Heap Sort: An In-Depth Analysis
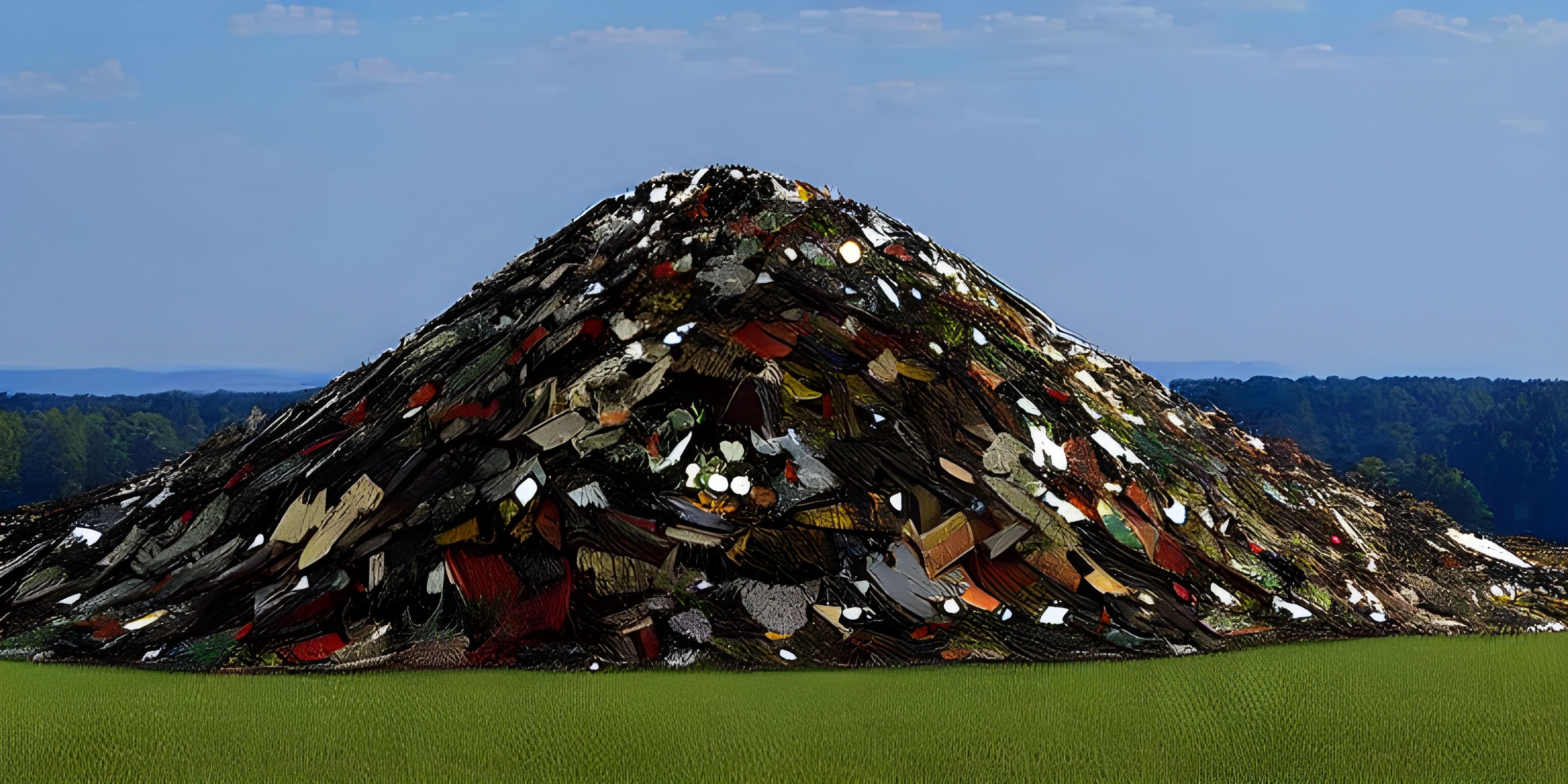
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Sorting algorithms are like different flavors of ice cream—each one has its unique taste and best-use scenario. Today, we’re going to indulge in the rich, complex flavor of heap sort. If you’ve never heard of it before, don’t worry—by the end of this article, you'll be a heap sort connoisseur.
