Integrating Redux with React for Scalable State Management
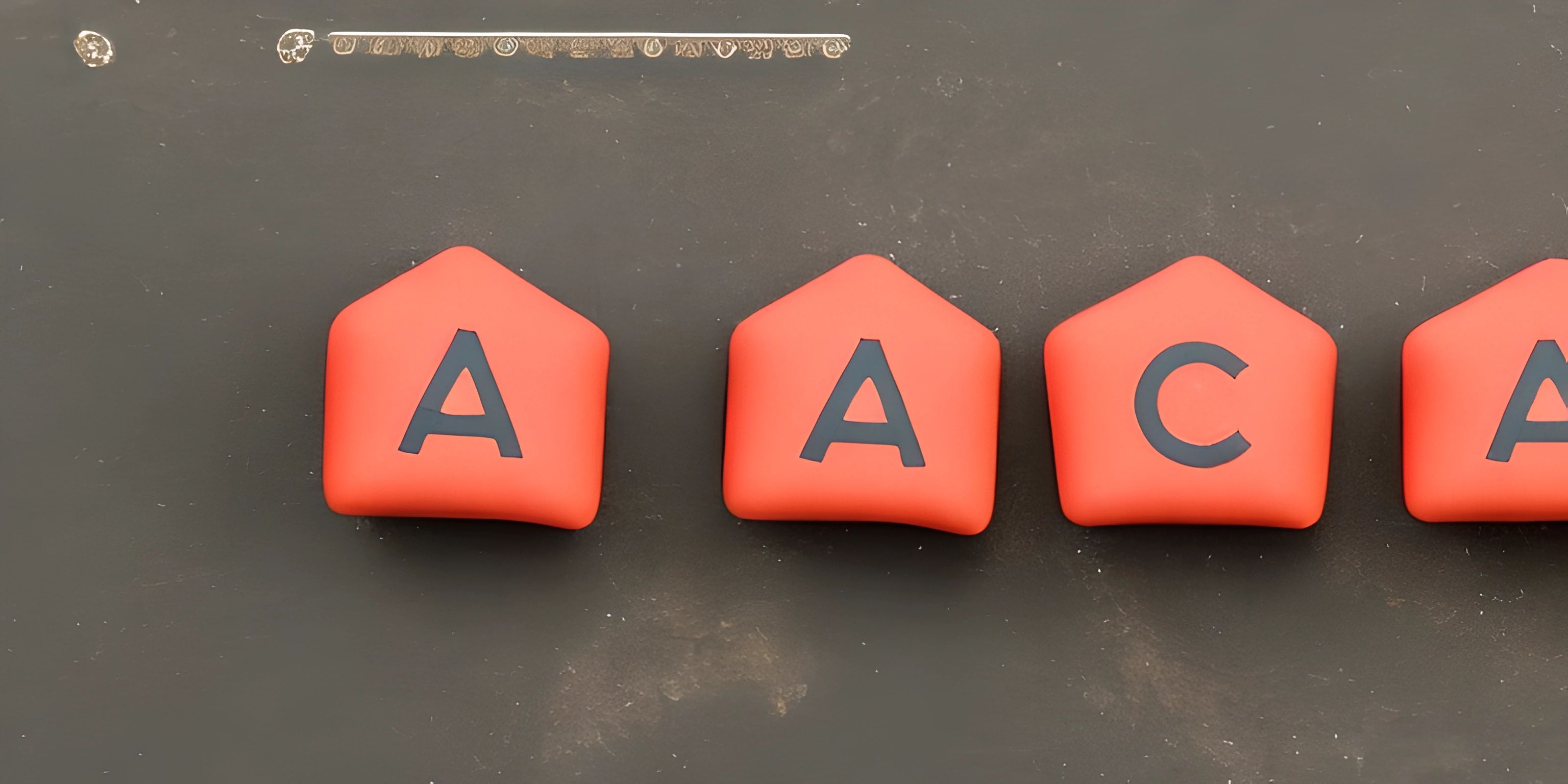
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React is known for its component-based architecture, which makes it easy to reuse code and build applications quickly. However, managing state in a large React application can be challenging, especially when many components need access to the same data. That's where Redux comes into play.
Redux is a predictable state container for JavaScript applications. It provides a central store to manage the state of your entire application, making it easier to track changes and debug issues. Combining Redux with React allows you to create scalable applications with well-managed state.
Setting up Redux in a React Project
Before we dive into integrating Redux with React, let's set up a simple React application. You can use create-react-app
to generate a new project.
npx create-react-app react-redux-example cd react-redux-example
Next, install the necessary Redux packages:
npm install redux react-redux
Now, you can start integrating Redux into your React application.
Creating the Redux Store
First, you need to create a Redux store that holds the state of your application. In the src
folder, create a new folder called store
, and inside it, create a file named index.js
. Add the following code:
import { createStore } from "redux"; import rootReducer from "../reducers"; const store = createStore(rootReducer); export default store;
Here, we import the createStore
function from Redux and a rootReducer
which we'll create next. The createStore
function is used to create the Redux store by passing in the rootReducer
.
