Bubble Sort
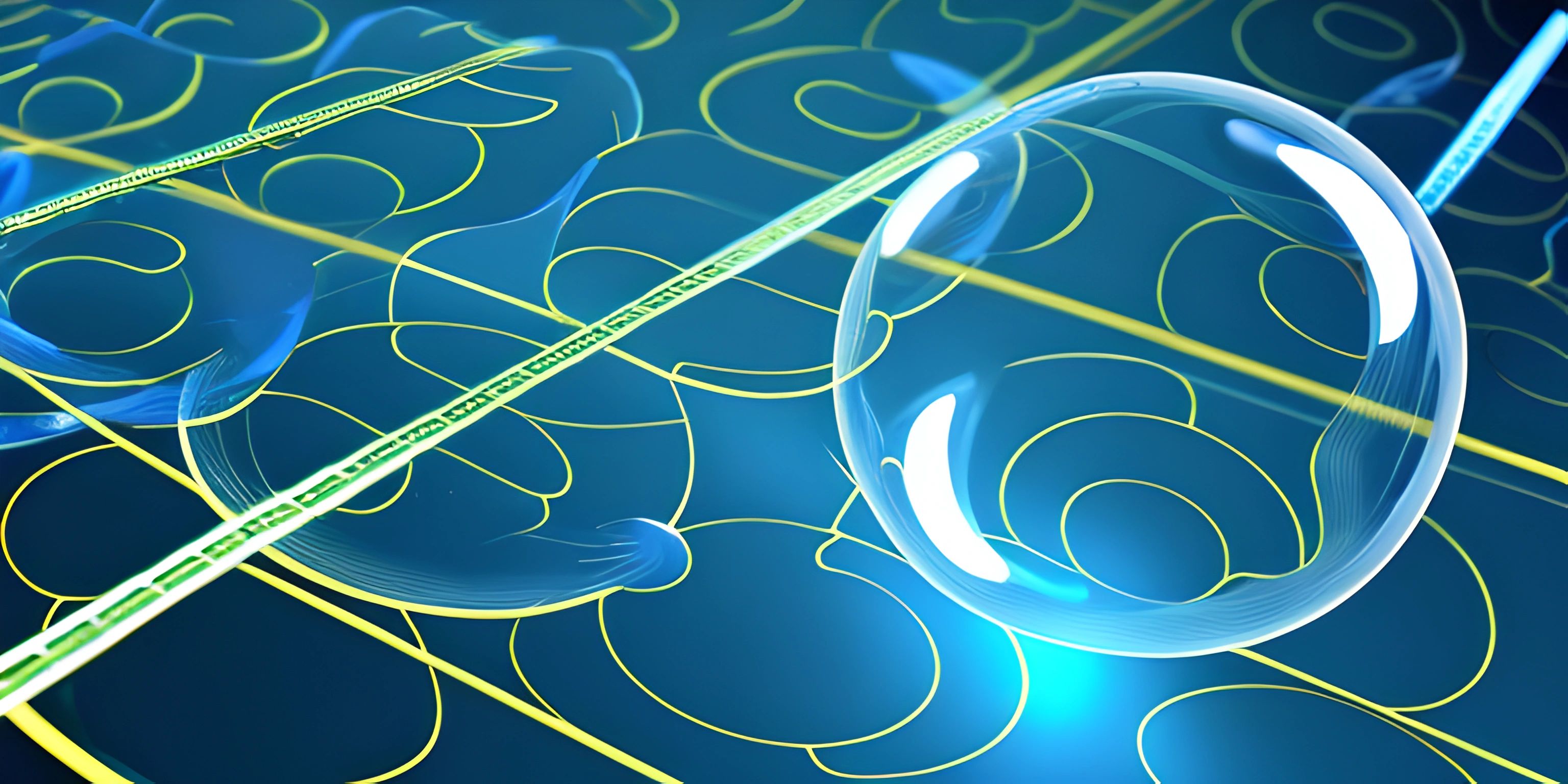
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Bubble Sort is a simple yet effective algorithm for sorting a list of elements. It's like organizing a line of dancers by height, where each time two dancers swap their positions, the entire line becomes more sorted. Bubble Sort involves repeatedly comparing and potentially swapping adjacent elements until the entire list is sorted.
