Heap Sort Explained
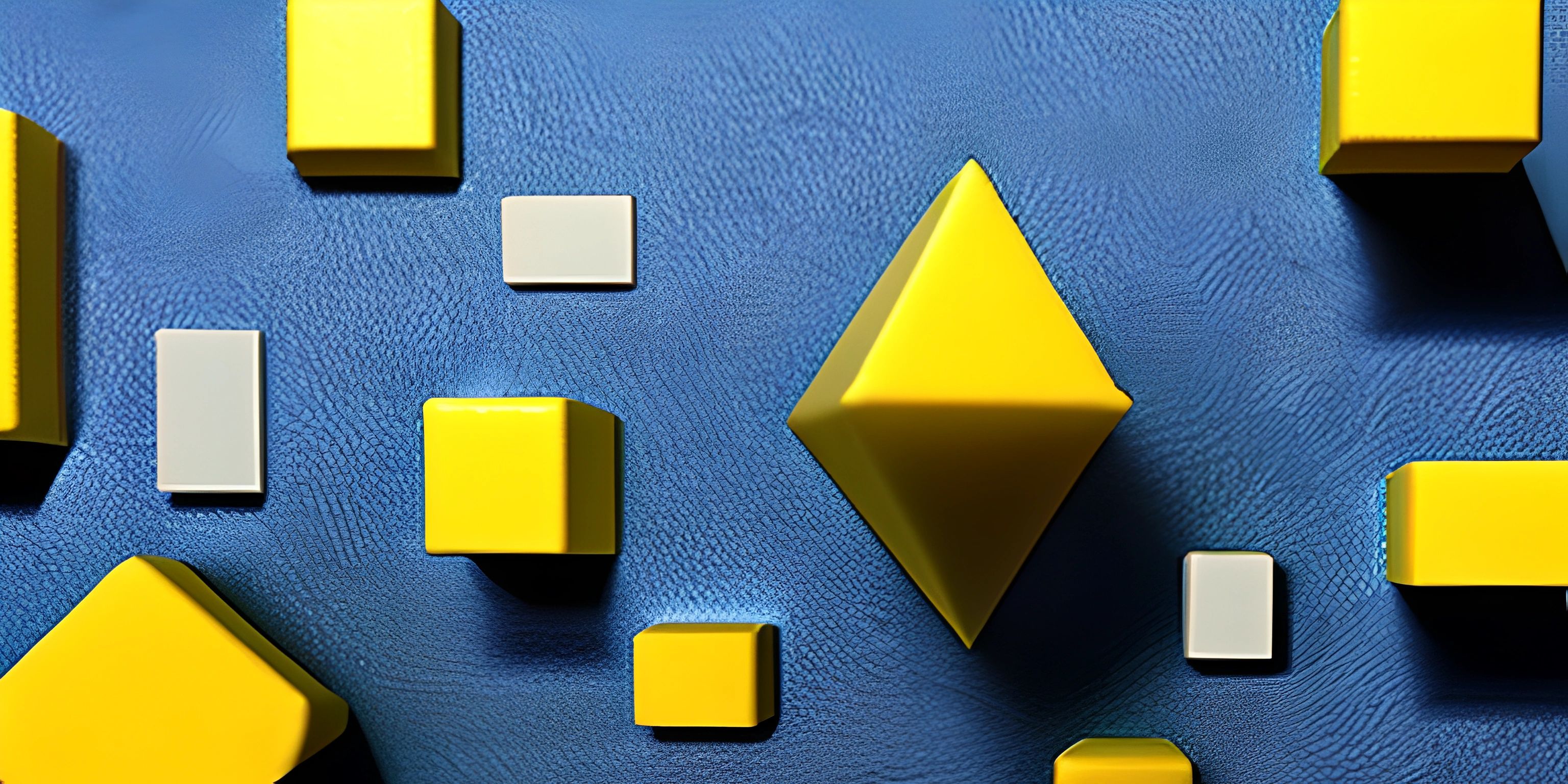
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the realm of sorting algorithms, heap sort stands out as one of the more efficient contenders. It makes use of the binary heap data structure to efficiently sort a list of elements.
What is Heap Sort?
Heap sort is a comparison-based sorting algorithm that transforms an input array into a binary heap and then sorts the data by comparing the elements at the top of the heap. The algorithm works in two main steps:
- Build a binary heap: Transform the input array into a binary heap data structure, either a max-heap or a min-heap.
- Extract elements and sort: Remove the top element from the heap, store it in the sorted array, and reheapify the heap until it's empty.
By using a binary heap, heap sort can achieve an average and worst-case time complexity of O(n log n), making it an efficient and reliable choice for sorting large datasets.
