Understanding For Loops
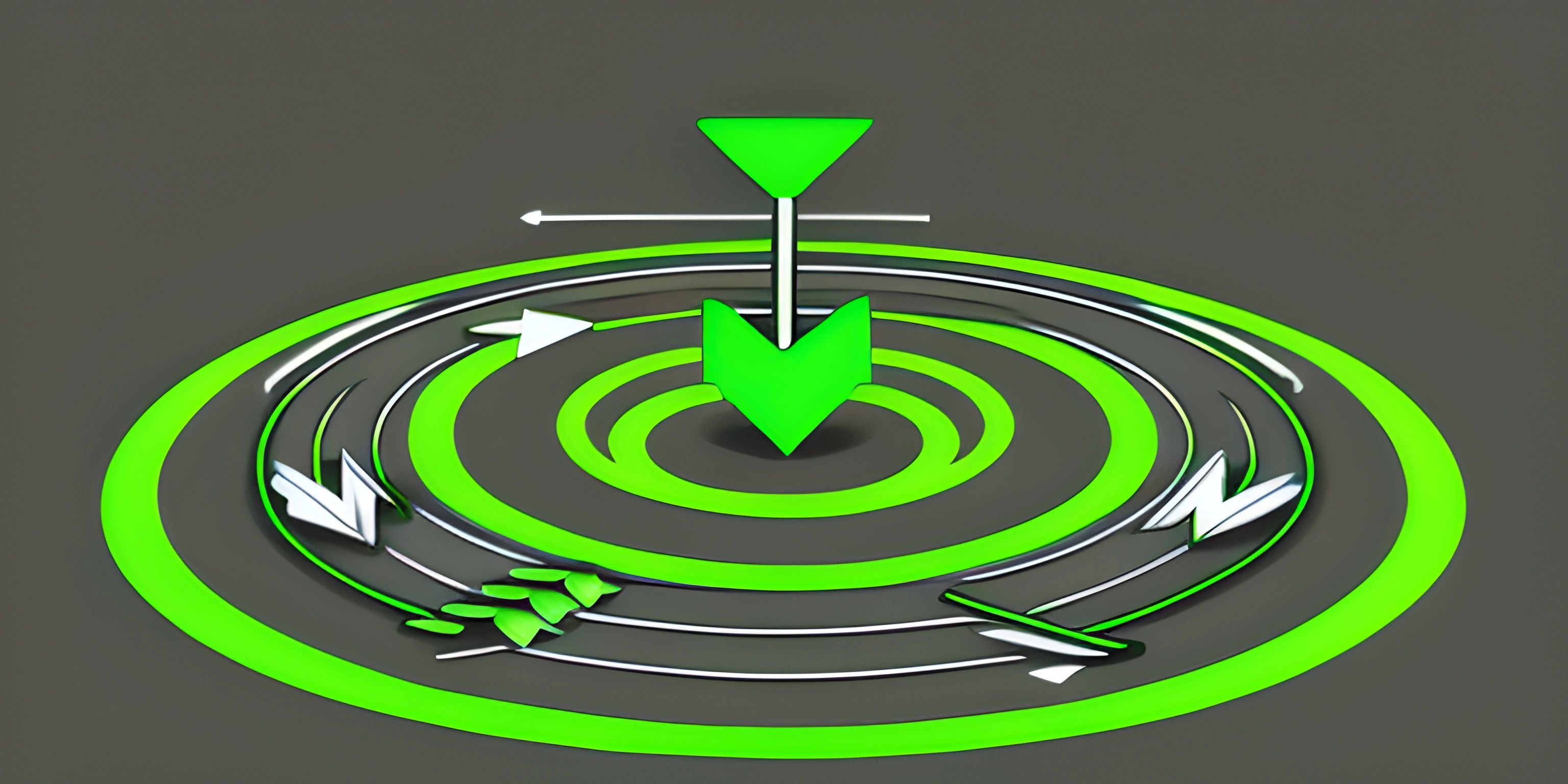
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
For loops are a cornerstone of programming, allowing you to repeat a block of code multiple times with a simple and concise structure. Think of them as the ultimate multi-taskers, like a skilled chef who can chop, dice, and mince in a matter of seconds!
The Anatomy of a For Loop
A for loop is composed of three main parts: the initialization, the condition, and the update. These ingredients work together to create a well-oiled looping machine.
Here's a basic example using JavaScript:
for (let i = 0; i < 5; i++) { console.log("I'm looping!"); }
Initialization
The initialization (let i = 0
) is where we declare and set up our loop counter variable. It's like setting the countdown timer before launching a rocket.
let i = 0;
Condition
The condition (i < 5
) is a test that will be checked before each iteration of the loop. If the condition is true, the loop continues; if it's false, the loop stops. It's the bouncer of the loop, ensuring that only qualified iterations get in.
i < 5;
Update
The update (i++
) modifies the loop counter variable at the end of each iteration. It's like a janitor making sure the loop is tidy and ready for the next run.
i++;
For Loop in Action
With our for loop, the code inside the curly braces {}
will be executed five times, each time printing "I'm looping!" to the console. It's like a well-rehearsed dance routine, with each step perfectly synchronized to the beat of the loop.
Nesting Loops
For loops can be nested inside one another, like Russian dolls or layers of an onion. This allows you to perform more complex iterations, such as traversing a multi-dimensional array or generating a grid of elements. Here's an example of nested for loops to create a multiplication table:
for (let i = 1; i <= 10; i++) { for (let j = 1; j <= 10; j++) { console.log(i * j); } }
In this example, the outer loop iterates through the numbers 1 to 10, while the inner loop does the same for each iteration of the outer loop. The result is a multiplication table from 1 to 100 – a beautiful piece of for loop art!
By understanding the power of for loops and how they work, you'll have a valuable tool in your programming arsenal, ready to conquer any repetitive task that comes your way. Just remember, with great looping power comes great responsibility – use it wisely!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is a for loop and why is it important in programming?
A for loop is a control structure used in programming to repeat a specific block of code for a predetermined number of iterations. It's important because it helps automate repetitive tasks, making the code more efficient and easier to maintain.
How do you write a basic for loop in Python and JavaScript?
In Python, a basic for loop can be written using the following syntax:
for variable in range(start, end, step): # code to be executed
In JavaScript, a basic for loop can be written using the following syntax:
for (let variable = start; variable < end; variable += step) { // code to be executed }
Can you provide an example of a for loop that prints numbers from 1 to 5?
Sure! Here's an example in Python:
for i in range(1, 6): print(i)
And here's an example in JavaScript:
for (let i = 1; i <= 5; i++) { console.log(i); }
What is the difference between a for loop and a while loop?
A for loop is typically used when you know how many times you want to iterate through a block of code. In contrast, a while loop is used when you want to continue looping through the code as long as a specific condition remains true. Both loops are used for repetitive tasks, but their use cases can differ based on the requirements.
How do I exit a for loop early if a certain condition is met?
To exit a for loop early, you can use the break
statement. When the break
statement is encountered, the loop terminates immediately. Here's an example in Python:
for i in range(1, 11): if i == 5: break print(i)
And here's an example in JavaScript:
for (let i = 1; i <= 10; i++) { if (i === 5) { break; } console.log(i); }