Elixir Processes and Message Passing
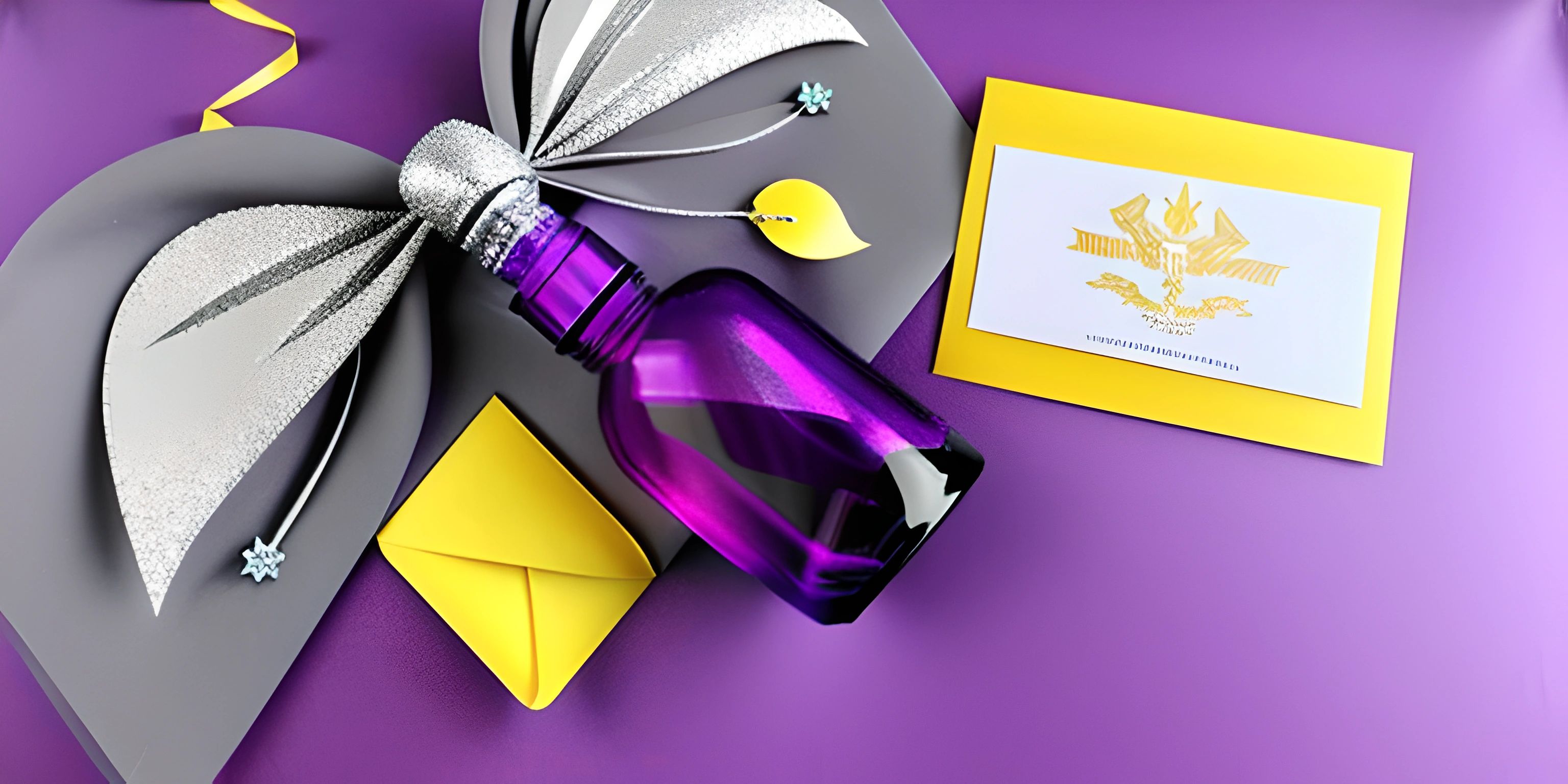
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Elixir, a language crafted for building scalable and maintainable applications, shines when it comes to concurrency. The secret sauce behind Elixir's concurrency model lies in its support for processes and message passing. These concepts, inspired by the Actor Model, give developers the tools to create efficient, concurrent applications.
