Pattern Matching in Elixir
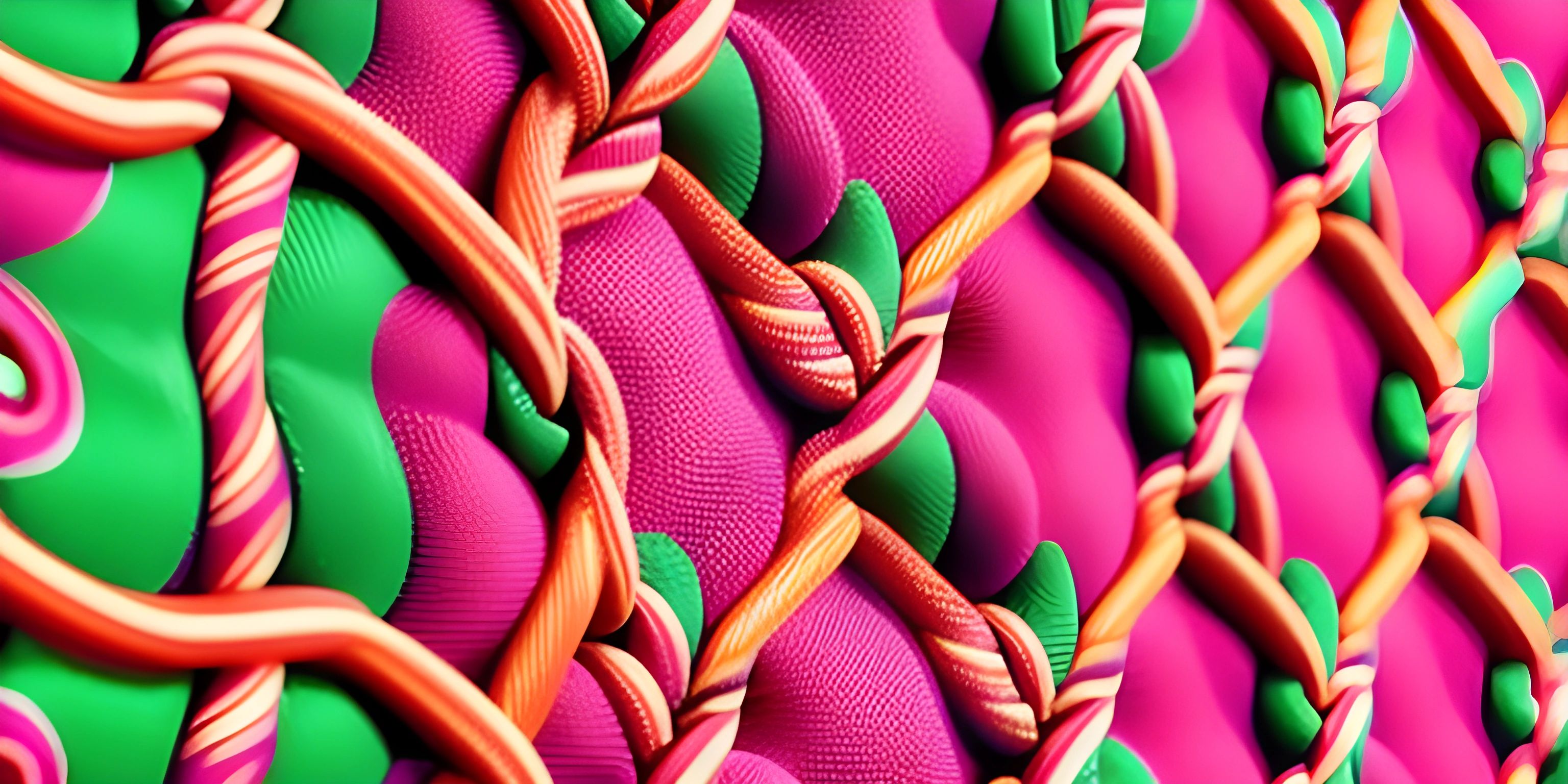
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever played with those childhood puzzle games where you fit different shaped blocks into their matching slots, you already have a basic understanding of pattern matching. In Elixir, it's not a game, but a fundamental concept that powers the language's elegance and expressiveness.
What is Pattern Matching?
Simply put, pattern matching in Elixir is a way of comparing and assigning values. It allows you to check if a certain pattern exists within your data, and if it does, to pull out and work with the parts you're interested in.
The Match Operator
The match operator =
is the heart of pattern matching in Elixir. Unlike in other languages where =
denotes assignment, in Elixir, it's more like a balance scale. Both sides of the =
must balance out or match
.
Here's a simple example:
x = 1
In this example, 1
is matched to x
. Easy, right? But, there's more to it when you start working with complex data types.
Pattern Matching with Lists
Lists are a common data structure in Elixir. Here's how you can use pattern matching with lists:
list = [1, 2, 3] [head | tail] = list
The list [1, 2, 3]
is matched with [head | tail]
. The head
corresponds to the first element of the list and tail
corresponds to the rest of the list. So, after matching, head
will be 1
and tail
will be [2, 3]
.
The Pin Operator
Sometimes, you want to prevent re-binding of a variable during pattern matching. This is where the pin operator ^
comes in. Let's see an example:
x = 1 ^x = 2
This will cause a MatchError because we are trying to match 2
with 1
. The pin operator ^
prevents x
from being re-bound to 2
.
Pattern matching is a powerful concept in Elixir that allows for more expressive, elegant, and maintainable code. So next time you're sifting through your data, remember, it's not just about matching socks, it's about pattern matching.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Enums (psst, it's free!).
FAQ
What is pattern matching in Elixir?
Pattern matching in Elixir is a way of comparing and assigning values. It allows you to check if a certain pattern exists within your data, and if it does, to pull out and work with the parts you're interested in.
What is the match operator in Elixir?
The match operator in Elixir is =
. It's used to compare and match values on both sides of the operator. If the values or patterns don't match, it results in a MatchError.
How can I prevent a variable from being re-bound during pattern matching?
You can prevent a variable from being re-bound during pattern matching in Elixir using the pin operator ^
.
What happens when patterns don't match in Elixir?
If the patterns don't match during pattern matching in Elixir, a MatchError is raised.
Can I use pattern matching with complex data types in Elixir?
Yes, you can use pattern matching with complex data types in Elixir. It's commonly used with lists and tuples to extract and work with specific data.