Elixir Basic Syntax
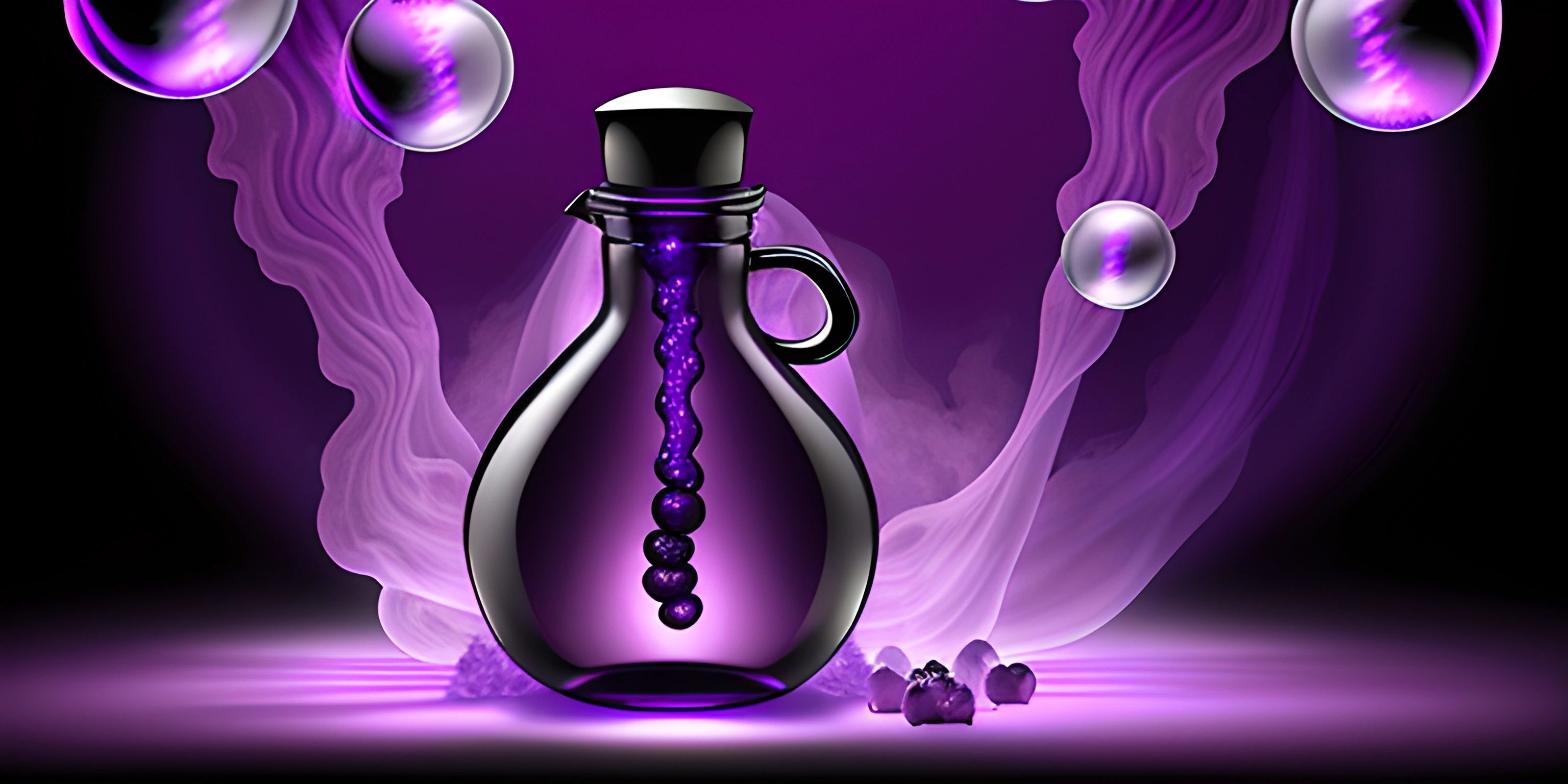
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Elixir is a dynamic, functional language designed for building scalable and maintainable applications. It leverages the Erlang VM, known for running low-latency, fault-tolerant systems. But before we jump into building world-saving applications, let's have a look at Elixir's basic syntax and structure.
