Route Parameters in Express.js
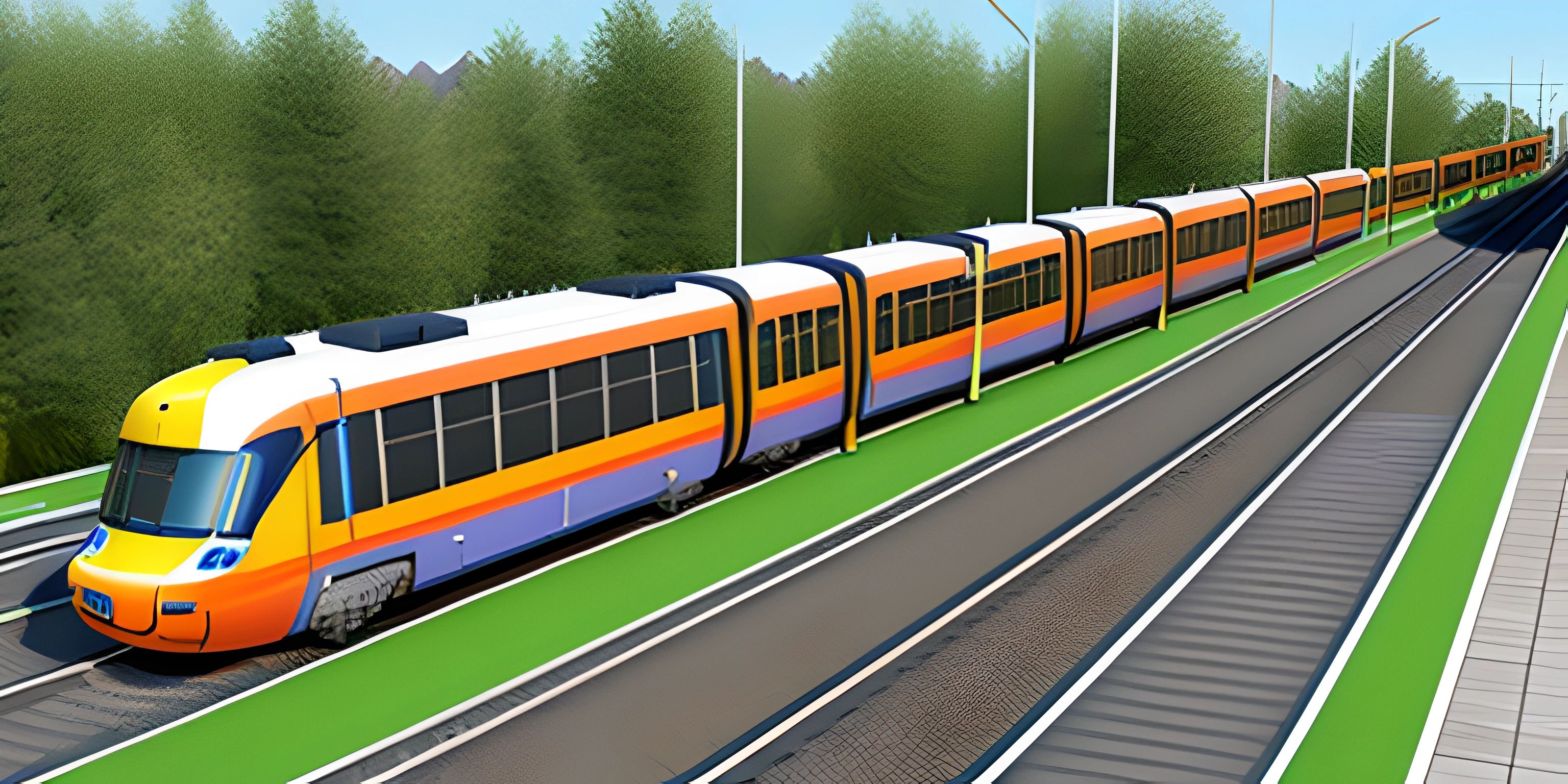
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Creating dynamic routes in your Express.js web applications is a powerful way to make your application more adaptable and responsive to user input. Route parameters provide an elegant way to achieve this, allowing you to define variables within your route URLs that can be captured and used within your route handlers.
What are Route Parameters?
In Express.js, route parameters allow you to define variables within your route URLs. When a request is made to the route, these variables are captured and can be accessed within the route handler. This allows you to create dynamic routes that can handle different inputs and respond accordingly.
Defining Route Parameters
To define a route parameter, you simply include a :
followed by the variable name within the route URL. For example, to define a route parameter userId
in a route, you would write:
app.get("/user/:userId", (req, res) => { // Route handler code here });
Accessing Route Parameter Values
Within your route handler, you can access the value of a route parameter using req.params
. The params
object contains key-value pairs, where the key is the name of the route parameter and the value is the actual value provided in the request URL.
Here's an example of how you can access the userId
route parameter value from the previous example:
app.get("/user/:userId", (req, res) => { const userId = req.params.userId; res.send(`User ID: ${userId}`); });
Now, if the client makes a request to /user/123
, the route handler will capture the userId
parameter value (123
) and send back a response with the content User ID: 123
.
Using Multiple Route Parameters
You can also define multiple route parameters within a single route URL. For example, let's say you want to create a route that retrieves a specific post for a given user:
app.get("/user/:userId/post/:postId", (req, res) => { const userId = req.params.userId; const postId = req.params.postId; res.send(`User ID: ${userId}, Post ID: ${postId}`); });
This route will now capture both the userId
and postId
parameter values, allowing you to handle requests for specific user posts more flexibly.
Route Parameter Tips
- Route parameters are case-sensitive. If you define a route with a parameter
:userId
, it will not match a request with:UserId
. - You can use regular expressions to further refine the route parameter matching process. For example, you can ensure that a route parameter only captures numeric values by adding a regular expression pattern, like
/user/:userId(\\d+)
.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).
FAQ
What are route parameters in Express.js?
Route parameters are variables defined within route URLs in Express.js. They allow you to capture and access specific values from the request URL within your route handlers, enabling you to create dynamic routes that can handle different inputs and respond accordingly.
How do I define a route parameter in Express.js?
To define a route parameter, include a :
followed by the variable name within the route URL. For example, to define a route parameter userId
, you would write: app.get("/user/:userId", (req, res) => {...});
.
How can I access route parameter values in Express.js?
Within your route handler, you can access the value of a route parameter using req.params
. This object contains key-value pairs, where the key is the name of the route parameter and the value is the actual value provided in the request URL.
Can I use multiple route parameters in a single route?
Yes, you can define multiple route parameters within a single route URL. This allows you to create even more flexible and adaptable routes that can handle a variety of inputs.
Can I use regular expressions with route parameters?
Yes, you can use regular expressions to further refine the route parameter matching process. For example, you can ensure that a route parameter only captures numeric values by adding a regular expression pattern, like /user/:userId(\\d+)
.