How Heap Sort Works
Let's break down the heap sort algorithm into more detail:
Building a Binary Heap
First, we need to build a binary heap from the input array. A binary heap is a complete binary tree where each node satisfies either the max-heap property or the min-heap property.
- Max-heap property: A node's value is greater than or equal to the values of its children.
- Min-heap property: A node's value is less than or equal to the values of its children.
In the context of heap sort, we'll focus on using a max-heap. The process of building a max-heap is called heapify, and it involves comparing parent nodes with their children and swapping if necessary to ensure the max-heap property is maintained.
Sorting the Array
Once we have a max-heap, we're ready to sort the array. We do this by repeatedly:
- Swapping the root node (the largest element in the heap) with the last element in the heap.
- Removing the last element from the heap and storing it in the sorted array.
- Reheapify the remaining nodes in the heap.
We repeat these steps until the heap is empty, and the sorted array contains all the elements in ascending order.
Example
Here's an example of heap sort in action, using the following array: [9, 3, 5, 1, 6]
.
- Build a max-heap:
[9, 6, 5, 1, 3]
. - Swap the root and last element, remove it from the heap, and store it in the sorted array:
[3, 6, 5, 1]
- sorted:[9]
. - Reheapify the remaining heap:
[6, 3, 5, 1]
. - Swap the root and last element, remove it from the heap, and store it in the sorted array:
[1, 3, 5]
- sorted:[9, 6]
. - Reheapify the remaining heap:
[5, 3, 1]
. - Swap the root and last element, remove it from the heap, and store it in the sorted array:
[1, 3]
- sorted:[9, 6, 5]
. - Reheapify the remaining heap:
[3, 1]
. - Swap the root and last element, remove it from the heap, and store it in the sorted array:
[1]
- sorted:[9, 6, 5, 3]
. - Reheapify the remaining heap:
[1]
. - Remove the last element from the heap and store it in the sorted array:
[]
- sorted:[9, 6, 5, 3, 1]
.
The final sorted array is [1, 3, 5, 6, 9]
.
Use Cases and Advantages
Heap sort is an excellent choice when you need to sort large datasets due to its O(n log n) time complexity. It's also an in-place sorting algorithm, meaning it doesn't require additional memory, which is beneficial when working with limited memory resources.
While heap sort may not be as fast as other sorting algorithms like quicksort in some cases, it has the advantage of a more consistent performance, avoiding the worst-case scenarios that can occur with quicksort.
Heap sort is useful in scenarios where you need to maintain a sorted dataset while continually adding new elements, as the binary heap can efficiently handle insertions and deletions while maintaining the sorted order.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is heap sort and how does it work?
Heap sort is a comparison-based sorting algorithm that works by dividing the input into a sorted and an unsorted region, then repeatedly extracting the maximum element from the unsorted region and placing it at the beginning of the sorted region. The main idea behind heap sort is to use a complete binary tree (heap) to organize the data while sorting. There are two types of heaps: max-heaps and min-heaps. Heap sort uses a max-heap to find the maximum element in the unsorted region, then swaps it with the last element of the unsorted region, effectively moving it to the sorted region.
What are the advantages of using heap sort compared to other sorting algorithms?
Heap sort has several advantages, including:
- In-place sorting: Heap sort does not require additional memory for temporary storage, making it an in-place sorting algorithm.
- Time complexity: Heap sort has a worst-case and average time complexity of O(n log n), which is quite good compared to sorting algorithms like bubble sort or insertion sort.
- Adaptability: Heap sort can handle large datasets efficiently, and it can also be parallelized to improve performance.
What is the difference between max-heap and min-heap?
A max-heap is a complete binary tree where each parent node has a value greater than or equal to its children's values, while a min-heap is a complete binary tree where each parent node has a value less than or equal to its children's values. In the context of heap sort, a max-heap is used to find the maximum element in the unsorted region of the input data.
How do you create a max-heap from an array?
To create a max-heap from an array, you can use the following process:
- Start at the middle of the array (index
n/2 - 1
for an array of sizen
). - Compare the current element with its children, and swap it with the largest child if the current element is smaller.
- Repeat the process for the next parent node, moving towards the root.
- Continue the process until the entire array forms a valid max-heap.
Can heap sort be used for sorting strings or other non-numeric data types?
Yes, heap sort can be used for sorting any data type that supports comparison, including strings or custom objects. The key is to define a comparison function that can determine the order of the elements in a consistent manner. For example, when sorting strings, you might compare their lexicographic order, and the heap sort algorithm would work just as well as with numeric data.