How Bubble Sort Works
Imagine we have a list of numbers that we want to put in ascending order. The Bubble Sort algorithm compares each pair of adjacent numbers and swaps them if they are out of order. This process continues until the entire list is sorted. Here's a step-by-step breakdown:
- Start at the first element of the list.
- Compare it with the next element.
- If the first element is greater than the second, swap them.
- Move on to the next pair and repeat steps 2-3 until the end of the list is reached.
- If any swaps were made in the previous pass, go back to step 1.
The name "Bubble Sort" comes from the fact that smaller elements "bubble" to the beginning of the list, while larger elements "sink" to the end.
Implementation in Python
Here's a simple implementation of Bubble Sort in Python:
def bubble_sort(arr): n = len(arr) for i in range(n): # Initialize a flag to check if any swaps were made swapped = False # Iterate through the unsorted part of the list for j in range(0, n - i - 1): # Swap elements if they are out of order if arr[j] > arr[j + 1]: arr[j], arr[j + 1] = arr[j + 1], arr[j] swapped = True # If no swaps were made, the list is already sorted if not swapped: break return arr
Time Complexity
Bubble Sort has a worst-case and average time complexity of O(n^2), where n is the number of elements in the list. This makes it inefficient for large lists. However, it has a best-case time complexity of O(n) when the list is already sorted, which makes it advantageous in certain situations.
Applications
Bubble Sort is best suited for small datasets or lists that are already partially sorted. It's also a popular choice for educational purposes due to its simplicity and ease of understanding.
While you're unlikely to use Bubble Sort in production environments or for large datasets, it's still an essential algorithm to learn and understand as a programmer. It lays the foundation for more advanced sorting algorithms and helps you appreciate the importance of algorithmic efficiency.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the Bubble Sort algorithm?
Bubble Sort is a simple sorting algorithm that works by repeatedly stepping through the list, comparing each pair of adjacent items and swapping them if they are in the wrong order. The algorithm continues to do this until it makes a complete pass through the list without having to make any swaps, at which point the list is considered sorted.
How does the basic implementation of Bubble Sort look like in Python?
Here's a basic implementation of the Bubble Sort algorithm in Python:
def bubble_sort(arr): n = len(arr) for i in range(n): for j in range(0, n-i-1): if arr[j] > arr[j+1]: arr[j], arr[j+1] = arr[j+1], arr[j] example_list = [64, 34, 25, 12, 22, 11, 90] bubble_sort(example_list) print("Sorted list is:", example_list)
What is the time complexity of Bubble Sort?
The time complexity of Bubble Sort is O(n^2) in the worst and average cases, where 'n' is the number of items being sorted. This means that the algorithm's performance significantly degrades as the size of the input list increases. In the best case (when the list is already sorted), Bubble Sort has a time complexity of O(n).
Can Bubble Sort be optimized for better performance?
Yes, Bubble Sort can be optimized by adding a flag to check if any swaps occurred during an iteration. If no swaps occur, it means the list is already sorted, and we can break out of the loop early. Here's the optimized version in Python:
def optimized_bubble_sort(arr): n = len(arr) for i in range(n): swapped = False for j in range(0, n-i-1): if arr[j] > arr[j+1]: arr[j], arr[j+1] = arr[j+1], arr[j] swapped = True if not swapped: break
What are some common use cases for Bubble Sort?
Bubble Sort is generally not recommended for large datasets due to its poor performance (O(n^2) time complexity). However, it can be useful for educational purposes, as it's easy to understand and implement. It's also suitable for small datasets or partially sorted lists, where its simplicity and ease of coding might outweigh the benefits of more complex and efficient algorithms.