While Loops
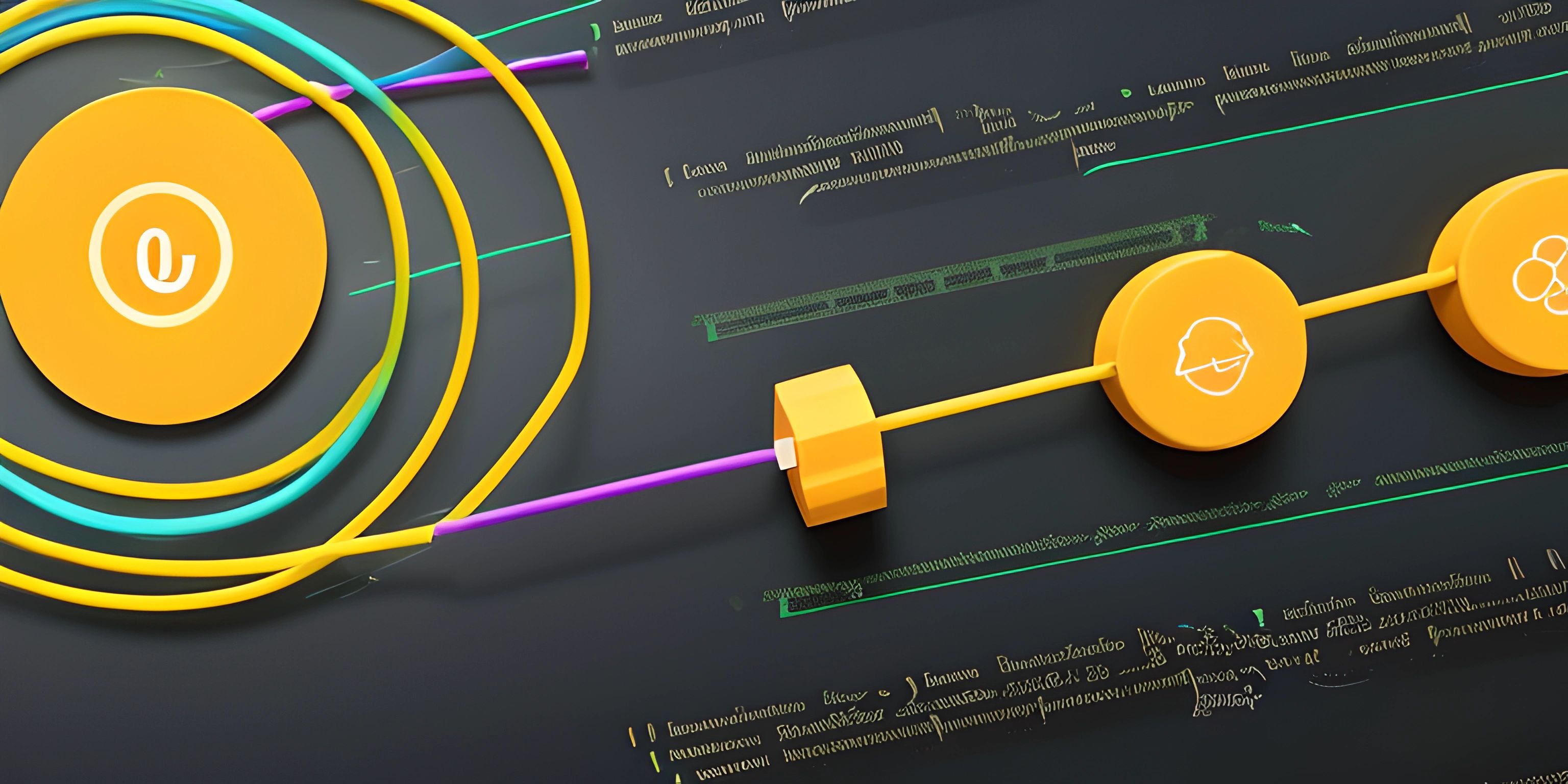
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you want to become a programming wizard, you must master the art of loops. Today, we'll focus on the magical incantations known as while loops. Just like a skilled magician can repeat a trick, a programmer can use loops to repeat a piece of code until a certain condition is met.
The Sorcery of While Loops
A while loop is a control flow structure that repeats a block of code as long as a specified condition remains true. When the condition becomes false, the loop exits, and the program continues with the next line of code.
Picture yourself as a code conjurer, and you need to create a potion that requires 10 drops of a magical ingredient. A while loop can help you do that without having to manually count and drop each ingredient. Here's a pseudocode example:
drops = 0 while drops < 10: add drop to potion drops = drops + 1
In this example, the loop iterates as long as the drops
variable is less than 10. Each iteration adds a drop to the potion and increments the drops
variable by 1. Once drops
reaches 10, the loop ends, and your potion is complete!
Advantages of While Loops
-
Code efficiency: Instead of writing the same code multiple times, a while loop lets you repeat a block of code with a single declaration. This makes your code cleaner and easier to maintain.
-
Dynamic repetition: While loops can adapt to changing conditions. If your potion suddenly requires 15 drops instead of 10, simply change the condition, and the loop will adjust accordingly.
-
Controlled execution: With while loops, you have full control over when the loop starts, how long it runs, and when it ends. This is useful when the number of iterations depends on external factors, like user input or data from a file.
While Loop Examples
Let's take a look at some code examples using different programming languages.
Python
count = 0 while count < 5: print("This is iteration", count) count += 1
JavaScript
let count = 0; while (count < 5) { console.log("This is iteration", count); count++; }
Java
int count = 0; while (count < 5) { System.out.println("This is iteration " + count); count++; }
These examples demonstrate how to use while loops in various languages. Notice how all three examples achieve the same goal with similar syntax.
Now that you've been initiated into the arcane knowledge of while loops, you can harness their power to create efficient, adaptable, and controlled code. Go forth and conjure your programming spells!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is a while loop and how does it work in programming?
A while loop is a control flow structure in programming that allows a block of code to be executed repeatedly as long as a certain condition is met. It works by continuously checking the condition, and if it remains true, the loop keeps executing the code block. Once the condition becomes false, the loop is terminated, and the program continues with the next statements outside the loop.
How do I write a basic while loop in Python?
In Python, you can write a basic while loop using the following syntax:
while condition: # Your code here
Here's a simple example that prints the numbers from 1 to 5:
counter = 1 while counter <= 5: print(counter) counter += 1
What are the advantages of using while loops in programming?
While loops offer a few advantages in programming:
- Code Reusability: They allow the same block of code to be executed multiple times without having to rewrite it.
- Dynamic Iterations: They provide flexibility in determining the number of loop iterations based on a condition, rather than a fixed number, which is especially useful when you don't know how many times the loop should run beforehand.
- Efficient Code Execution: While loops can help reduce the size and complexity of your code, making it easier to read, maintain, and debug.
Can a while loop run indefinitely? How can I prevent this?
Yes, a while loop can run indefinitely if its condition never becomes false. This is called an infinite loop and can cause your program to become unresponsive. To prevent this, make sure to include a statement within the loop that changes the value of the condition or provides a way to break out of the loop. Always check your loop's logic and ensure that the condition will eventually become false.
How can I use a 'break' statement in a while loop?
The break
statement is used to exit a while loop prematurely, even if the loop's condition is still true. It is helpful when you want to stop the loop based on a specific event or value. Here's an example in Python:
counter = 1 while counter <= 10: if counter == 6: break print(counter) counter += 1
In this example, the loop stops when the counter
variable reaches 6, even though the original condition (counter <= 10
) is still true.