!
What is Command Handling?
Imagine your bot as a restaurant, and commands are the dishes it serves. As your menu expands, it becomes harder to keep track of every dish and its ingredients. Command handling is like having a well-organized kitchen, where each dish has its own recipe card, making it easier to find, modify, or remove dishes.
In Discord.js bot terms, command handling means organizing your commands into separate files and dynamically loading them when needed. This makes your bot more modular, scalable, and maintainable.
Setting Up Your Project
First, ensure you have Node.js installed, and then create a new project folder. Initialize your project using npm init
, and install the discord.js
library:
npm install discord.js
Next, create an index.js
file, which will be the entry point of your bot, and set up a basic Discord.js bot with your token:
const Discord = require("discord.js"); const client = new Discord.Client(); client.on("ready", () => { console.log(`Logged in as ${client.user.tag}!`); }); client.login("your-token-here"); // Replace with your bot's token
Now, let's venture into the realm of command handling!
Creating the Command Handler
-
Organize your commands: Create a new folder called
commands
in your project directory. Each command will be a separate file inside this folder, named after the command (e.g.,ping.js
for theping
command). -
Define command properties: In each command file, we'll define a
name
and anexecute
function. For example, inping.js
:
module.exports = { name: "ping", execute(message, args) { message.channel.send("Pong!"); }, };
- Load your commands: Back in
index.js
, create acommands
collection to store your commands:
client.commands = new Discord.Collection();
Then, read the command files from the commands
folder and load them into the collection:
const fs = require("fs"); const commandFiles = fs.readdirSync("./commands").filter(file => file.endsWith(".js")); for (const file of commandFiles) { const command = require(`./commands/${file}`); client.commands.set(command.name, command); }
- Handle incoming messages: Modify your bot's
message
event to parse the command and its arguments, and execute the corresponding command:
const prefix = "!"; // Replace with your desired prefix client.on("message", message => { if (!message.content.startsWith(prefix) || message.author.bot) return; const args = message.content.slice(prefix.length).split(/ +/); const commandName = args.shift().toLowerCase(); const command = client.commands.get(commandName); if (!command) return; try { command.execute(message, args); } catch (error) { console.error(error); message.reply("There was an error trying to execute that command!"); } });
And there you have it - your very own command handler for your Discord.js bot! You can now easily add, modify, or remove commands by simply changing the files in the commands
folder. Happy bot building!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is a command handler in Discord.js?
A command handler is a system that helps manage and organize commands for your Discord.js bot. It allows you to separate each command into its own file, making your code more modular and easier to maintain. With a command handler, you can quickly add, remove, or modify commands without having to dig through your bot's entire codebase.
How do I set up a command handler in Discord.js?
To set up a command handler in Discord.js, follow these steps:
-
Create a folder named
commands
in your bot's root directory. This is where you'll store each command's file. -
In each command file, export an object with the properties
name
,description
, andexecute
. Theexecute
function should contain the code to run when the command is called.Example of a command file (ping.js):
module.exports = { name: "ping", description: "Ping the bot to check its response time.", execute(message, args) { message.channel.send("Pong!"); } };
-
In your bot's main file (e.g.,
index.js
), read the command files from thecommands
folder using thefs
module. Store them in a collection. -
In the bot's
message
event, parse the command and its arguments. Look up the command in the collection and execute itsexecute
function if it exists. Here's an example of how your bot's main file (e.g.,index.js
) could look like:const fs = require("fs"); const Discord = require("discord.js"); const client = new Discord.Client(); client.commands = new Discord.Collection(); const commandFiles = fs.readdirSync("./commands").filter(file => file.endsWith(".js")); for (const file of commandFiles) { const command = require(`./commands/${file}`); client.commands.set(command.name, command); } client.on("message", message => { if (!message.content.startsWith(prefix) || message.author.bot) return; const args = message.content.slice(prefix.length).split(/ +/); const commandName = args.shift().toLowerCase(); const command = client.commands.get(commandName) || client.commands.find(cmd => cmd.aliases && cmd.aliases.includes(commandName)); if (!command) return; try { command.execute(message, args); } catch (error) { console.error(error); message.reply("There was an error trying to execute that command!"); } }); client.login("your-token");
How can I add aliases to my commands with a command handler?
To add aliases to your commands, simply include an aliases
property in the command's exported object. The aliases
property should be an array of strings, containing alternative names for the command.
Here's an example of how to add aliases to a command (e.g., ping.js
):
module.exports = { name: "ping", aliases: ["pingbot", "pingme"], description: "Ping the bot to check its response time.", execute(message, args) { message.channel.send("Pong!"); } };
Then, in your bot's main file (e.g., index.js
), update the command look-up to search for aliases as well:
const command = client.commands.get(commandName) || client.commands.find(cmd => cmd.aliases && cmd.aliases.includes(commandName));
Now your command can be triggered using any of its aliases.
How do I handle command arguments with a command handler?
To handle command arguments with a command handler, first parse the arguments from the message content in your bot's main file (e.g., index.js
). Then, pass the arguments as a parameter to the command's execute
function.
Here's an example of parsing command arguments and passing them to the execute
function:
// In your bot's main file (e.g., index.js) client.on("message", message => { if (!message.content.startsWith(prefix) || message.author.bot) return; const args = message.content.slice(prefix.length).split(/ +/); const commandName = args.shift().toLowerCase(); const command = client.commands.get(commandName) || client.commands.find(cmd => cmd.aliases && cmd.aliases.includes(commandName)); if (!command) return; try { command.execute(message, args); } catch (error) { console.error(error); message.reply("There was an error trying to execute that command!"); } });
Now, you can access the command arguments within the execute
function of your command files (e.g., ping.js
):
// In a command file (e.g., ping.js) module.exports = { name: "ping", description: "Ping the bot to check its response time.", execute(message, args) { // Use the args array to access command arguments message.channel.send("Pong!"); } };