Python Loops - For and While
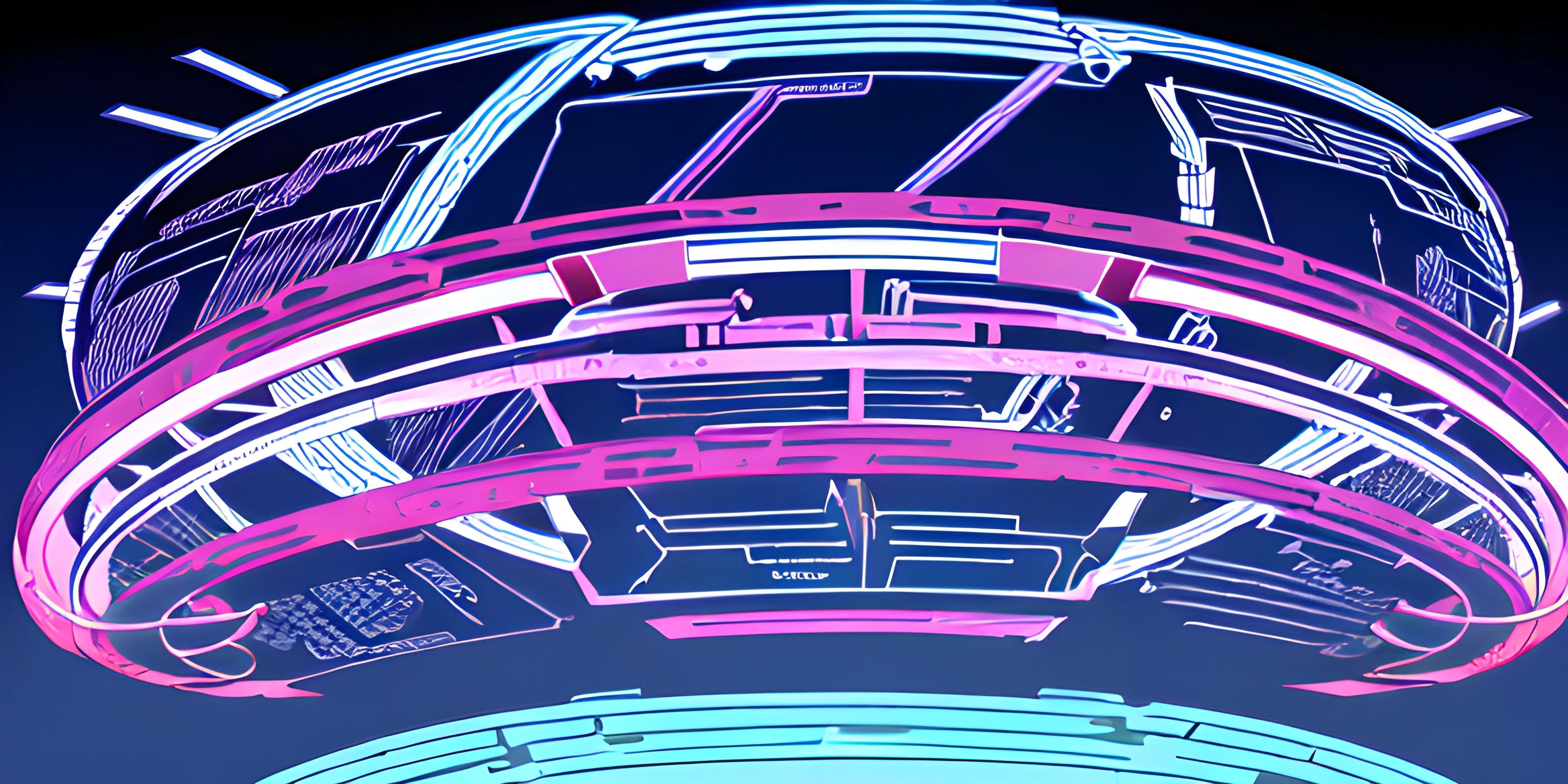
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Loops are one of the most essential and powerful concepts in programming. They allow us to execute a block of code multiple times, making it easy to work with large amounts of data and perform repetitive tasks. In Python, there are two main types of loops: for
loops and while
loops. Let's dive in and see how they work.
For Loops
A for
loop in Python is used to iterate over a sequence, such as a list, tuple, or string. It allows you to execute a block of code for each item in the sequence. The general syntax for a for
loop is:
for variable in sequence: # Code to execute for each item in the sequence
Here's a simple example that iterates through a list of numbers and prints each one:
numbers = [1, 2, 3, 4, 5] for num in numbers: print(num)
You can also use the range()
function to generate a sequence of numbers for iteration. For example, to print numbers 0 through 9:
for i in range(10): print(i)
While Loops
A while
loop in Python executes a block of code as long as a certain condition is True
. The general syntax for a while
loop is:
while condition: # Code to execute while the condition is True
Here's an example that prints numbers from 1 to 5 using a while
loop:
count = 1 while count <= 5: print(count) count += 1
In this example, the loop will continue to execute as long as the value of count
is less than or equal to 5. After each iteration, count
is incremented by 1.
Be careful with while
loops, as it's possible to create an infinite loop if your condition never becomes False
. Always ensure there's a way to break out of the loop.
Loop Control Statements
There are two loop control statements in Python that can alter the flow of loops: break
and continue
.
break
: This statement is used to exit the loop prematurely, stopping any further iterations.continue
: This statement is used to skip the rest of the current iteration and move on to the next one.
Here's an example that demonstrates the use of both break
and continue
:
for i in range(1, 11): if i == 5: continue if i == 8: break print(i)
In this example, the continue
statement skips the number 5, and the break
statement stops the loop when i
reaches 8.
Now that you've got a good grasp of for
and while
loops in Python, you can start iterating through data and performing tasks more efficiently. Happy looping!
FAQ
What are the two main types of loops in Python?
The two main types of loops in Python are for
loops and while
loops. For
loops are used to iterate over a sequence, while while
loops execute a block of code as long as a certain condition is True
.
How do I create a `for` loop in Python?
To create a for
loop in Python, use the following syntax: for variable in sequence:
, followed by an indented block of code to execute for each item in the sequence.
How do I create a `while` loop in Python?
To create a while
loop in Python, use the following syntax: while condition:
, followed by an indented block of code to execute while the condition is True
.
What are loop control statements in Python?
Loop control statements in Python are break
and continue
. Break
is used to exit the loop prematurely, while continue
is used to skip the rest of the current iteration and move on to the next one.
How do I use the `range()` function with a `for` loop?
You can use the range()
function to generate a sequence of numbers for iteration in a for
loop. For example: for i in range(10):
would create a loop that iterates through the numbers 0 to 9.