Java Web Development
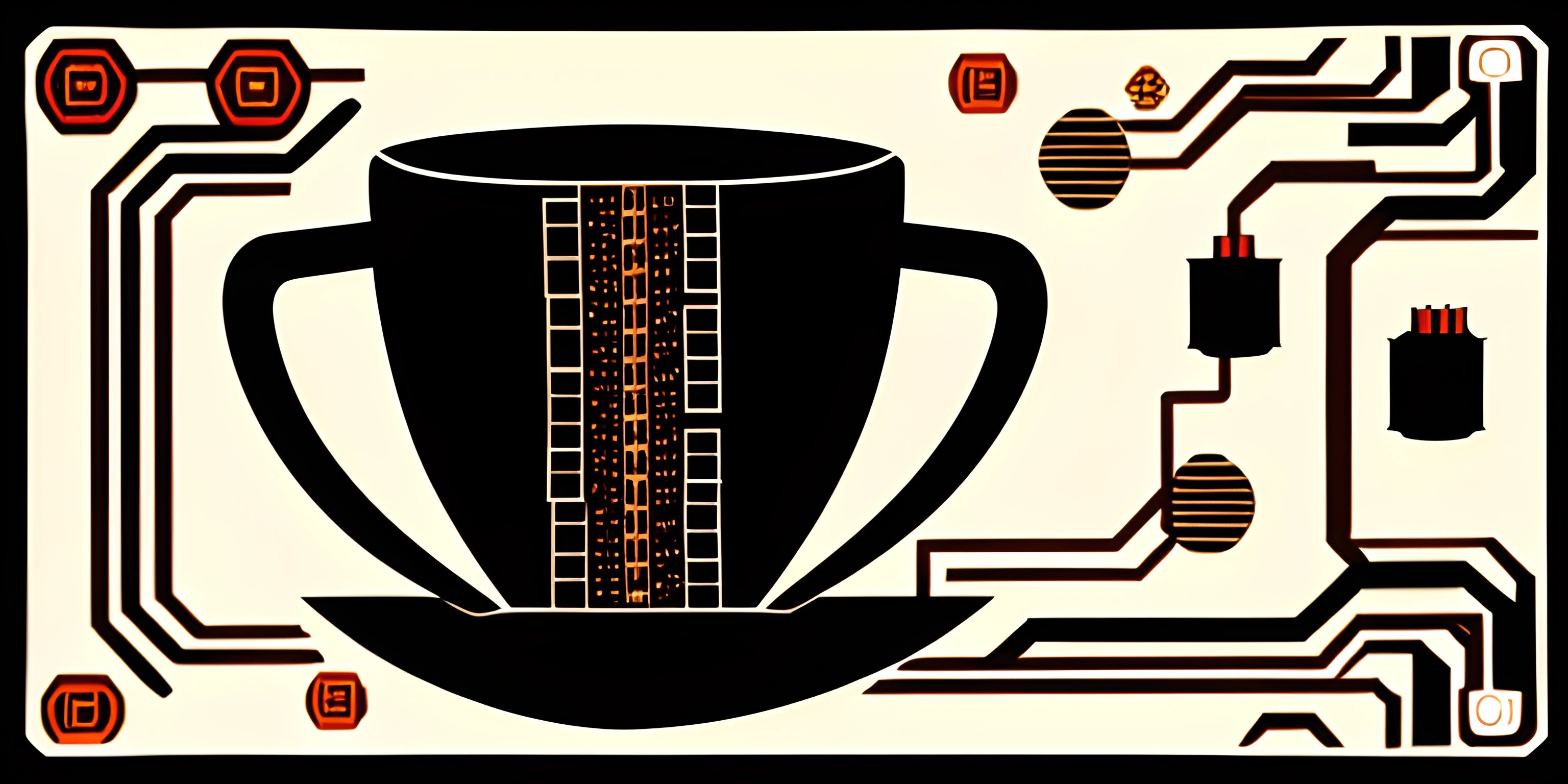
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Diving headfirst into web development with Java is like learning to ride a bike – a little wobbly at first, but with practice, it becomes second nature. In this guide, we'll walk through the process of building a web application using Java and its associated technologies.
Java Web Development Technologies
Before we hit the road, let's discuss the main technologies used in Java web development:
-
Java EE (Enterprise Edition): A powerful platform for building large-scale, distributed, and multi-tiered applications. Java EE provides a set of specifications and APIs that simplify the development of web applications.
-
Servlet: A Java class that extends the capabilities of servers hosting web applications. Servlets handle client requests, process them, and return a response, usually in the form of an HTML page.
-
JavaServer Pages (JSP): A technology that allows embedding Java code directly into HTML files, enabling the creation of dynamic web pages with Java.
-
Spring Framework: A popular Java framework that simplifies web application development by providing a comprehensive infrastructure for building, deploying, and maintaining web applications.
-
Hibernate: A powerful Object-Relational Mapping (ORM) library for Java that streamlines the process of mapping Java objects to database tables and vice versa.
Setting up the Environment
To kick off Java web development, we'll need the following tools:
- JDK (Java Development Kit): A development environment for building Java applications.
