For Loops Deconstructed
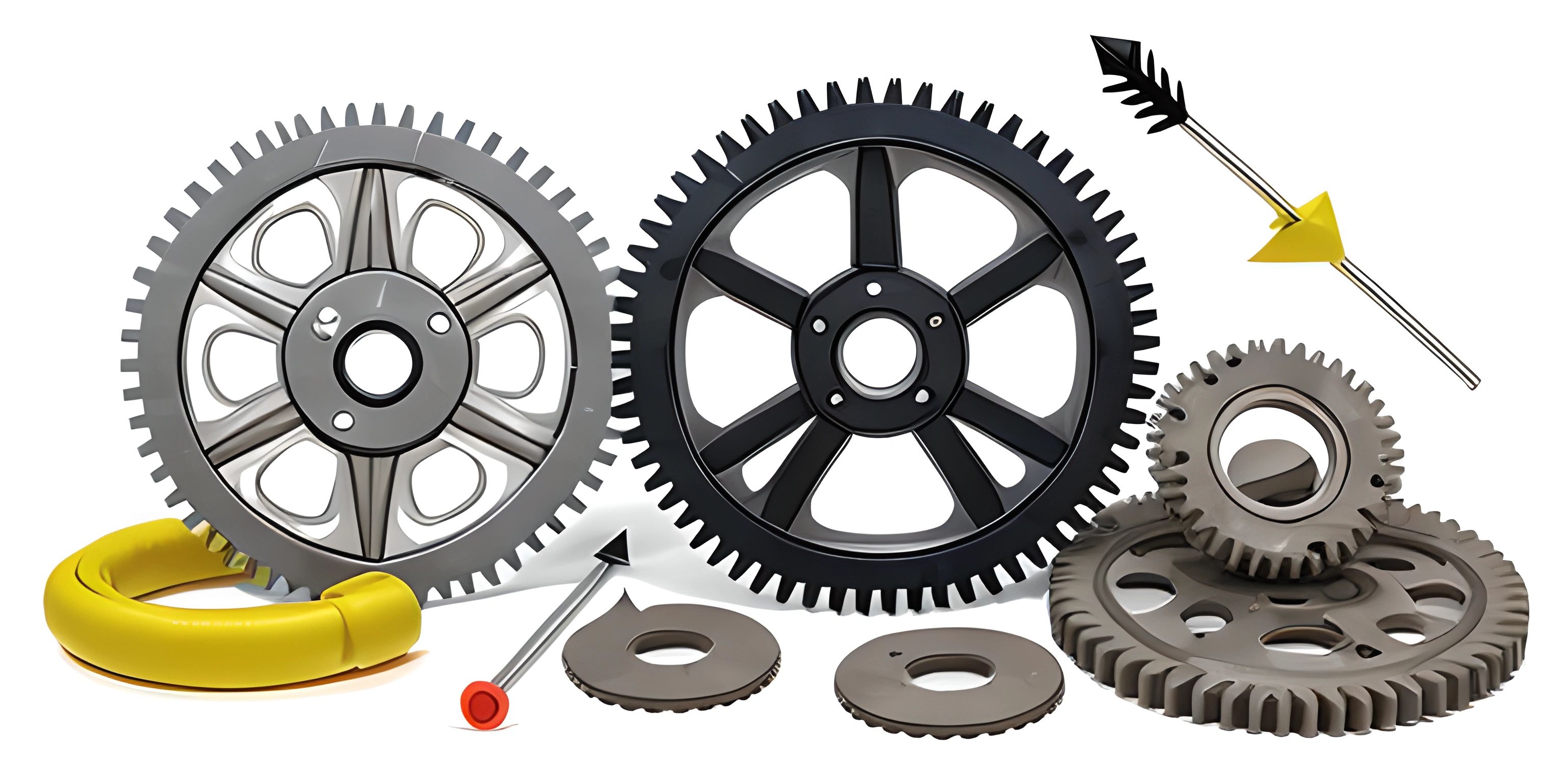
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're a robot on an assembly line, repeating the same task over and over. You wouldn't want to rewire your brain's circuitry every time you need to perform the task, right? That's where loops come in handy, and specifically, the workhorse of the looping world: the for loop.
