Understanding Hibernate and Its Role in Java Persistence
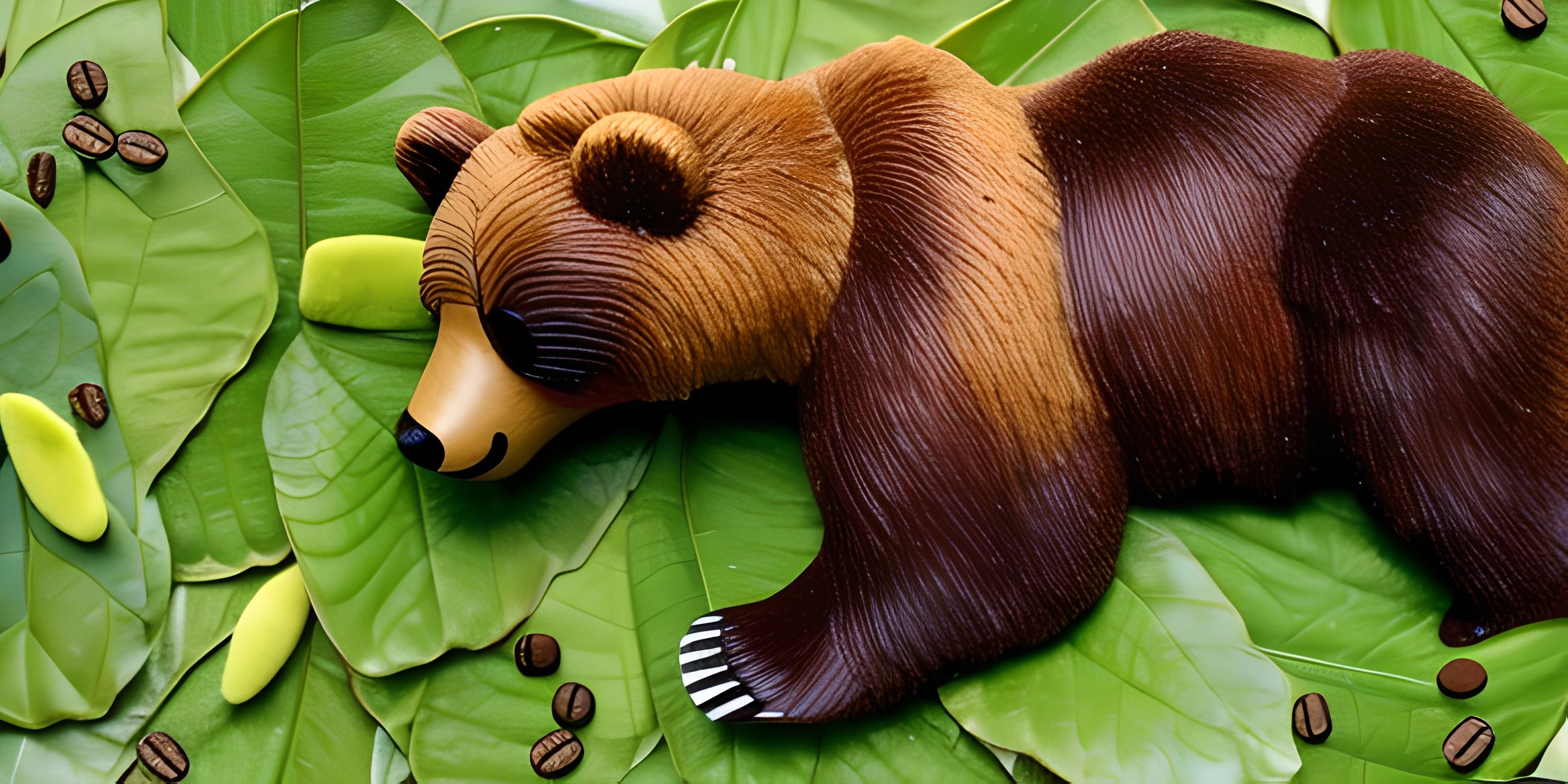
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you have ever worked with Java and databases, you’ve likely heard about Hibernate. Hibernate is an open-source, robust, and high-performance persistence framework for Java. It simplifies the task of mapping Java Object Relational Mapping (ORM) to relational databases, making it easier to manage and access data. Let's dive into the world of Hibernate and understand its role in Java persistence.
Java Persistence
Java persistence refers to the process of storing, retrieving, and managing data from a relational database using Java objects. This is achieved by mapping Java objects to database tables and converting the object's attributes to table columns. The ORM (Object Relational Mapping) technique is used for this purpose.
Before Hibernate came into the picture, developers had to write a lot of boilerplate code to handle database operations. But with Hibernate, developers can now focus on the actual business logic and let the framework handle the underlying database operations. Hibernate not only simplifies the development process but also improves the performance and maintainability of applications.
