A Comprehensive Guide to Creating Views in Django
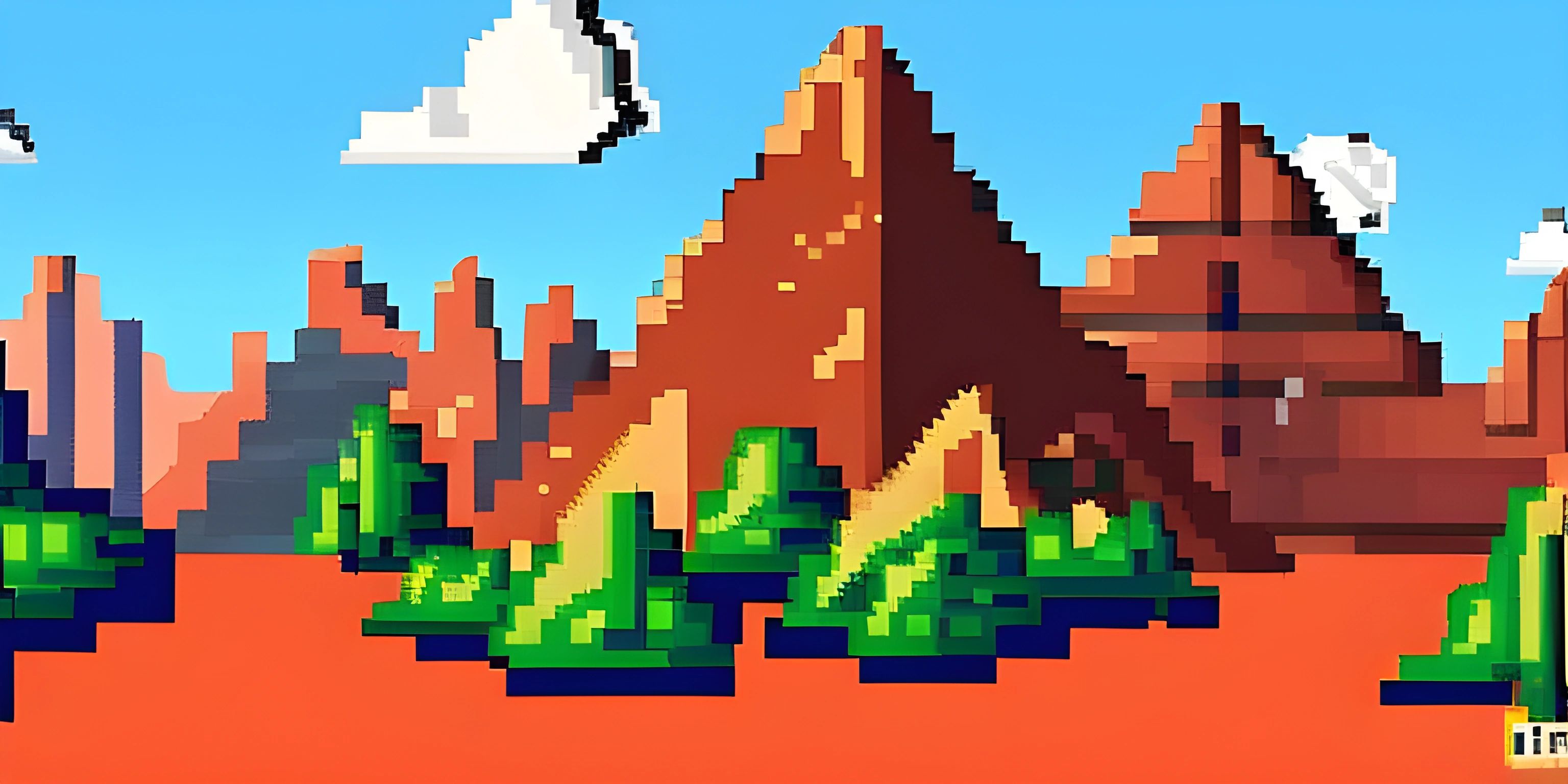
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to building web applications with Django, views are the unsung heroes that make everything tick. Think of views as the directors in a movie production. They decide how the script (your data) is presented to the audience (the users). Without views, your app would just be a collection of static files—boring!
In this guide, we'll cover the basics of creating views in Django, explore the different types of views, and look at some advanced techniques to make your views even more powerful. Let's get started!
What is a View?