Creating Reducers
Reducers are pure functions that take the current state and an action, then return a new state. Create a new folder called reducers
in the src
directory, and inside it, create a file named index.js
. Add the following code:
import { combineReducers } from "redux"; import exampleReducer from "./exampleReducer"; const rootReducer = combineReducers({ example: exampleReducer }); export default rootReducer;
Here, we import the combineReducers
function from Redux, which allows us to combine multiple reducers into a single root reducer. We also import an exampleReducer
which we'll create next.
Create a new file in the reducers
folder called exampleReducer.js
:
const initialState = { data: [] }; const exampleReducer = (state = initialState, action) => { switch (action.type) { case "ADD_DATA": return { ...state, data: [...state.data, action.payload] }; default: return state; } }; export default exampleReducer;
We define an initial state and create the exampleReducer
function. Inside the reducer, we handle an ADD_DATA
action that adds data to the state.
Connecting Redux to React
Now that we have the Redux store and reducers set up, let's connect them to our React application. Open the src/index.js
file and wrap the App
component with the Provider
component from react-redux
:
import React from "react"; import ReactDOM from "react-dom"; import { Provider } from "react-redux"; import store from "./store"; import App from "./App"; ReactDOM.render( <Provider store={store}> <App /> </Provider>, document.getElementById("root") );
Now, Redux is connected to your React application, and you can access the store from any component using the useSelector
and useDispatch
hooks.
Accessing and Updating State in Components
In your components, you can use the useSelector
hook to access the state and the useDispatch
hook to dispatch actions to update the state. Here's an example:
import React from "react"; import { useSelector, useDispatch } from "react-redux"; const ExampleComponent = () => { const data = useSelector((state) => state.example.data); const dispatch = useDispatch(); const addData = (newData) => { dispatch({ type: "ADD_DATA", payload: newData }); }; return ( <div> {/* Render data */} <button onClick={() => addData("New data")}>Add Data</button> </div> ); }; export default ExampleComponent;
In this example, we access the data
from the Redux state and display it. We also have a button that adds new data to the state by dispatching the ADD_DATA
action.
That's it! You've successfully integrated Redux with React for scalable state management in your application. Now you can focus on building more complex and feature-rich applications without worrying about state management chaos. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).
FAQ
What is the purpose of integrating Redux with React?
Integrating Redux with React allows for better state management within your application, making it more predictable and easier to maintain. This integration is particularly useful when building large-scale applications, as it helps to manage the complexity of the application's state and logic.
How do I install and set up Redux with React?
To install and set up Redux with React, follow these steps:
- Install the required packages:
npm install redux react-redux
- Create a Redux store to hold the application's state.
- Use the
Provider
component fromreact-redux
to make the store available to all components within your application. - Connect your React components to the Redux store using the
connect
function fromreact-redux
.
How do I create a Redux store for my React application?
Creating a Redux store requires three main steps:
- Define your application's state structure.
- Create reducer functions to handle state updates.
- Create the Redux store by invoking
createStore
and passing the root reducer. Here's an example of creating a simple Redux store:
import { createStore } from "redux"; const initialState = { counter: 0 }; function rootReducer(state = initialState, action) { switch (action.type) { case "INCREMENT": return { ...state, counter: state.counter + 1 }; case "DECREMENT": return { ...state, counter: state.counter - 1 }; default: return state; } } const store = createStore(rootReducer);
How do I connect my React components to the Redux store?
To connect your React components to the Redux store, use the connect
function from react-redux
. The connect
function takes two arguments: mapStateToProps
and mapDispatchToProps
. The first maps the Redux state to the component's props, while the second maps dispatch functions to the component's props.
Here's an example of connecting a React component to the Redux store:
import React from "react"; import { connect } from "react-redux"; function Counter({ counter, increment, decrement }) { return ( <div> <button onClick={decrement}>-</button> <span>{counter}</span> <button onClick={increment}>+</button> </div> ); } const mapStateToProps = (state) => ({ counter: state.counter }); const mapDispatchToProps = (dispatch) => ({ increment: () => dispatch({ type: "INCREMENT" }), decrement: () => dispatch({ type: "DECREMENT" }) }); export default connect(mapStateToProps, mapDispatchToProps)(Counter);
Can I use Redux with React hooks?
Yes, you can use Redux with React hooks by utilizing the useSelector
and useDispatch
hooks from the react-redux
package. The useSelector
hook allows you to access the Redux state within your functional components, while the useDispatch
hook provides access to the dispatch
function. Here's an example:
import React from "react"; import { useSelector, useDispatch } from "react-redux"; function Counter() { const counter = useSelector((state) => state.counter); const dispatch = useDispatch(); const increment = () => dispatch({ type: "INCREMENT" }); const decrement = () => dispatch({ type: "DECREMENT" }); return ( <div> <button onClick={decrement}>-</button> <span>{counter}</span> <button onClick={increment}>+</button> </div> ); } export default Counter;