Heap sort is a comparison-based sorting technique that utilizes a binary heap data structure. The algorithm can be broken down into a few straightforward steps, but it's the underlying mechanics that make it truly fascinating.
What is a Heap?
Before we jump into the algorithm, we need to understand what a heap is. A heap is a special tree-based data structure that satisfies the heap property. For a max heap, the value of each node is greater than or equal to the values of its children, and for a min heap, the value of each node is less than or equal to the values of its children.
Here’s a visual of a max heap:
50 / \ 30 20 / \ / \ 15 10 8 16
In a max heap, the largest element is always at the root. Conversely, in a min heap, the smallest element is at the root.
Building a Heap
Heap sort involves two main phases: building a heap and then sorting the elements. Let's start with building a heap from an unsorted array.
- Insert Elements: Insert elements into the heap one by one, ensuring that the heap property is maintained after each insertion.
- Heapify: Starting from the last non-leaf node, adjust the heap so that it satisfies the heap property.
Here's a Python implementation for building a max heap:
def heapify(arr, n, i): # Assume largest as root largest = i left = 2 * i + 1 right = 2 * i + 2 # Check if left child exists and is greater than root if left < n and arr[i] < arr[left]: largest = left # Check if right child exists and is greater than largest so far if right < n and arr[largest] < arr[right]: largest = right # Change root if needed if largest != i: arr[i], arr[largest] = arr[largest], arr[i] # Swap # Heapify the root heapify(arr, n, largest) def build_heap(arr): n = len(arr) # Start from the last non-leaf node and heapify each node for i in range(n // 2 - 1, -1, -1): heapify(arr, n, i)
Sorting the Heap
Once we have a max heap, the largest element is at the root. We swap it with the last element of the heap and reduce the heap size by one. Then, we heapify the root again to maintain the heap property. We repeat this process until the heap size is 1.
Here's the code to sort the heap:
def heap_sort(arr): n = len(arr) # Build a max heap build_heap(arr) # One by one extract elements for i in range(n - 1, 0, -1): arr[i], arr[0] = arr[0], arr[i] # Swap heapify(arr, i, 0) # Example usage arr = [12, 11, 13, 5, 6, 7] heap_sort(arr) print("Sorted array is", arr)
Advantages of Heap Sort
Heap sort has several advantages that make it a useful algorithm in various scenarios:
- Time Complexity: The time complexity of heap sort is O(n log n) for all cases—best, average, and worst. This is better than algorithms like bubble sort or insertion sort, which have a worst-case time complexity of O(n^2).
- Space Complexity: Heap sort is an in-place sorting algorithm, meaning it requires only a constant amount O(1) of additional memory space.
- No Quadratic Worst-Case: Unlike quicksort, heap sort doesn't suffer from a quadratic worst-case time complexity, making it more reliable for large datasets.
However, it's worth noting that heap sort is not a stable sort, meaning that the relative order of equal elements may not be preserved.
When to Use Heap Sort
Heap sort is particularly useful when you need a guarantee of O(n log n) time complexity and cannot afford the worst-case performance of quicksort. It’s also handy when working with limited memory, as it doesn’t require additional space like merge sort.
Heap sort is often used in priority queues and graph algorithms like Dijkstra's shortest path algorithm, where efficient retrieval of the maximum or minimum element is crucial.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).
FAQ
What is the difference between a max heap and a min heap?
In a max heap, the value of each node is greater than or equal to the values of its children, making the largest element the root. In a min heap, the value of each node is less than or equal to the values of its children, making the smallest element the root.
Is heap sort a stable sorting algorithm?
No, heap sort is not a stable sorting algorithm. The relative order of equal elements may not be maintained.
Can heap sort be used for large datasets?
Yes, heap sort is suitable for large datasets due to its O(n log n) time complexity and O(1) space complexity.
How does heap sort compare to quicksort?
Heap sort has a guaranteed O(n log n) time complexity in all cases, whereas quicksort has a worst-case time complexity of O(n^2). However, quicksort is often faster in practice due to better cache performance and lower constant factors.
Why is heap sort not commonly used in practice?
Despite its advantages, heap sort is not commonly used because other algorithms like quicksort and merge sort tend to be faster in practice due to better cache performance and simpler implementations.