Java Servlets Introduction
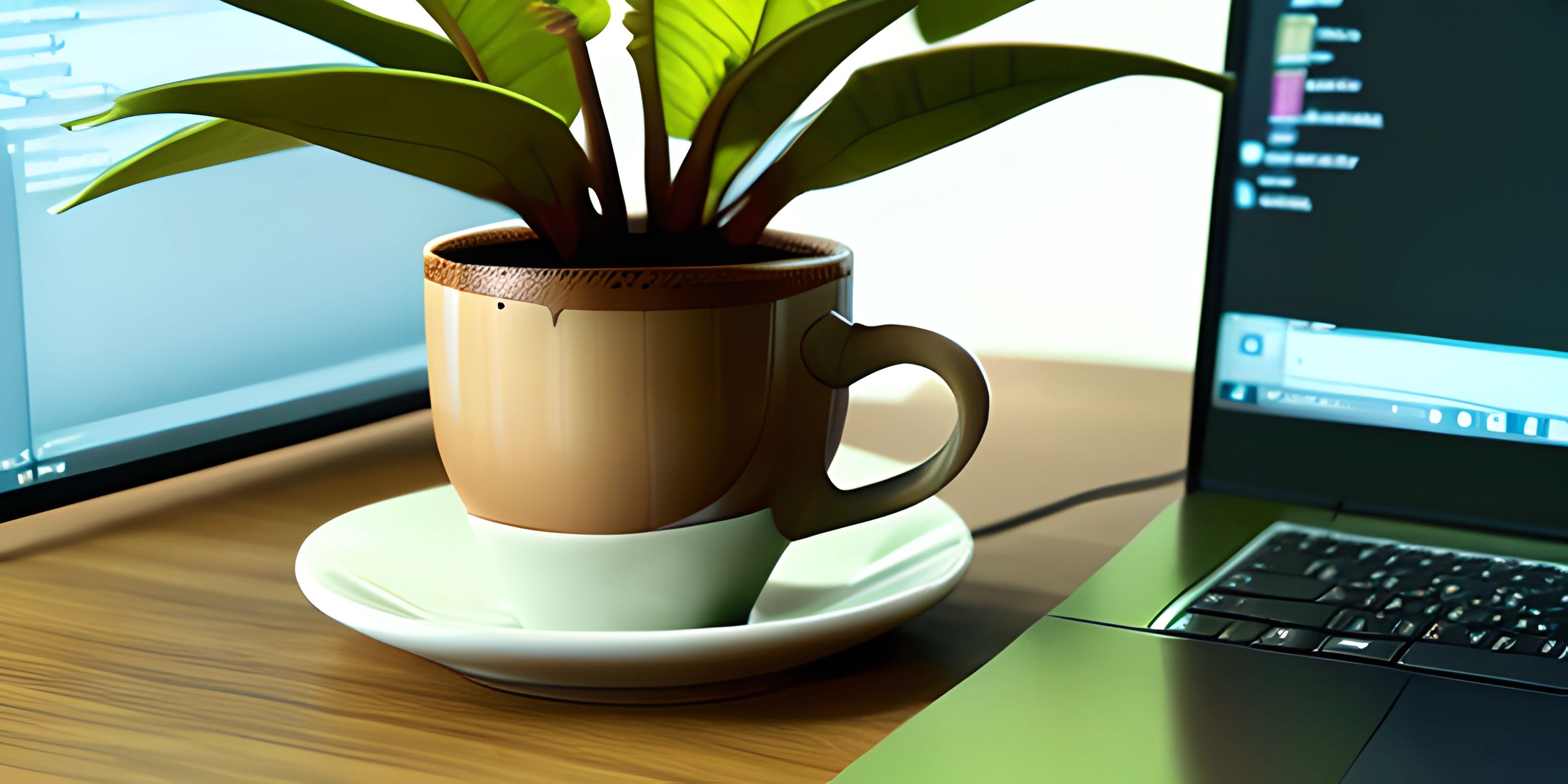
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the fascinating world of Java Servlets! These little web dynamos are an essential part of Java web development, and understanding them will unlock a world of possibilities for you. Let's jump right in!
What are Java Servlets?
Java Servlets are server-side components that extend the capabilities of web servers that host Java applications. They are used to handle HTTP requests, process data, and generate dynamic content for web pages. Servlets are like the backstage crew in a theatrical production, making sure everything runs smoothly and efficiently.
How Servlets Work
When a client (usually a web browser) sends an HTTP request to a web server, the server looks for a servlet that can handle the request. Once it finds the appropriate servlet, the server passes the request to the servlet, which processes the request and generates a response. The server then sends this response back to the client.
Creating a Java Servlet
Before you can create a Java Servlet, make sure you have the following installed on your computer:
- Java Development Kit (JDK)
- Apache Tomcat (a popular Java Servlet container)
- Integrated Development Environment (IDE), such as Eclipse or IntelliJ IDEA
Once you have these tools in place, follow these steps to create a simple Java Servlet:
-
Create a new Java web project in your IDE. Make sure to select "Dynamic Web Project" or a similar option when creating the project.
-
Add a new Java class to the project. Name the class something like
MyFirstServlet
and make sure it extendsjavax.servlet.http.HttpServlet
. -
Override the
doGet()
method. This method is automatically called by the server when an HTTP GET request is received. Inside this method, you can write the code to process the request and generate the response. Here's a basic example:
import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/MyFirstServlet") public class MyFirstServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); response.getWriter().println("<h1>Hello, World!</h1>"); } }
- Configure the web application. Open the
web.xml
file in your project'sWEB-INF
folder and add the following XML code to define the servlet and its URL mapping:
<servlet> <servlet-name>MyFirstServlet</servlet-name> <servlet-class>com.example.MyFirstServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>MyFirstServlet</servlet-name> <url-pattern>/MyFirstServlet</url-pattern> </servlet-mapping>
- Run the application. Deploy the web application to your Apache Tomcat server and access it using a web browser. You should see the "Hello, World!" message displayed on the page.
Congratulations! You've just created your very first Java Servlet!
Next Steps
Now that you have a basic understanding of Java Servlets and how to create them, it's time to dive deeper into more advanced topics, such as JavaServer Pages (JSP) and Java Servlet Filters. Keep learning and experimenting, and you'll soon become a master of Java web development!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Cratecode Playground (psst, it's free!).
FAQ
What are Java Servlets?
Java Servlets are server-side components that extend the capabilities of web servers that host Java applications. They handle HTTP requests, process data, and generate dynamic content for web pages.
How do Java Servlets work?
When a client sends an HTTP request to a web server, the server looks for a servlet that can handle the request. The server passes the request to the servlet, which processes the request and generates a response. The server then sends this response back to the client.
What are the prerequisites for creating a Java Servlet?
Before creating a Java Servlet, you need to have the Java Development Kit (JDK), Apache Tomcat (a popular Java Servlet container), and an Integrated Development Environment (IDE) such as Eclipse or IntelliJ IDEA installed on your computer.
How do I create a basic Java Servlet?
To create a basic Java Servlet, create a new Java web project in your IDE, add a new Java class that extends HttpServlet
, override the doGet()
method with your custom code, configure the web application using web.xml
, and run the application on your Apache Tomcat server.
What are some advanced topics to explore after learning about Java Servlets?
After getting started with Java Servlets, you can explore more advanced topics such as JavaServer Pages (JSP) and Java Servlet Filters to enhance your web development skills further.