Algorithms and Data Structures
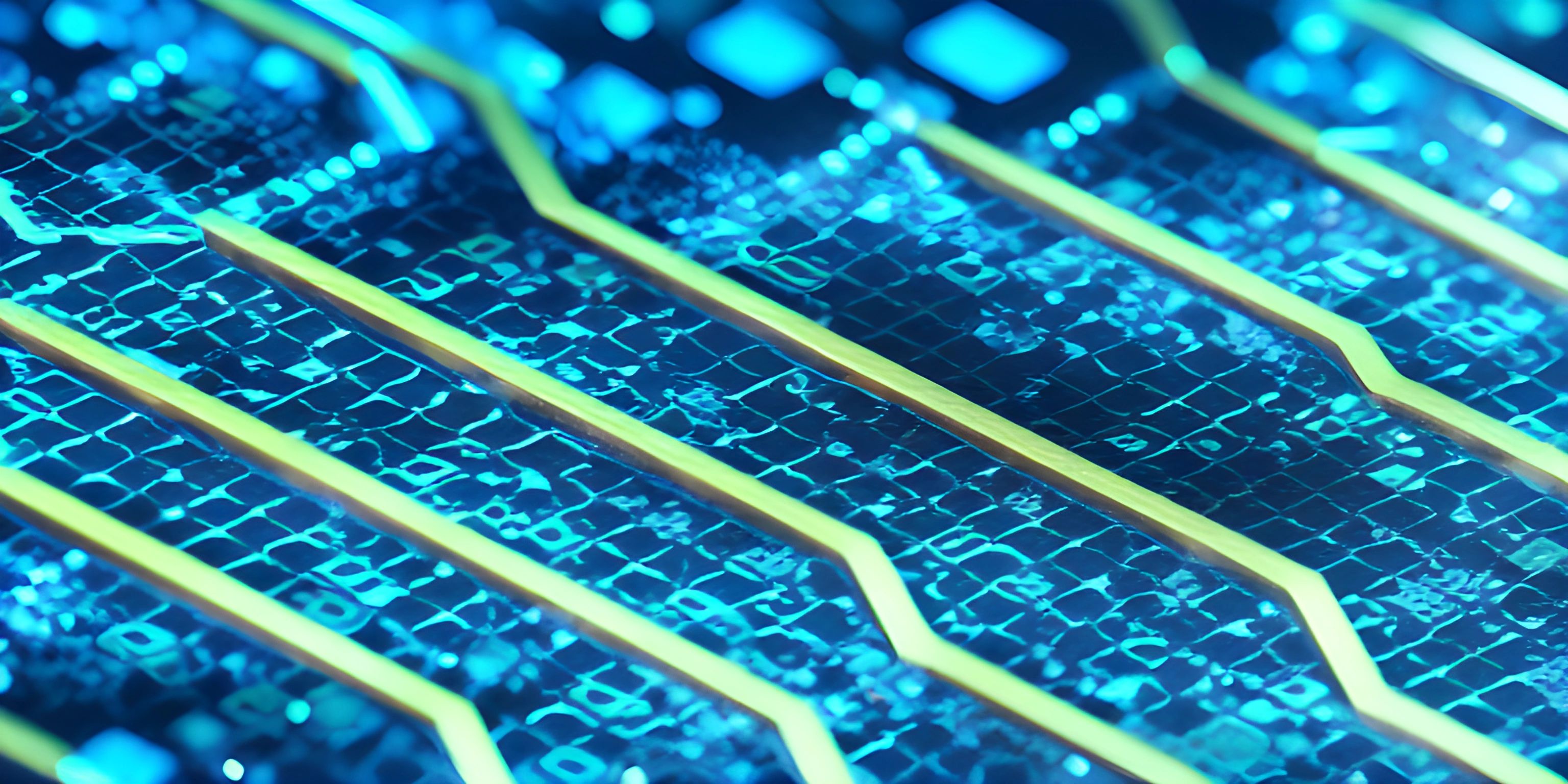
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Algorithms and data structures are the building blocks of efficient programs. They help us solve complex problems and optimize our code for better performance. To become a proficient programmer, it's essential to understand these key concepts and how to implement and utilize them effectively.
Algorithms
An algorithm is a step-by-step procedure to perform a specific task or to solve a particular problem. Some of the most well-known algorithms are:
-
Sorting algorithms: Arrange elements in a specific order. Examples include Bubble Sort, Quick Sort, and Merge Sort.
-
Search algorithms: Find the location of a specific element in a data structure. Examples include Linear Search and Binary Search.
-
Graph algorithms: Solve problems related to graphs, such as finding the shortest path between two nodes. Examples include Dijkstra's Algorithm and Depth-First Search.
Data Structures
A data structure is a way to organize and store data in a computer. Some common data structures are:
-
Arrays: A fixed-size collection of elements, stored in a contiguous block of memory. Arrays can be accessed using their index, making them very efficient for certain tasks.
-
Linked Lists: A collection of elements, where each element points to the next. Linked lists are useful when you need to insert or delete elements frequently.
-
Stacks: A collection of elements that follow the Last-In-First-Out (LIFO) principle. Elements are added and removed from the top of the stack.
-
Queues: A collection of elements that follow the First-In-First-Out (FIFO) principle. Elements are added at the rear and removed from the front.
-
Trees: A hierarchical structure with a root node and multiple children nodes. Examples include binary trees, AVL trees, and binary search trees.
Implementing and Using Algorithms and Data Structures
To effectively implement and use algorithms and data structures in your programs, follow these tips:
-
Understand the problem: Before diving into the implementation, make sure you clearly understand the problem you're trying to solve.
-
Choose the right tools: Select the most appropriate algorithm and data structure for the task. Consider factors such as time complexity, space complexity, and ease of implementation.
-
Test your code: Thoroughly test your implementation using various inputs to ensure correctness and efficiency.
-
Optimize: Analyze your code for potential improvements and make any necessary optimizations to enhance its performance.
-
Document your work: Write clear and concise comments to explain your code and make it easier to understand for others, and for yourself in the future.
By mastering the art of implementing and using algorithms and data structures, you'll be well on your way to becoming a skilled programmer.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Taking User Input (psst, it's free!).
FAQ
What are algorithms and data structures?
Algorithms are step-by-step procedures to perform a specific task or to solve a particular problem. Data structures are ways to organize and store data in a computer. Both are essential building blocks of efficient programs.
Can you give examples of some well-known algorithms?
Examples of well-known algorithms include Bubble Sort, Quick Sort, Merge Sort, Linear Search, Binary Search, Dijkstra's Algorithm, and Depth-First Search.
What are some common data structures?
Some common data structures include arrays, linked lists, stacks, queues, and trees.
How can I effectively implement and use algorithms and data structures in my programs?
To effectively implement and use algorithms and data structures, understand the problem, choose the right tools, test your code, optimize your implementation, and document your work.