Understanding Merge Sort and Its Use Cases
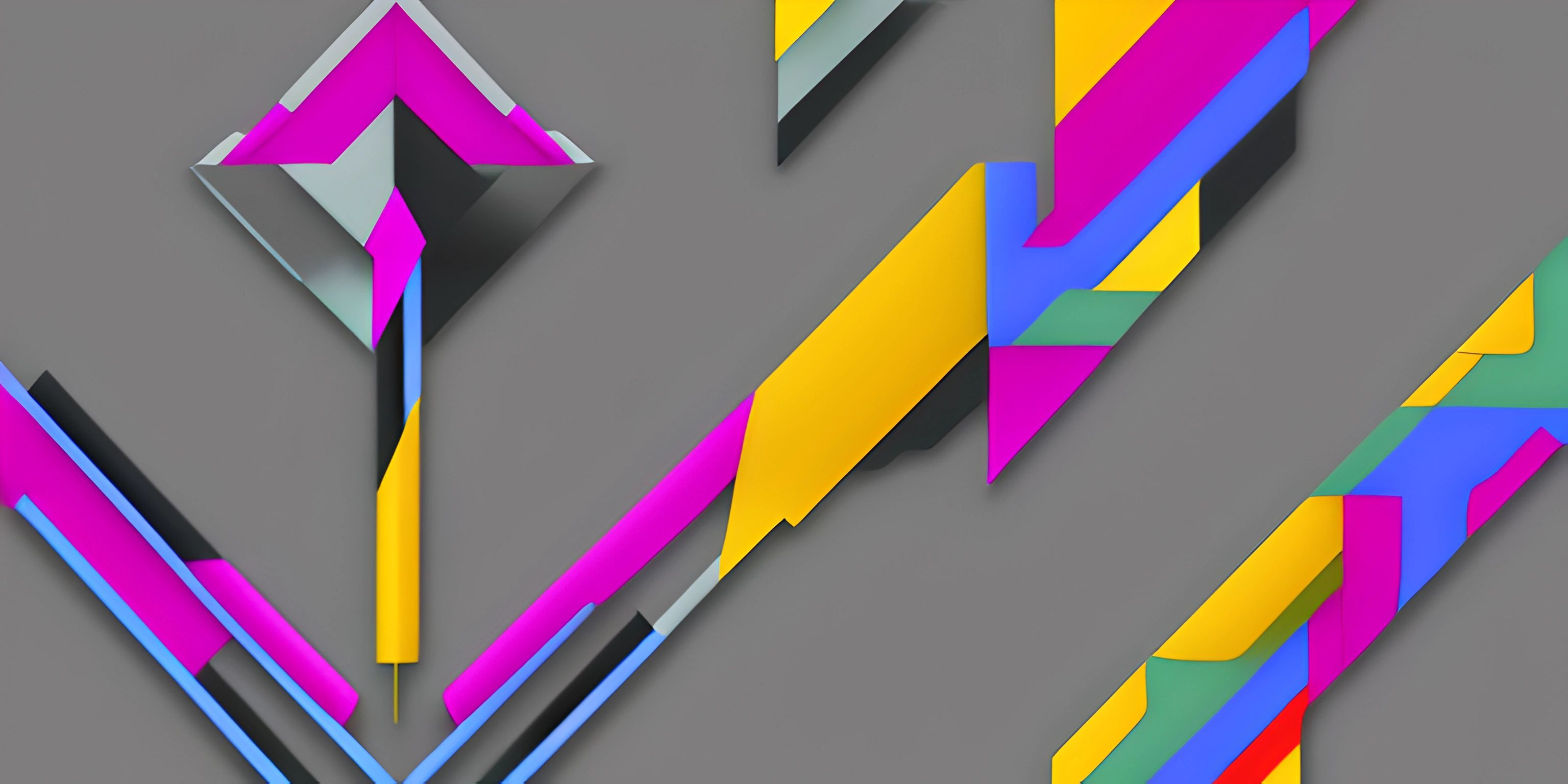
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to sorting data, there are many algorithms to choose from. One such famous algorithm is merge sort. Known for its efficiency and simplicity, merge sort is a powerful algorithm that can come to the rescue when you need to sort large amounts of data. Let's dive into the world of merge sort and understand how it ticks!
Divide and Conquer
Merge sort uses the divide and conquer approach, which means it breaks the problem down into smaller sub-problems until they are simple enough to solve. In the case of merge sort, the smaller sub-problems are arrays of length 1 or 2 which are easily sorted. Once sorted, the sub-arrays are merged back together in a sorted manner.
The Merge Sort Process
Let's walk through an example to see how merge sort works its magic. We'll use the following array of numbers:
[8, 3, 5, 1, 7, 9, 2, 4]
- First, we divide the array into two halves:
[8, 3, 5, 1] [7, 9, 2, 4]
- We continue dividing each half until we have arrays of length 1 or 2:
[8, 3] [5, 1] [7, 9] [2, 4]
- Now that we have simple sub-problems, we sort each sub-array:
[3, 8] [1, 5] [7, 9] [2, 4]
- Finally, we merge the sorted sub-arrays back together:
// Merge [3, 8] and [1, 5] [1, 3, 5, 8] // Merge [7, 9] and [2, 4] [2, 4, 7, 9] // Merge [1, 3, 5, 8] and [2, 4, 7, 9] [1, 2, 3, 4, 5, 7, 8, 9]
And voila! Our original array is now sorted.
Time Complexity and Use Cases
Merge sort has a time complexity of O(n log n), which makes it an efficient choice for sorting large datasets. However, it comes with a trade-off: it requires additional memory for the temporary arrays during the merge step. This extra memory usage might make merge sort less suitable for situations with limited memory.
Some use cases where merge sort shines include:
- Sorting large datasets
- Sorting data that is partially sorted
- Sorting linked lists, as merge sort can be implemented without extra memory
Conclusion
Merge sort is a powerful and efficient sorting algorithm that can handle large amounts of data. By understanding how it works and when to use it, you can make informed decisions when deciding which sorting algorithm is best for your specific needs. Happy sorting!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).