- Java IDE (Integrated Development Environment): A software application that provides a comfortable environment for Java developers, such as Eclipse or IntelliJ IDEA.
- Web server: A software that serves web pages, such as Apache Tomcat.
Once these tools are installed and configured, we can start building our web application.
Create a Servlet
Servlets are the building blocks of Java web applications. They act as a bridge between the user interface (HTML, CSS, JavaScript) and the server-side logic (Java code, databases).
Here's a simple example of a servlet:
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; public class HelloWorldServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { PrintWriter out = response.getWriter(); out.println("Hello, World!"); } }
This servlet responds to an HTTP GET request with the message "Hello, World!". To make this servlet accessible, we'll need to register it in the web.xml
file:
<servlet> <servlet-name>HelloWorldServlet</servlet-name> <servlet-class>HelloWorldServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>HelloWorldServlet</servlet-name> <url-pattern>/hello</url-pattern> </servlet-mapping>
Now, when we access the URL http://localhost:8080/your-app-name/hello
, the servlet will be executed and display "Hello, World!".
Integrate with JSP
JavaServer Pages (JSP) enable us to create dynamic web pages by mixing HTML and Java code. Here's a simple JSP example:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <title>Java Web Development</title> </head> <body> <h1>Welcome to Java Web Development!</h1> <% String name = "John Doe"; %> <p>Your name is <%= name %>.</p> </body> </html>
In this example, we declare a Java variable name
and display its value in the HTML content using <%= name %>
. JSP files are typically placed in the WebContent
folder of a Java web application.
Incorporate Spring and Hibernate
The Spring Framework and Hibernate provide powerful tools for developing complex web applications. To integrate them, we'll first need to add their dependencies to our project. In a Maven project, this is done by updating the pom.xml
file:
<dependencies> <!-- Spring Framework --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.3.10</version> </dependency> <!-- Hibernate --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>5.6.1.Final</version> </dependency> </dependencies>
Next, we can create a Spring configuration class to set up Spring MVC and Hibernate:
@Configuration @EnableWebMvc @ComponentScan(basePackages = "com.example") public class AppConfig { // ... }
With Spring and Hibernate integrated, we can now leverage their powerful features to create advanced web applications, such as accessing databases, managing transactions, and incorporating security features.
Conclusion
Java web development may seem daunting at first, but with the right tools and technologies, it becomes a breeze. By learning Servlets, JSP, Spring, and Hibernate, you'll be well on your way to building impressive web applications using Java. So gear up, and let the Java web development adventure begin!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What is Backend? (psst, it's free!).
FAQ
What technologies are commonly used in Java web development?
Java web development relies on a variety of technologies to create robust and efficient web applications. Some of the commonly used technologies include:
- Servlets: Java classes that handle web requests and generate dynamic responses.
- JavaServer Pages (JSP): A technology that allows embedding Java code directly into HTML pages for server-side scripting.
- JavaServer Faces (JSF): A component-based web application framework for building user interfaces.
- Spring Framework: A popular framework that simplifies various aspects of Java web development, such as dependency injection, data access, and security.
- Hibernate: An object-relational mapping (ORM) library for mapping Java objects to database tables.
How do I create a basic Java web application using Servlets?
You can create a basic Java web application using Servlets by following these steps:
- Install a Java Development Kit (JDK) and set up your development environment.
- Create a new Java project in your preferred IDE.
- Add the necessary dependencies, such as the Java Servlet API.
- Create a Java class that extends HttpServlet and overrides the doGet() or doPost() methods.
- In the overridden method, write your application logic and generate the HTML response using response.getWriter().
- Configure the web.xml file to map the Servlet to a specific URL pattern.
- Deploy your application to a web server, such as Apache Tomcat. Here's a simple example of a Servlet:
import javax.servlet.*; import javax.servlet.http.*; import java.io.*; public class HelloWorldServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { PrintWriter out = response.getWriter(); out.println("<html><head><title>Hello World</title></head>"); out.println("<body><h1>Hello World!</h1></body></html>"); } }
What are the advantages of using Java for web development?
Java offers several advantages for web development, including:
- Platform independence: Java applications can run on any platform with a Java Virtual Machine (JVM), making them highly portable.
- Robustness: Java's strong typing and exception handling mechanisms help to create reliable and bug-free applications.
- Scalability: Java's multi-threading capabilities and robust libraries make it suitable for building large-scale, high-performance web applications.
- Rich ecosystem: Java has a vast ecosystem of libraries, frameworks, and tools that simplify various aspects of web development.
- Strong community support: Java is a popular programming language with a large and active community, providing resources, tutorials, and help forums.
Can I use Java with popular front-end frameworks like React and Angular?
Yes, you can use Java alongside popular front-end frameworks like React and Angular to create powerful web applications. Java can be used for server-side operations, such as handling API requests, data processing, and database interactions, while React or Angular can be used for building responsive and interactive user interfaces. This approach allows you to leverage the strengths of both Java and the chosen front-end framework to create a feature-rich and efficient web application.