Elixir Metaprogramming
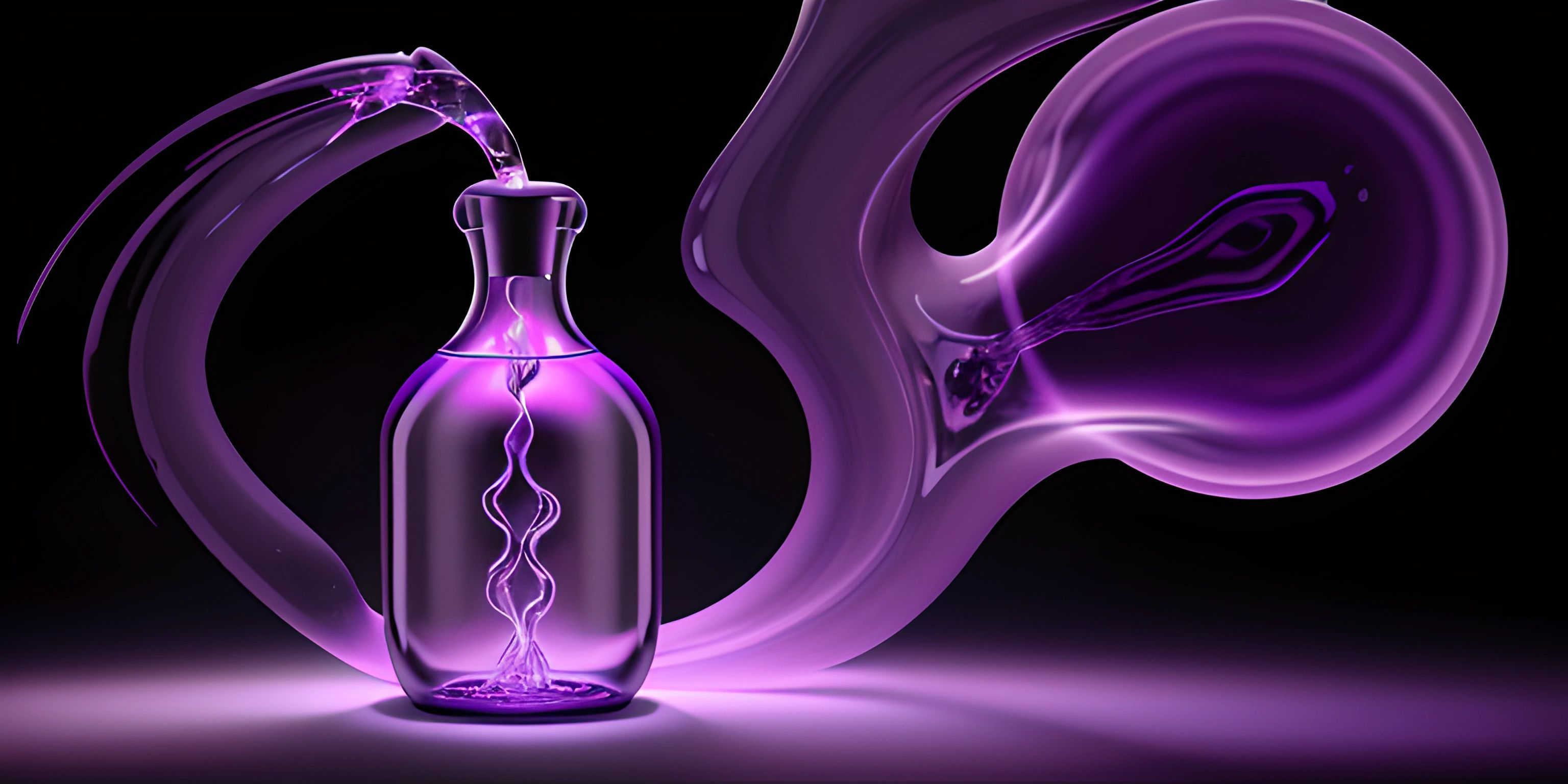
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Elixir, a dynamic, functional language built on the Erlang VM, has a special power called metaprogramming. This power allows you to write code that generates more code at compile-time, like a software-based Inception. Let's dive into this fascinating world and see how it can make our Elixir programs more flexible and powerful, while looking stylish in our code editor.
The Power of Macros
Metaprogramming in Elixir is built around the concept of macros. Macros are essentially chunks of code that get executed at compile-time, generating more code in the process. It's like a magic trick where the magician pulls another magician out of a hat, and that magician pulls another magician out of their hat, and so on.
Before we get too deep into macros, it's important to understand Elixir's Abstract Syntax Tree (AST). The AST is a tree-like structure that represents the syntactic structure of our code. It's the source of truth for our macros, as they manipulate and generate code based on the AST.
Defining Macros
To define a macro in Elixir, we use the defmacro
keyword. This tells the compiler we're creating a macro and not just a regular function. Like the name suggests, macros are all about transforming code, so they take a code block as an argument and return a new code block as a result.
Let's look at a simple example of a macro that doubles the value of an expression:
defmodule DoubleMacro do defmacro double(expression) do quote do unquote(expression) * 2 end end end
Here, we're defining a macro called double
that takes an expression
as its argument. The quote
and unquote
functions are crucial for metaprogramming in Elixir, as they allow us to work with the AST. The quote
function captures the AST of the block of code inside it, while the unquote
function injects the value of expression
back into the quoted code.
Using Macros
To use our shiny new double
macro, we need to import it into our module and call it like any other function:
defmodule MyApp do import DoubleMacro def my_function do double(21) end end
When we call my_function
, it will return 42
, as the double
macro generates the code that multiplies the input expression by 2
at compile-time.
Macro Hygiene
An important concept in Elixir metaprogramming is macro hygiene. This refers to how macros should avoid introducing or capturing variables that could conflict with the surrounding code. Elixir takes care of this automatically by generating unique variable names when necessary. You can use the var!/2
function if you want to explicitly capture or introduce a variable, but this should be done with caution.
Conclusion
Elixir metaprogramming and macros are powerful tools that allow you to manipulate and generate code at compile-time. They can help make your programs more expressive, flexible, and DRY (Don't Repeat Yourself). But with great power comes great responsibility, so always remember the importance of macro hygiene and be cautious when using these techniques. Now go forth and create code that writes code, you Elixir sorcerer!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).