A view in Django is essentially a Python function (or class-based view) that takes a web request and returns a web response. It's like a waiter in a restaurant who takes your order and brings you your food. Here's a simple example of a view function:
# views.py from django.http import HttpResponse def hello_world(request): return HttpResponse("Hello, world!")
In this example, the hello_world
function takes a request
object and returns an HttpResponse
object with the text "Hello, world!".
Mapping Views to URLs
Once you've created a view, you need to map it to a URL so that users can access it. This is done in the urls.py
file. Here's how you can map the hello_world
view to a URL:
# urls.py from django.urls import path from .views import hello_world urlpatterns = [ path('hello/', hello_world, name='hello_world'), ]
Now, when users visit /hello/
, they'll see the "Hello, world!" message.
Function-Based Views vs. Class-Based Views
Django offers two types of views: function-based views (FBVs) and class-based views (CBVs).
Function-Based Views (FBVs)
FBVs are simple and straightforward. They are just regular Python functions. Here's an example of a more complex FBV:
# views.py from django.shortcuts import render def home(request): context = {'message': "Welcome to the Home Page"} return render(request, 'home.html', context)
This view uses the render
shortcut to return an HTML template with some context data.
Class-Based Views (CBVs)
CBVs provide more structure and modularity, allowing you to reuse code between views. Here's a simple CBV example:
# views.py from django.http import HttpResponse from django.views import View class HelloWorldView(View): def get(self, request): return HttpResponse("Hello, world!")
To map this view to a URL, you would use:
# urls.py from django.urls import path from .views import HelloWorldView urlpatterns = [ path('hello/', HelloWorldView.as_view(), name='hello_world'), ]
Using Templates with Views
Templates allow you to separate your HTML from your Python code, making your application more maintainable. Here's how you can use templates with views:
# views.py from django.shortcuts import render def home(request): context = {'message': "Welcome to the Home Page"} return render(request, 'home.html', context)
In the home.html
template, you can access the message
context variable:
<!-- home.html --> <!DOCTYPE html> <html> <head> <title>Home Page</title> </head> <body> <h1>{{ message }}</h1> </body> </html>
Advanced View Techniques
Handling Forms in Views
Forms are a crucial part of any web application. Django makes it easy to handle forms in views. Here's an example:
# views.py from django.shortcuts import render, redirect from .forms import ContactForm def contact(request): if request.method == "POST": form = ContactForm(request.POST) if form.is_valid(): # Process the form data return redirect('success') else: form = ContactForm() return render(request, 'contact.html', {'form': form})
In the contact.html
template, you can render the form:
<!-- contact.html --> <!DOCTYPE html> <html> <head> <title>Contact Us</title> </head> <body> <h1>Contact Us</h1> <form method="post"> {% csrf_token %} {{ form.as_p }} <button type="submit">Submit</button> </form> </body> </html>
Using Mixins with Class-Based Views
Mixins are a way to add reusable functionality to your class-based views. For example, you can use the LoginRequiredMixin
to restrict access to authenticated users:
# views.py from django.contrib.auth.mixins import LoginRequiredMixin from django.views.generic import TemplateView class DashboardView(LoginRequiredMixin, TemplateView): template_name = 'dashboard.html'
Decorating Views
Decorators can be used to add functionality to your views. For example, you can use the @login_required
decorator to restrict access to authenticated users:
# views.py from django.contrib.auth.decorators import login_required from django.shortcuts import render @login_required def dashboard(request): return render(request, 'dashboard.html')
Conclusion
Views are the backbone of any Django application. Whether you're using function-based views or class-based views, understanding how to create and manage views will help you build robust and scalable web applications. Remember, views are like the directors of your web application—they decide how your data is presented to the users.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Websites (psst, it's free!).
FAQ
What is a view in Django?
A view in Django is a Python function or class-based view that takes a web request and returns a web response. It controls what data is sent to the user and how it's presented.
How do you map a view to a URL in Django?
You map a view to a URL in the urls.py
file using the path
function from django.urls
. For example: path('hello/', hello_world, name='hello_world')
.
What is the difference between function-based views (FBVs) and class-based views (CBVs)?
FBVs are simple Python functions that handle requests and return responses. CBVs provide more structure and modularity, allowing for reusability and better organization of code.
How can you use templates with views in Django?
You can use the render
shortcut in your views to return an HTML template along with context data. The template can then use the context data to render dynamic content.
What are mixins in Django views?
Mixins are reusable classes that add specific functionality to class-based views. They allow you to add features like login requirement, permissions, and more without repeating code.