Understanding Control Structures in Elixir
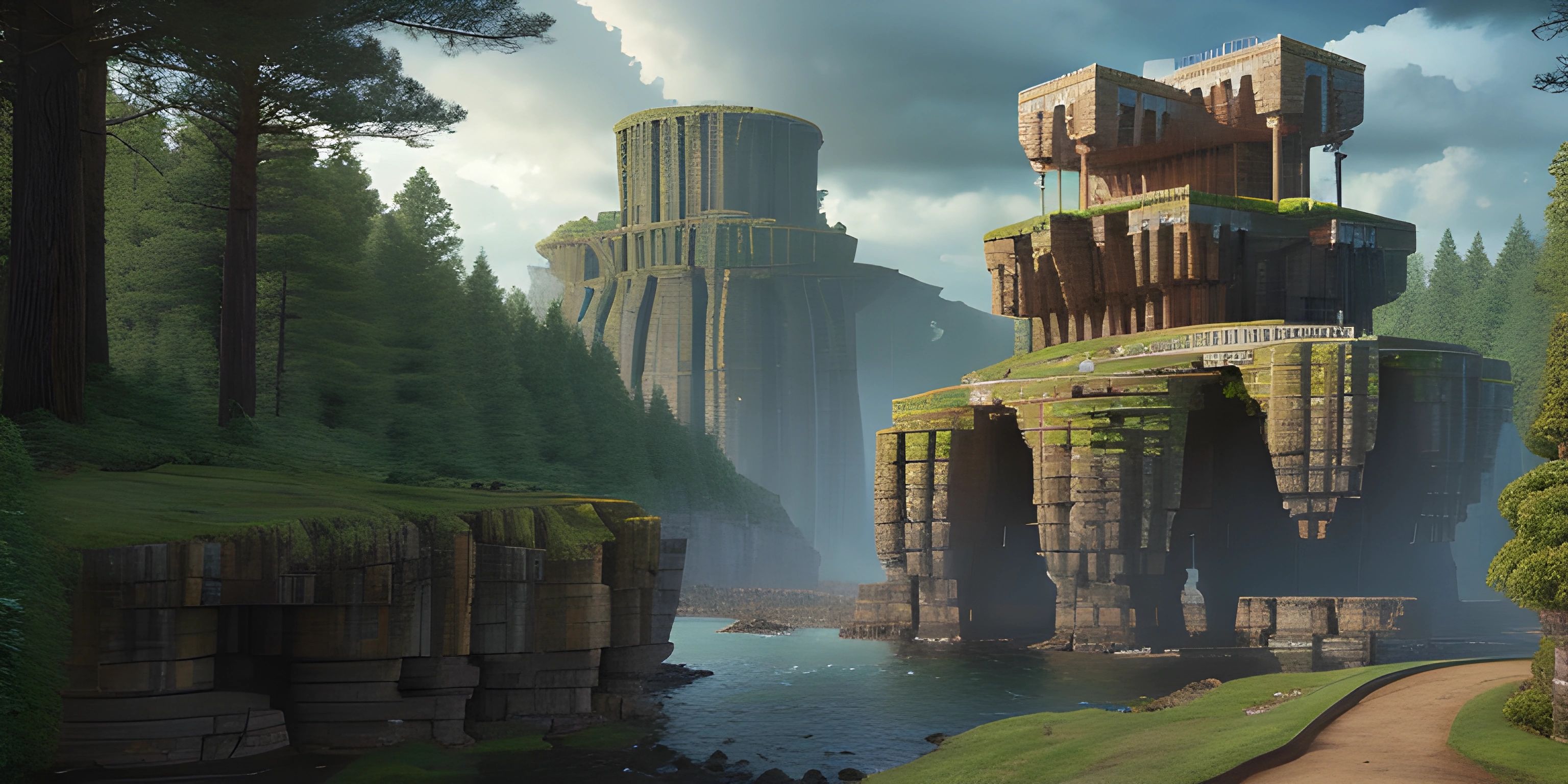
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Elixir, the vibrant and robust programming language that it is, offers a bunch of control structures that allow us to steer our code's execution flow. These structures help us create more complex, dynamic, and interactive programs. Buckle up, and let's embark on this exciting journey!
What are Control Structures?
In Elixir, just like in other programming languages, control structures are the beating heart that keeps the code alive. These structures guide the program's flow and make decisions based on certain conditions. They are the crossroads where your program decides whether to go left or right.
Conditional Structures
Let's start with the bread-and-butter of control structures - the conditional statements. In Elixir, we have if/else
, unless
and cond
all ready to jump into action based on the conditions we set.
Here's a tiny example for an if/else
statement:
if 5 > 3 do IO.puts "Math is fun!" else IO.puts "Math is confusing!" end
In this case, since 5 is indeed greater than 3, our program will print "Math is fun!" But if we lived in some alternate universe where 3 is greater than 5, it would print "Math is confusing!"
Loops
Next on the agenda, we have loops. Now, Elixir does things a little differently. It doesn't have the traditional for
or while
loops like other languages. It uses recursion for looping, which makes everything feel like magic!
Here's a simple recursive function that acts as a loop:
def print_multiple_times(message, n) when n > 0 do IO.puts message print_multiple_times(message, n - 1) end
This function will keep printing the given message until n
reaches 0. The magic of recursion!
Case Structures
case
is another powerful control structure in Elixir. It's like a multi-lane highway for your code, where each lane (or clause) is a potential path your program could take.
Here's an example:
case File.read("example.txt") do {:ok, content} -> IO.puts "File read successfully: #{content}" {:error, reason} -> IO.puts "Failed to read file: #{reason}" end
In this case, (no pun intended) the File.read
function will try to read the file "example.txt". If successful, it will print the content. But if it fails, it will print the reason for failure.
And that's a quick peek into the world of control structures in Elixir!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are control structures in Elixir?
Control structures in Elixir refer to the constructs that manipulate the flow of execution in a program. They include conditional structures like if/else, unless, and cond, looping through recursion, and multiple pathways using case structures.
How does Elixir handle loops?
Elixir handles loops through recursion. It doesn't have traditional for or while loops as seen in other languages. Instead, Elixir uses recursive function calls to implement looping behavior.
What is a case structure in Elixir?
A case structure in Elixir is a powerful control structure that allows your program to choose from multiple paths based on the evaluation of an expression. It matches the result of an expression with different patterns and executes the associated code block.
How do I use if/else in Elixir?
In Elixir, you can use if/else like this: if condition do ... else ... end
. The code after do will be executed if the condition is true, otherwise, the code after else will be executed.
What is the use of control structures?
Control structures are used to alter the flow of execution in a program based on specific conditions and loops. They allow programs to make decisions and perform repetitive tasks, which enables creating more dynamic and interactive applications.