For Loop Basics
A for loop is a control-flow structure in programming that allows you to repeat a block of code a specific number of times. It's universally applicable, as it's found in almost every programming language, and it's a key tool for making your code more efficient and concise.
Anatomy of a For Loop
Let's examine the general structure of a for loop, using pseudocode:
for (initialization; condition; update) { // Code to be repeated }
- Initialization: This is where you define a starting point, usually by declaring a loop counter variable. It's executed only once, right before the loop starts.
- Condition: This is a boolean expression that checks if the loop should continue running. If it evaluates to
true
, the loop will execute the code block. If it'sfalse
, the loop will terminate. - Update: After each iteration, this expression updates the loop counter variable. It often involves incrementing or decrementing the counter.
For Loop in Action
Let's see a for loop in action, using a common programming task: printing numbers from 1 to 5:
for (int i = 1; i <= 5; i++) { print i }
Here's how the for loop works, step-by-step:
- The loop counter variable
i
is initialized with a value of1
. - The condition
i <= 5
is checked. Since1
is less than or equal to5
, the condition istrue
. - The code block is executed, printing the value of
i
(1
). - The update expression
i++
incrementsi
by1
. Now,i
is2
. - Steps 2-4 are repeated until
i
is greater than5
, at which point the loop terminates.
Nesting For Loops
For loops can also be nested within one another, which is useful when dealing with multi-dimensional data or when you need to perform a task multiple times based on another loop. Here's an example of nested for loops to print a 3x3 grid of asterisks:
for (int i = 1; i <= 3; i++) { for (int j = 1; j <= 3; j++) { print "* " } print "\n" // Move to the next line }
In this example, the outer loop iterates three times, and for each iteration, the inner loop also iterates three times, printing an asterisk. After the inner loop completes, a newline character is printed to move to the next row, creating a 3x3 grid of asterisks.
Loop Control Statements
Two useful loop control statements are break
and continue
. break
terminates the loop immediately, while continue
skips the rest of the current iteration and proceeds to the next one.
Here's an example of using break
and continue
in a for loop to print all numbers from 1
to 10
, except for 5
:
for (int i = 1; i <= 10; i++) { if (i == 5) { continue } print i }
When the loop counter variable i
is 5
, the continue
statement is executed, skipping the print i
line and moving on to the next iteration.
Now that you've got a grip on for loops, it's time to put them to use in your programming endeavors. Just remember: like any tool, for loops can be overused, so always consider if a loop is the most efficient way to solve a problem. Happy looping!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is a for loop in programming?
A for loop is a type of control structure in programming languages that allows you to execute a block of code repeatedly for a specified number of times. It is often used when you know in advance how many iterations you want to perform. The loop consists of four main components: initialization, condition, execution, and update. Here's an example in Python:
for i in range(5): print("Iteration", i)
How do I write a for loop in different programming languages?
The syntax for writing a for loop varies across programming languages, but the concept remains the same. Here are examples of for loops in Python, JavaScript, and C++: Python:
for i in range(5): print("Iteration", i)
JavaScript:
for (let i = 0; i < 5; i++) { console.log("Iteration", i); }
C++:
#include <iostream> using namespace std; int main() { for (int i = 0; i < 5; i++) { cout << "Iteration " << i << endl; } return 0; }
How do I use a for loop to iterate over a collection of items?
In many programming languages, you can use a for loop to iterate over a collection of items, such as an array or list. This allows you to perform an operation on each item in the collection. Here's an example in Python:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print("I like", fruit)
Can I use nested for loops in my code?
Yes, you can use nested for loops when you need to perform iterations within iterations. This is often the case when working with multi-dimensional data structures like matrices or grids. Here's an example in Python of using nested for loops to print out a multiplication table:
for i in range(1, 6): for j in range(1, 6): print(i * j, end="\t") print() # Print a newline for the next row
How do I control the execution of a for loop with break and continue statements?
break
and continue
statements are used to control the flow of your loop. The break
statement exits the loop immediately, while the continue
statement skips the current iteration and moves to the next one. Here's an example in Python:
for i in range(1, 11): if i == 5: continue # Skip printing the number 5 if i == 8: break # Stop the loop when i reaches 8 print(i)