Processes in Elixir
Processes in Elixir are lightweight and isolated, running concurrently and independently from one another. Unlike operating system processes or threads, Elixir processes are managed by the language's runtime system, the Erlang Virtual Machine (BEAM).
Creating a new process in Elixir is as simple as calling the spawn
function. Let's see an example:
spawn(fn -> IO.puts("Hello from a new process!") end)
The spawn
function accepts an anonymous function (a lambda) and creates a new process to run it. In this case, the new process will output "Hello from a new process!".
Message Passing
Elixir processes communicate through message passing. When you send a message to a process, it goes into the process's mailbox. The receiving process can then retrieve and handle these messages in the order they were received, using the receive
construct.
Here's a simple example of message passing between two processes:
defmodule Greeter do def greet(pid) do send(pid, {:greeting, "Hello from Greeter process!"}) end end receiver = spawn(fn -> receive do {:greeting, message} -> IO.puts("Received: #{message}") end end) Greeter.greet(receiver)
In this example, we define a Greeter
module that contains a greet
function. The function accepts a process ID (pid) and sends a tuple {:greeting, "Hello from Greeter process!"}
to the given process. Next, we spawn a new process, which will wait for a message using the receive
construct. After spawning the receiver process, we call the Greeter.greet
function, passing the receiver's process ID. The receiver will then display the received message.
Concurrency and Fault Tolerance
Elixir processes provide fault tolerance by running independently; if one process fails, it doesn't crash the whole system. Additionally, processes can be supervised, allowing for automatic restarts or other recovery strategies when a failure occurs.
In conclusion, processes and message passing form the backbone of concurrency in Elixir. They allow efficient communication between isolated processes, enabling developers to build scalable and fault-tolerant applications. Remember, with great power comes great responsibility - it's up to you to harness the full potential of Elixir's concurrency model and create remarkable applications!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are Elixir processes, and why are they important for concurrency?
Elixir processes are lightweight, isolated units of computation that can run concurrently in the Elixir runtime. They are important for concurrency because they allow multiple tasks to execute simultaneously, leading to efficient and scalable applications. Elixir processes are not the same as operating system processes, as they are managed by the Erlang Virtual Machine (BEAM) and consume fewer resources.
How does message passing work in Elixir processes?
Message passing is the primary method of communication between Elixir processes. To pass a message, one process sends a message to another process's mailbox using the send/2
function. The receiving process then reads the message from its mailbox using the receive/1
block. Here's an example:
parent = self() spawn(fn -> send(parent, {:greeting, "Hello from the child process!"}) end) receive do {:greeting, message} -> IO.puts(message) end
Can Elixir processes maintain state?
Yes, Elixir processes can maintain state. Since processes are isolated from one another, they can store and manage their own state without interfering with other processes. To maintain state, a process can use tail recursion in a loop, receiving messages and updating its state accordingly. For example:
defmodule Counter do def start() do spawn(fn -> loop(0) end) end defp loop(current_count) do receive do :increment -> loop(current_count + 1) {:report, caller} -> send(caller, {:count, current_count}) loop(current_count) end end end
What is the role of the `spawn/1` function in Elixir processes?
The spawn/1
function is used to create a new Elixir process. It takes a single argument, an anonymous function, which defines the code to be executed in the new process. The spawn/1
function returns the process ID (PID) of the newly created process. For example:
pid = spawn(fn -> IO.puts("Hello from a new process!") end)
How do you link Elixir processes and monitor them for crashes?
To link Elixir processes, you can use the spawn_link/1
function, which works similarly to spawn/1
but also links the parent and child processes. If one process crashes, the other process will also be terminated. To monitor a process, you can use the Process.monitor/1
function, which returns a reference. When the monitored process terminates, a :DOWN
message with the reference is sent to the monitoring process. For example:
pid = spawn_link(fn -> raise "Oops!" end) ref = Process.monitor(pid) receive do {:DOWN, ^ref, :process, _object, reason} -> IO.puts("Process crashed: #{reason}") end