Loops in Programming
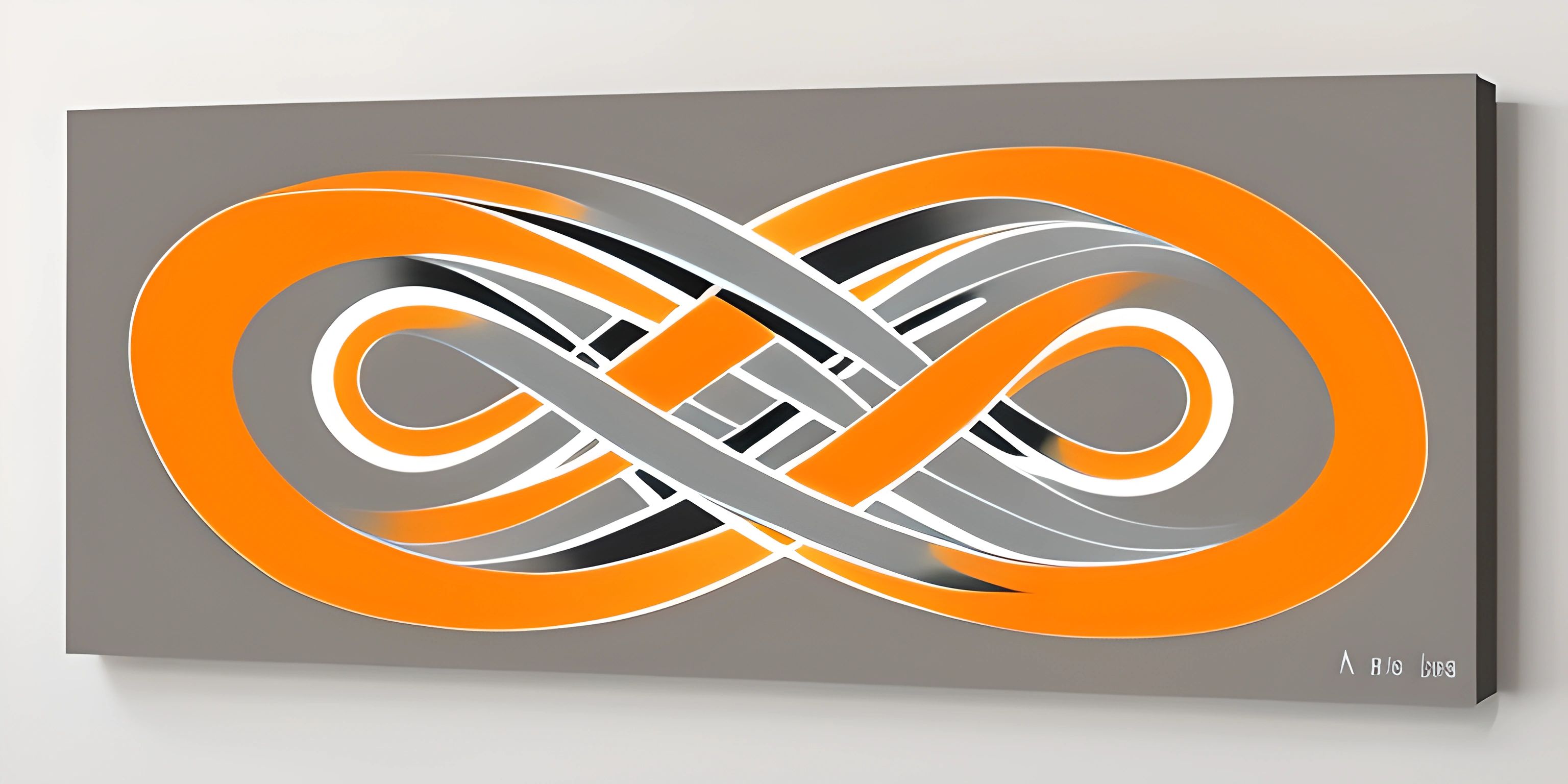
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Loops are like the spinning teacup ride at an amusement park: they keep going until the operator (or a condition) says it's time to stop. In programming, loops are a way to repeat a block of code multiple times until a certain condition is met. They are an essential part of almost every programming language, allowing developers to avoid repetitive code and manage complex tasks more efficiently.
Types of Loops
There are two main types of loops in programming: while loops and for loops. Each of these loop types has its own unique implementation, but they both serve the purpose of executing a block of code repeatedly.
While Loops
A while loop is like a stubborn toddler who won't leave the playground until their parent convinces them to go home. It continues executing the code block as long as a specified condition remains true. Here's a simple pseudocode example:
counter = 0 while counter < 5: print "Spinning on the teacup!" counter = counter + 1
In this example, the loop will keep executing and printing "Spinning on the teacup!" until the counter
variable reaches 5. The loop checks the condition counter < 5
before every iteration, and if it's still true, the code block inside the loop runs again.
For Loops
A for loop, on the other hand, is like a well-planned dinner party with a set guest list. It iterates over a specified sequence (like a list or a range) and executes the code block for each element in that sequence. Here's a pseudocode example:
for guest in guest_list: print "Welcome, " + guest + "!"
In this example, the loop iterates through each element (or guest
) in the guest_list
sequence, printing a personalized welcome message for each one.
Loop Control Statements
Sometimes, you might want to have more control over your loops. That's where loop control statements come in. They can help you alter the flow of your loops, like a master puppeteer pulling the strings. The two main loop control statements are break and continue.
Break
The break statement is like an emergency exit for loops. It immediately halts the loop's execution and jumps out of it, no matter where it is in the iteration. Here's a pseudocode example:
while True: response = read_user_input() if response == "STOP": break print "You entered: " + response
In this example, the loop will keep asking for user input and printing it until the user enters "STOP". When that happens, the break statement will be executed, and the loop will exit.
Continue
The continue statement is like a polite way of saying, "Let's move on to the next topic." It skips the remaining code block in the current iteration and jumps to the next iteration of the loop. Here's a pseudocode example:
for number in range(1, 11): if number % 2 == 0: continue print "Odd number: " + number
In this example, the loop iterates through the numbers 1 to 10. If a number is even, the continue statement executes, skipping the print statement and moving on to the next number. If the number is odd, it gets printed.
Loops are a powerful programming tool that can simplify complex tasks and make your code more efficient. By understanding the different types of loops and the loop control statements, you'll be well-equipped to tackle a wide range of programming challenges. So buckle up and enjoy the ride!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).