Hibernate Overview
Hibernate is an ORM framework that implements the Java Persistence API (JPA) specifications. It provides a powerful and flexible programming model for working with data in Java applications. Some of the key features of Hibernate include:
-
Transparent Persistence: Hibernate takes care of the underlying database operations, allowing developers to focus on writing clean and maintainable code.
-
Efficient Data Retrieval: Hibernate supports advanced querying capabilities, such as HQL (Hibernate Query Language), Criteria Query, and Native SQL Query, which provide efficient ways to retrieve data from the database.
-
Caching: Hibernate provides a powerful caching mechanism that helps reduce the number of database calls, thereby improving the performance of applications.
-
Lazy Loading: Hibernate uses lazy loading techniques to minimize the amount of data fetched from the database, which helps optimize application performance.
-
Concurrency Control: Hibernate offers various concurrency control mechanisms, including optimistic and pessimistic locking, to ensure data consistency in multi-user environments.
Getting Started with Hibernate
To get started with Hibernate, you'll need to follow these steps:
- Add Hibernate Dependencies: Include the required Hibernate libraries in your project using a build tool like Maven or Gradle.
<!-- Maven --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>5.6.5.Final</version> </dependency>
- Configure Hibernate: Create a
hibernate.cfg.xml
configuration file to specify the database settings, such as the JDBC driver, database URL, username, and password.
<hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="hibernate.connection.driver_class">org.h2.Driver</property> <property name="hibernate.connection.url">jdbc:h2:mem:testdb</property> <property name="hibernate.connection.username">sa</property> <property name="hibernate.connection.password"></property> <!-- Hibernate properties --> <property name="hibernate.dialect">org.hibernate.dialect.H2Dialect</property> <property name="hibernate.show_sql">true</property> <!-- Mapping of Java classes to database tables --> <mapping class="com.example.Employee"/> </session-factory> </hibernate-configuration>
- Create Java Entities: Define Java classes that represent the database tables, and use JPA annotations to map the class attributes to table columns.
@Entity public class Employee { @Id @GeneratedValue private Long id; private String name; private String email; // Getters and setters }
- Perform CRUD Operations: Use the
Session
andTransaction
objects provided by Hibernate to perform Create, Read, Update, and Delete (CRUD) operations on the Java entities.
// Create a new employee Session session = HibernateUtil.getSessionFactory().openSession(); Transaction transaction = session.beginTransaction(); Employee employee = new Employee("John Doe", "[email protected]"); session.save(employee); transaction.commit(); session.close();
Conclusion
Hibernate has undoubtedly revolutionized the way Java developers interact with relational databases. It provides a powerful, flexible, and performance-optimized framework for implementing Java persistence. By simplifying the complexities of database operations, Hibernate allows developers to focus on writing clean, maintainable, and efficient code. So, go ahead and give Hibernate a try in your next Java project, and experience the difference for yourself.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Hibernate in Java, and why is it important?
Hibernate is an open-source Java framework that simplifies the implementation of Java persistence, allowing you to store and retrieve Java objects in a relational database. It provides a powerful Object-Relational Mapping (ORM) solution that streamlines the process of interacting with databases, reducing the need for repetitive Data Access Object (DAO) code. By utilizing Hibernate, developers can optimize performance and maintainability, while effectively dealing with complex data relationships.
How does Hibernate's Object-Relational Mapping (ORM) work?
Hibernate's ORM functionality allows you to map Java objects to database tables and vice versa, using XML or annotation-based configuration. This mapping creates a virtual object-database table association, enabling seamless data manipulation through simple Java code. Hibernate handles the conversion of Java objects into database-specific SQL statements, abstracting the underlying database complexity and allowing developers to focus on the application logic.
@Entity @Table(name = "users") public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "username") private String username; @Column(name = "email") private String email; // Getters and Setters }
What are the main components of Hibernate?
The main components of Hibernate include:
- SessionFactory: A thread-safe, singleton object that is created once per application and used to obtain Session instances.
- Session: Represents a single unit of work and is used to interact with the database. Each session provides methods for CRUD operations, queries, and transactions.
- Transaction: Represents a single atomic unit of work that can be committed or rolled back. It provides methods for managing database transactions, ensuring data consistency and integrity.
- Query: Allows you to perform database queries using Hibernate Query Language (HQL), Criteria API or SQL. It provides a consistent, object-oriented way to query the database, regardless of the underlying SQL dialect.
Can I use Hibernate with different databases?
Yes, Hibernate is highly adaptable and supports a wide range of databases, including MySQL, PostgreSQL, Oracle, SQL Server, and more. It achieves this database-agnostic nature through its dialect configuration, which translates Hibernate's generic SQL generation into database-specific SQL statements. By simply updating the dialect in the Hibernate configuration, you can switch between different databases without modifying your Java code.