Elixir Distributed Systems
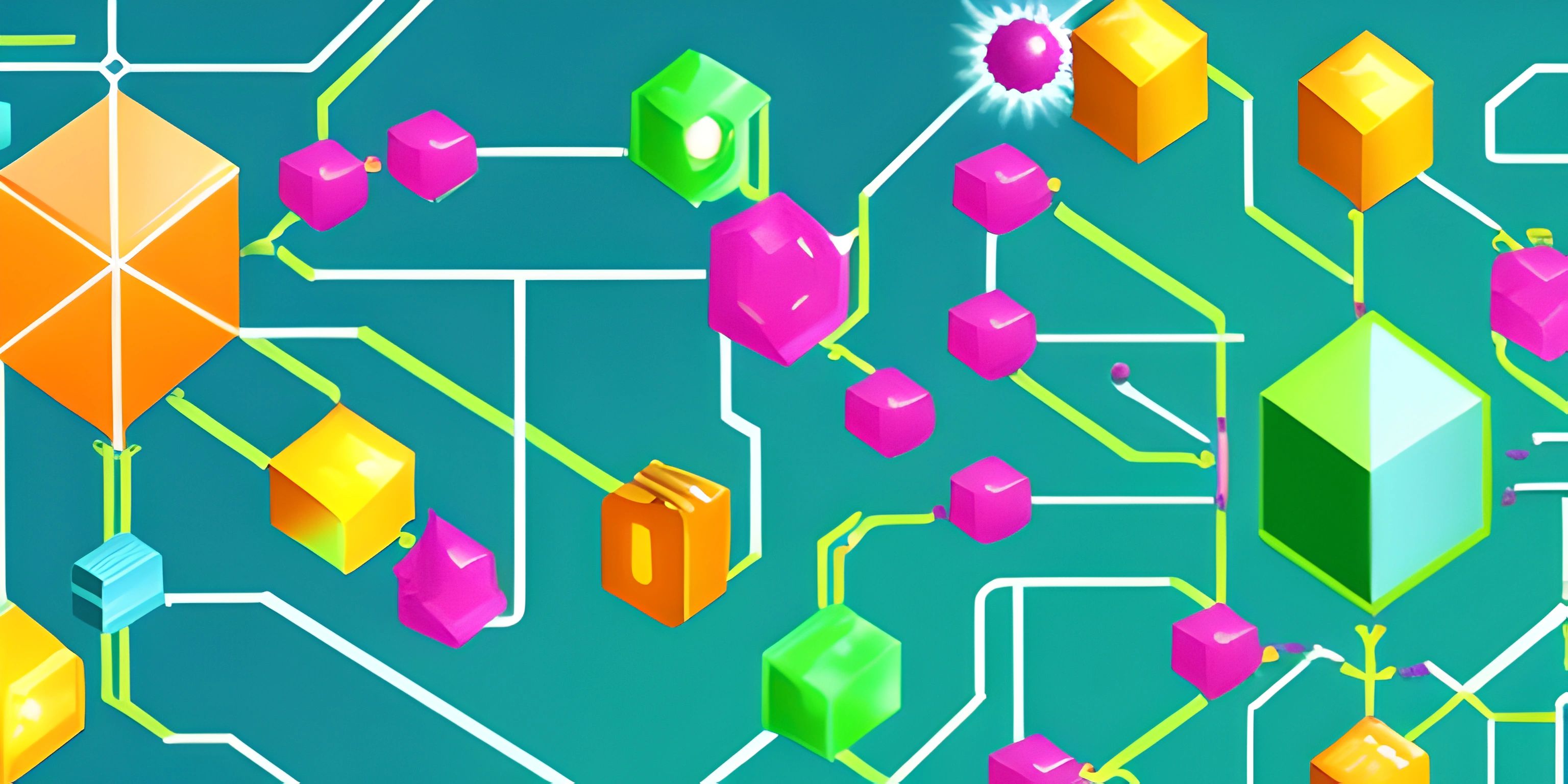
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of applications, scalability and fault-tolerance are highly desirable traits. Elixir, being built on the powerful Erlang VM (BEAM), has some fantastic features that can help us achieve these goals, making it an excellent choice for implementing distributed systems. In this article, we'll discuss Elixir's core concepts and explore how to create a distributed application.
Elixir and Erlang for Distributed Systems
Elixir is a functional, concurrent, and distributed programming language designed for building fault-tolerant and scalable applications. It leverages the power of the Erlang VM, which has been battle-tested in the telecommunication industry for decades. The combination of Elixir's elegant syntax and the robustness of the Erlang VM make it a popular choice for building distributed systems.
The Actor Model and Concurrency
Elixir's concurrency model is based on the Actor Model, where independent processes communicate by sending and receiving messages. This model allows for high concurrency and fault-tolerance, as processes are isolated from each other, preventing cascading failures.
