Django Framework
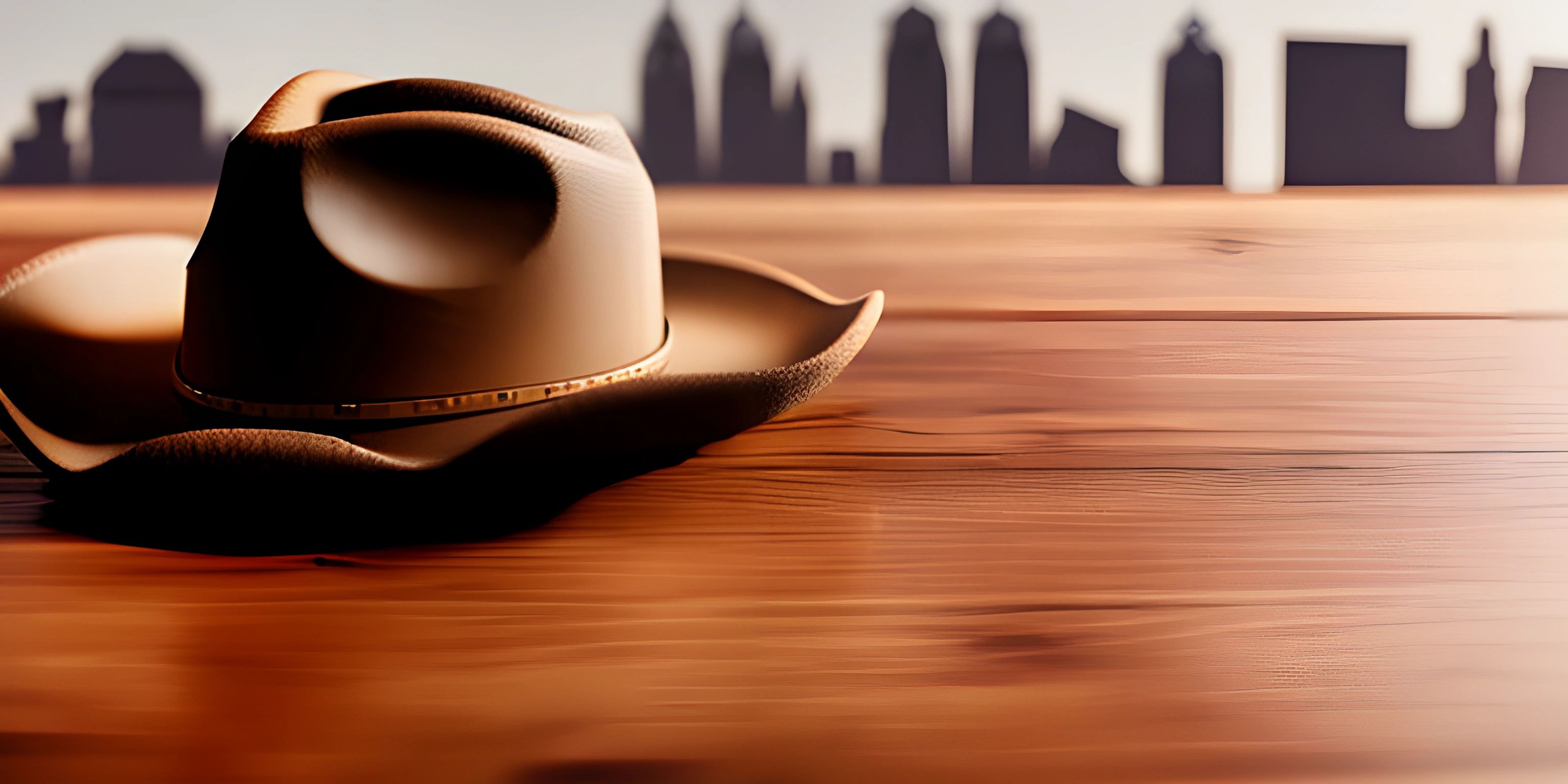
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Developed by experienced developers, Django takes care of much of the hassle of web development, so you can focus on writing your app without needing to reinvent the wheel. It's free and open-source, with a thriving community behind it. It's used by some of the biggest companies, including Instagram, Mozilla, and Disqus, to name a few.
Why Django?
There are plenty of web frameworks out there, so what makes Django stand out? Here are a few reasons:
-
Batteries included: Django comes with a wide array of built-in components and features that help you build your web applications quickly and efficiently. This includes an ORM, an admin interface, authentication, and more.
-
Clean and maintainable code: Django follows the DRY (Don't Repeat Yourself) principle, which helps reduce duplication and makes your code easier to maintain over time. It also promotes the use of best practices and design patterns, such as the MVC (Model-View-Controller) architecture.
-
Scalability: Django has been designed to handle high levels of traffic and is built on a highly-scalable architecture, which makes it suitable for both small and large-scale applications.
-
Security: Security is a top priority for Django. The framework provides built-in protection against common web vulnerabilities, such as cross-site scripting (XSS) and cross-site request forgery (CSRF).
-
Flexible: Django is highly customizable and can be tailored to fit the needs of your specific project. Its modular architecture allows you to plug in third-party apps and libraries easily.
Getting Started with Django
To get started with Django, you first need to have Python installed on your system. If you don't have it yet, you can grab the latest version from the official Python website.
Once you have Python installed, you can install Django using the following command:
pip install django
This will install the latest version of Django on your system. To verify that it has been installed correctly, run the following command:
django-admin --version
This should display the Django version number. Now you're ready to start building your first Django project!
Creating a Django Project
A Django project is a collection of settings and configurations for a specific web application. To create a new project, run the following command:
django-admin startproject myproject
Replace myproject
with the name you'd like to give your project. This will create a new directory with the same name as your project, containing the necessary files and directories to get started.
Running the Django Development Server
Django comes with a built-in development server that allows you to run your project locally for testing and development purposes. To start the server, navigate to your project directory and run the following command:
python manage.py runserver
By default, the server will run on port 8000. You can access your project by visiting http://127.0.0.1:8000/
in your web browser. You should see a "Welcome to Django" page, indicating that everything is set up correctly.
Building Your First Django App
In Django, individual components of a project are called "apps." An app is a self-contained module that encapsulates a specific functionality of your web application. To create a new app, run the following command inside your project directory:
python manage.py startapp myapp
Replace myapp
with the name you'd like to give your app. This will create a new directory with the same name as your app, containing the necessary files and directories to get started.
From here, you can start building your app by defining models, views, and templates. As you progress, you'll find that Django's built-in features and extensive documentation make it easy to build powerful, feature-rich web applications quickly and efficiently.
Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the Django Framework?
The Django Framework is a high-level Python web framework that enables rapid development and promotes clean, pragmatic design in web applications.
What are the main components of the Django Framework?
The main components of the Django Framework include the Model-View-Template (MVT) architecture, URL routing, forms, authentication, and the admin interface.
How does the Django Framework promote rapid development?
Django promotes rapid development by providing a collection of ready-to-use components and built-in tools, such as the ORM, admin interface, and reusable apps, which allows developers to focus on writing their application logic rather than reinventing the wheel.
What is the Model-View-Template (MVT) architecture in Django?
The Model-View-Template (MVT) architecture is Django's version of the Model-View-Controller (MVC) design pattern. It separates an application into three main components: the Model (data structure), View (how the data is displayed), and Template (how the data is presented).
How does Django handle URL routing?
Django handles URL routing through a URL dispatcher, which maps URLs to views. Developers define URL patterns in their applications using regular expressions or the newer path syntax, allowing for flexible and maintainable URL management.