Modules and Functions
In Elixir, code is organized into modules. Modules consist of functions that are used to perform specific tasks. You can think of modules as a recipe book and functions as the individual recipes.
Here's an example of a simple Elixir module:
defmodule Greetings do def hello do "Hello, world!" end end
In this example, we have a module called Greetings
and a single function called hello
. The function returns the string "Hello, world!"
.
Function Calls
Functions can be called by specifying their module, followed by a dot, and the function name. Let's call our hello
function:
IO.puts Greetings.hello()
This code will output "Hello, world!" onto the console. The IO.puts
is another Elixir function that is used to print messages to the console.
Variables and Pattern Matching
Elixir has a unique way of working with variables. In Elixir, variables are used for pattern matching.
Here's an example to illustrate this concept:
{status, message} = {:ok, "Success!"}
In this example, we are pattern matching the tuple {:ok, "Success!"}
with the tuple {status, message}
. The variable status
will be assigned the value :ok
, and message
will be assigned the value "Success!"
.
Control Structures
Elixir provides several control structures like if
, case
, and cond
to help with decision making.
Here's an example using the if
control structure:
number = 42 if number > 0 do "This is a positive number." else "This is a non-positive number." end
In this example, we use the if
control structure to check if number
is greater than 0. If it is, we return the string "This is a positive number." Otherwise, we return "This is a non-positive number."
Comments
To make your code more readable and maintainable, Elixir allows you to write comments. Comments start with a #
symbol and span until the end of the line.
# This is a single-line comment in Elixir
Wrapping Up
We've only scratched the surface of Elixir's basic syntax and structure. As you continue exploring Elixir, you'll discover its powerful pattern matching, expressive control structures, and modular design that will make your coding experience a delightful one. So go forth and conquer the world of Elixir!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What are the basic data types in Elixir?
Elixir has several basic data types:
- Integers: Whole numbers like 1, 42, or -7.
- Floats: Decimal numbers like 3.14, -0.01, or 6.0.
- Booleans: Either
true
orfalse
. - Atoms: Constants with their own name as their value, like
:ok
or:error
. - Strings: Text enclosed in double quotes, like "Hello, World!".
- Lists: Ordered collections of elements, like [1, 2, 3].
- Tuples: Similar to lists, but faster for fixed-size collections, like {1, 2, 3}.
How do I define a variable in Elixir?
To define a variable in Elixir, simply use the assignment operator =
. For example:
name = "Alice" age = 30
This creates two variables, name
with the value "Alice" and age
with the value 30.
What is pattern matching in Elixir?
Pattern matching is a powerful feature in Elixir that allows you to match the structure of data and assign values to variables. It uses the =
operator, similar to assignment. For example:
{a, b, c} = {1, 2, 3}
This code matches the structure of the tuple on the left with the tuple on the right, and assigns the values 1, 2, and 3 to the variables a
, b
, and c
, respectively.
How do I define a function in Elixir?
Functions in Elixir are defined using the def
keyword inside a module. Here's an example of defining a function that adds two numbers:
defmodule Math do def add(a, b) do a + b end end
This code defines a module named Math
and a function named add
that takes two arguments, a
and b
, and returns their sum.
How do I call a function in Elixir?
To call a function in Elixir, you typically use the module name followed by a dot and the function name, passing any required arguments. For example, if you have the Math
module with the add
function defined as in the previous question, you'd call it like this:
result = Math.add(1, 2)
This would call the add
function in the Math
module with the arguments 1 and 2, and store the result (3) in the variable result
.