OTP (Open Telecom Platform) Framework
Another advantage of using Elixir for distributed systems is its OTP Framework that provides battle-tested abstractions for building distributed applications. OTP includes powerful tools like supervisors for managing process lifecycles, GenServers for implementing server processes, and applications for organizing code.
Building a Distributed Elixir Application
Now that we understand the advantages of using Elixir for distributed systems, let's dive into building a simple distributed application.
Setting up the Project
First, create a new Elixir project using the mix
command:
mix new distributed_example cd distributed_example
Implementing a GenServer
Start by implementing a GenServer that will handle the core functionality of our distributed application. In this example, we'll create a simple key-value store.
Create a new file called kv_store.ex
in the lib
directory and add the following code:
defmodule DistributedExample.KVStore do use GenServer # Starts the GenServer def start_link(_) do GenServer.start_link(__MODULE__, %{}, name: __MODULE__) end # Initializes the state def init(_) do {:ok, %{}} end # Handle the put operation def handle_cast({:put, key, value}, state) do {:noreply, Map.put(state, key, value)} end # Handle the get operation def handle_call({:get, key}, _from, state) do {:reply, Map.get(state, key), state} end end
This code defines a simple GenServer that stores key-value pairs in its state. We can put and get values using the handle_cast
and handle_call
callbacks, respectively.
Connecting Nodes and Distributing Work
Now that we have our GenServer, it's time to connect nodes in a distributed system. First, start two instances of our application:
# Terminal 1 (Node 1) iex --name [email protected] --cookie secret -S mix # Terminal 2 (Node 2) iex --name [email protected] --cookie secret -S mix
These commands start two instances of our application with different node names, using the same cookie for authentication. Now we can connect the nodes:
# In Node 1 Node.connect(:"[email protected]")
With our nodes connected, we can now distribute the work between them. For instance, we can start the KVStore on both nodes and put and get values from either node:
# In Node 1 DistributedExample.KVStore.start_link([]) # In Node 2 DistributedExample.KVStore.start_link([]) # In Node 1 GenServer.cast(DistributedExample.KVStore, {:put, :message, "Hello, world!"}) # In Node 2 GenServer.call(DistributedExample.KVStore, {:get, :message})
The value "Hello, world!" is now accessible from both nodes, demonstrating a simple example of a distributed Elixir application.
While this example is basic, Elixir offers a plethora of tools and techniques for building more advanced, fault-tolerant, and scalable distributed systems. Embrace Elixir's powerful features and build resilient applications that can withstand the challenges of modern computing.
FAQ
What is Elixir and why is it suitable for distributed systems?
Elixir is a functional, concurrent, and general-purpose programming language that runs on the Erlang Virtual Machine (BEAM). It is particularly suitable for distributed systems due to its lightweight processes, fault-tolerance, concurrency, and the ability to scale horizontally. These features make it ideal for building reliable and efficient distributed applications.
How do Elixir processes work in distributed systems?
In Elixir, processes are lightweight and run independently, making them an excellent choice for distributed systems. Processes communicate with each other using asynchronous message passing, which allows for concurrency and fault tolerance. They can be distributed across different nodes in the system, enabling the application to scale horizontally and handle a large number of simultaneous requests.
What role does the Erlang Virtual Machine (BEAM) play in Elixir distributed systems?
The Erlang Virtual Machine (BEAM) provides the runtime environment for Elixir applications. It is responsible for managing processes, garbage collection, and scheduling. BEAM enables Elixir to inherit the fault-tolerance and concurrency features from Erlang, which are essential for building distributed systems. It also allows Elixir applications to interact with Erlang libraries and modules, further enhancing its capabilities for creating scalable and resilient distributed applications.
How do I create Elixir nodes and connect them in a distributed system?
To create Elixir nodes and connect them in a distributed system, you need to start the Elixir runtime with a unique node name and the appropriate cookie for authentication. Here's an example of starting two Elixir nodes:
elixir --sname node1 --cookie mycookie -S mix run elixir --sname node2 --cookie mycookie -S mix run
Once the nodes are up and running, you can connect them using the Node.connect/1
function:
Node.connect(:"node1@hostname")
Replace "hostname" with the actual hostname of the machine on which the node is running.
What is the role of OTP in Elixir distributed systems?
OTP (Open Telecom Platform) is a set of libraries and design principles for building fault-tolerant and scalable applications in Erlang. Elixir, being built on top of Erlang, also benefits from OTP. OTP provides abstractions such as GenServer, Supervisor, and Application, which help developers create robust distributed systems with Elixir. These abstractions simplify the process of managing processes, handling failures, and organizing applications into modular components.