While Loops Explained
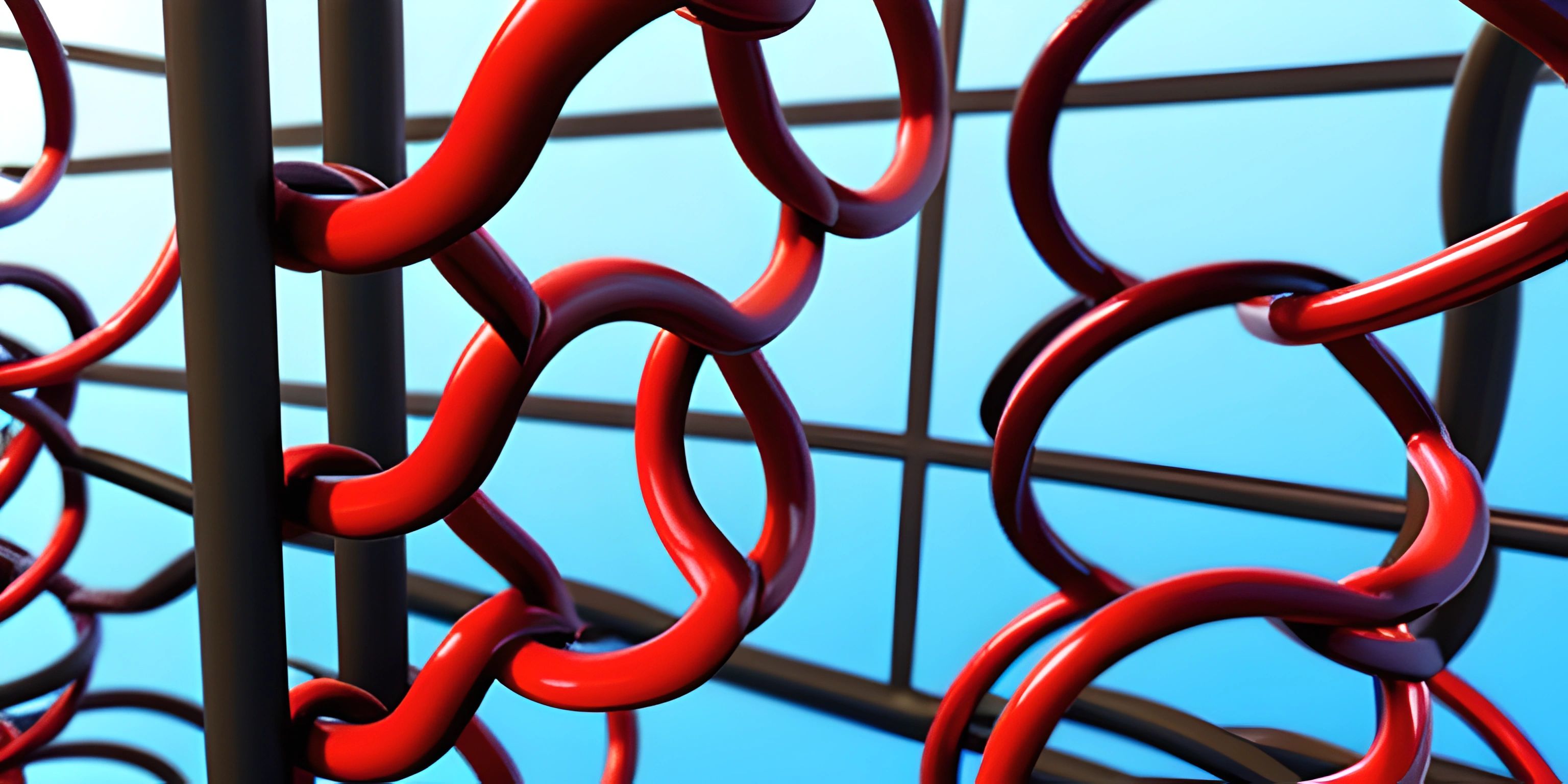
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Loops are a fundamental concept in programming, and a while loop is one of the most basic and versatile types of loops you'll encounter. Let's dive into what while loops are, how they work, and how to implement them in different programming languages.
What is a While Loop?
A while loop is a control structure that repeatedly executes a block of code as long as a specified condition is true. Think of it like a never-ending game of "Simon Says" – as long as Simon keeps saying "Simon says," you keep doing the action. The moment Simon stops saying "Simon says," you're out of the loop.
Here's a visual representation using pseudocode:
while condition is true: execute code
Anatomy of a While Loop
A while loop consists of three main components:
- The keyword
while
: This indicates that you're creating a while loop. - The condition: The loop will continue running as long as this condition evaluates to true.
- The code block: The lines of code that will be executed repeatedly as long as the condition is true.
Let's break down what's happening here:
- The program checks if the condition is true.
- If the condition is true, it executes the code block within the loop.
- It goes back to step 1 and repeats the process until the condition becomes false.
Implementing While Loops
Now that we know what a while loop is and how it works, let's see how we can implement one in several popular programming languages.
Python
counter = 0 while counter < 5: print("Loop iteration", counter) counter += 1
JavaScript
let counter = 0; while (counter < 5) { console.log("Loop iteration", counter); counter++; }
Java
int counter = 0; while (counter < 5) { System.out.println("Loop iteration " + counter); counter++; }
Remember, while loops can be powerful, but also dangerous – if you forget to update the condition, you may end up with an infinite loop! Always make sure your loops have an exit strategy, or you might find yourself trapped in a never-ending game of "Simon Says" with no escape.
Conclusion
A while loop is an essential tool in a programmer's arsenal. By understanding how they work and how to implement them, you'll be able to create more efficient and dynamic programs. As you progress in your programming journey, you'll encounter other types of loops, too, like for loops and nested loops. Remember, practice makes perfect, so keep looping!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Reverse Word Splitter (psst, it's free!).
FAQ
What is a while loop and when should I use it?
A while loop is a fundamental programming construct that repeatedly executes a block of code as long as a specified condition is true. You should use while loops when you need to perform a particular task multiple times but don't know the exact number of iterations beforehand.
How do I implement a while loop in Python?
Implementing a while loop in Python is quite simple. Start with the while
keyword, followed by the condition that needs to be true for the loop to continue, then a colon, and finally the block of code to be executed. Here's an example:
counter = 0 while counter < 5: print("Loop iteration:", counter) counter += 1
How do I implement a while loop in JavaScript?
In JavaScript, you can implement a while loop similar to Python. Use the while
keyword, followed by the condition in parentheses, and then the block of code to be executed in curly braces. Here's an example:
let counter = 0; while (counter < 5) { console.log("Loop iteration:", counter); counter++; }
How do I break out of a while loop if a certain condition is met?
You can break out of a while loop using the break
statement. This statement will immediately exit the loop, regardless of the loop's original condition. Here's an example in Python:
counter = 0 while True: print("Loop iteration:", counter) counter += 1 if counter >= 5: break
How can I use a while loop with an else clause?
In some programming languages like Python, you can use an else
clause with a while loop. The code inside the else
block will be executed only when the loop condition becomes false. Here's an example:
counter = 0 while counter < 5: print("Loop iteration:", counter) counter += 1 else: print("Loop finished!")