Creating a Discord Bot
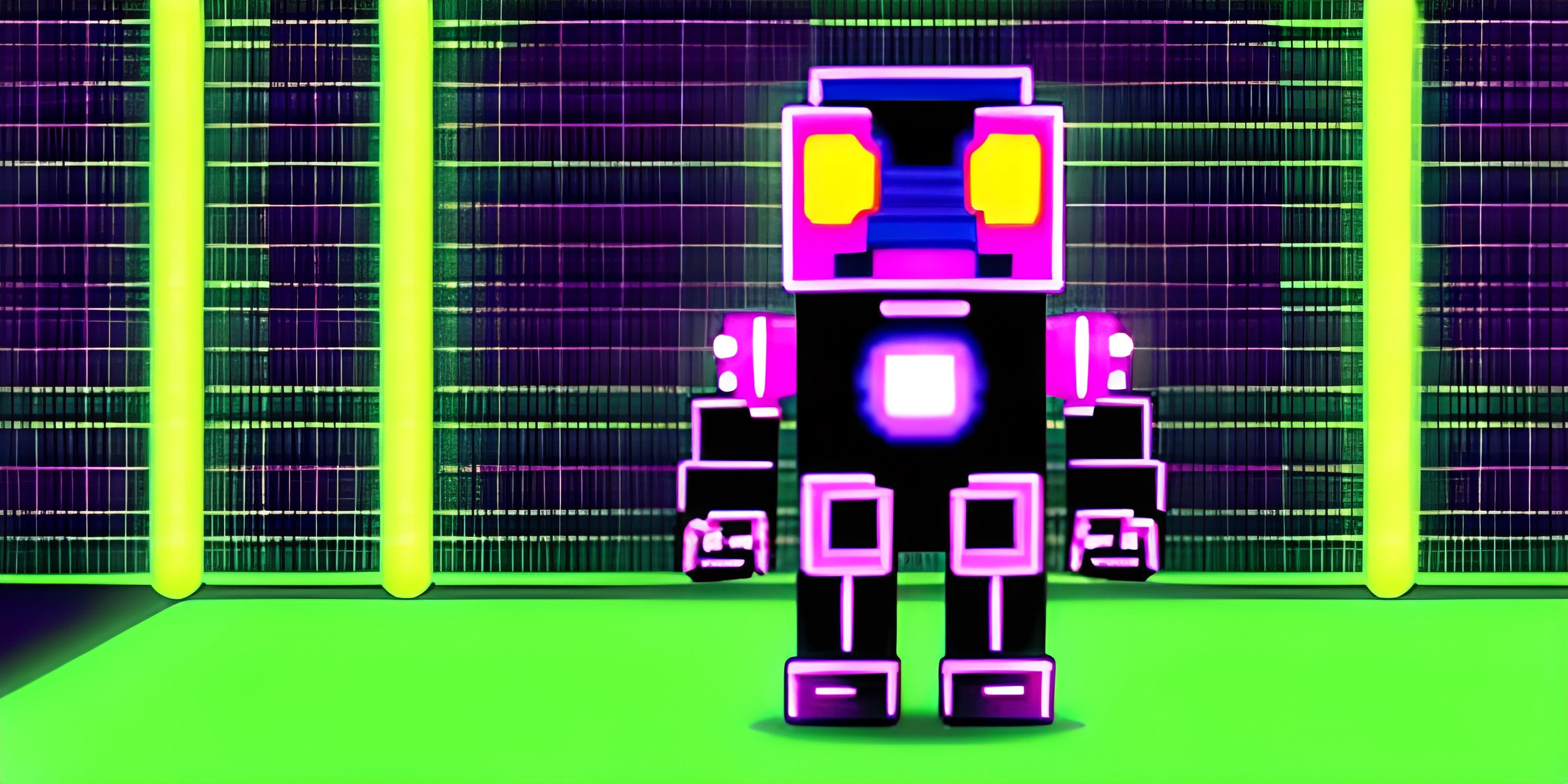
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Discord is a popular messaging platform among gamers, developers, and many other communities. Bots are a great way to enhance and automate various aspects of your Discord server. With the Discord API and some popular libraries, creating a bot is easier than you might think!
Choosing a Language and Library
The first thing to decide is which language you want to use for your bot. Discord provides an API that can be utilized with many programming languages. Some popular choices are Python and JavaScript.
Once you've chosen a language, you'll want to pick a library that simplifies interacting with the Discord API. For Python, discord.py is a popular choice, while discord.js is often used for JavaScript.
Creating a Bot Account
To get started, you'll need to create a bot account on the Discord Developer Portal. Log in with your Discord account and create a new application. Then, navigate to the "Bot" tab and click "Add Bot" to create your bot account.
Take note of your bot's token - this is a unique identifier that allows your code to connect to the bot. Keep it secret, as anyone with the token can control your bot!
Setting Up Your Project
Next, create a new project in your chosen programming language. Make sure to install the appropriate library for Discord bot development:
- For Python:
pip install discord.py
- For JavaScript:
npm install discord.js
Now, you're ready to start coding your bot! Begin by importing the Discord library and creating a new bot client:
Python (discord.py)
import discord from discord.ext import commands bot = commands.Bot(command_prefix="!") @bot.event async def on_ready(): print(f"Logged in as {bot.user.name}") bot.run("your_token_here")
JavaScript (discord.js)
const Discord = require("discord.js"); const client = new Discord.Client(); client.on("ready", () => { console.log(`Logged in as ${client.user.tag}`); }); client.login("your_token_here");
Replace "your_token_here"
with your bot's token from the Discord Developer Portal.
Inviting Your Bot to a Server
Before your bot can respond to commands, you'll need to invite it to your Discord server. Go back to the Discord Developer Portal, navigate to the "OAuth2" tab, and select the "bot" scope. Then, choose the permissions your bot requires and generate an invite link.
Visit the link in your browser, select a server, and authorize the bot. Your bot should now appear in the server's member list!
Implementing Basic Commands
With your bot connected to the server, you can start implementing commands for it to respond to. Here's an example of a simple "ping" command:
Python (discord.py)
@bot.command() async def ping(ctx): await ctx.send("Pong!")
JavaScript (discord.js)
client.on("message", (message) => { if (message.content === "!ping") { message.reply("Pong!"); } });
Now, run your bot and type !ping
in your Discord server. Your bot should respond with "Pong!".
Conclusion
You've now taken the first steps towards creating a Discord bot! There's a lot more to learn, such as managing server roles, handling voice channels, and integrating with external APIs. Explore the documentation for your chosen library and the Discord API to discover more possibilities!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What are the prerequisites for creating a Discord bot?
Before you start creating a Discord bot, make sure you have the following:
- Basic knowledge of programming languages such as JavaScript, Python, or Ruby.
- A Discord account with server permissions to add bots.
- Familiarity with Discord and its features.
How do I create a Discord bot using the Discord API?
To create a Discord bot using the Discord API, follow these steps:
- Visit the Discord Developer Portal at https://discord.com/developers/applications
- Log in to your Discord account.
- Click on "New Application" and enter a name for your bot.
- Navigate to the "Bot" tab and click "Add Bot."
- Acquire the bot's token, which will be used for authentication in your code. Now you can use the Discord API with your preferred programming language and a library that supports Discord bot development, such as discord.js (JavaScript), discord.py (Python), or discordrb (Ruby).
What are some popular libraries for creating Discord bots?
Some popular libraries for creating Discord bots include:
- discord.js (JavaScript): https://discord.js.org
- discord.py (Python): https://discordpy.readthedocs.io
- discordrb (Ruby): https://github.com/shardlab/discordrb These libraries provide a simple and intuitive way to interact with the Discord API, making it easier to create and manage your bot.
How do I add my Discord bot to a server?
To add your Discord bot to a server, follow these steps:
- Go to the Discord Developer Portal (https://discord.com/developers/applications).
- Select your bot's application.
- Navigate to the "OAuth2" tab.
- In the "Scopes" section, select "bot."
- In the "Bot Permissions" section, choose the permissions your bot requires.
- Copy the generated URL and paste it into a new browser window.
- Log in to your Discord account and select the server you want to add the bot to.
- Click "Authorize" and complete the CAPTCHA to finish adding the bot to your server.
Can I use multiple programming languages to create a single Discord bot?
While it is technically possible to use multiple programming languages for a single Discord bot, it is generally not recommended. Mixing languages can lead to increased complexity and maintenance challenges. Instead, choose a single programming language and library that best suits your needs and stick with it throughout the development process.